先决条件:MongoDB:简介
MongoDB是一个跨平台的, 面向文档的数据库, 可处理集合和文档的概念。 MongoDB提供高速, 高可用性和高可伸缩性。
在人们心中出现的下一个问题是"为什么要使用MongoDB"?
选择MongoDB的原因:
- 它支持分层数据结构(请参阅docs有关详细信息)
- 它支持关联数组, 例如Python中的Dictionary。
- 内置Python驱动程序, 可将python应用程序与数据库连接。示例-PyMongo
- 它是为大数据而设计的。
- MongoDB的部署非常容易。
MongoDB与RDBMS
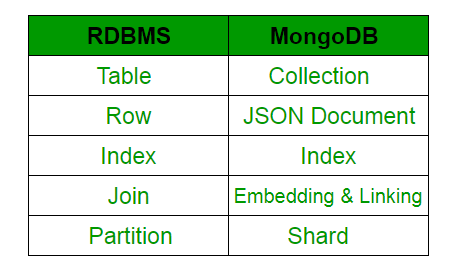
MongoDB和PyMongo安装指南
首先使用以下命令从命令提示符启动MongoDB:
方法1:
mongod
or
方法2:
net start MongoDB
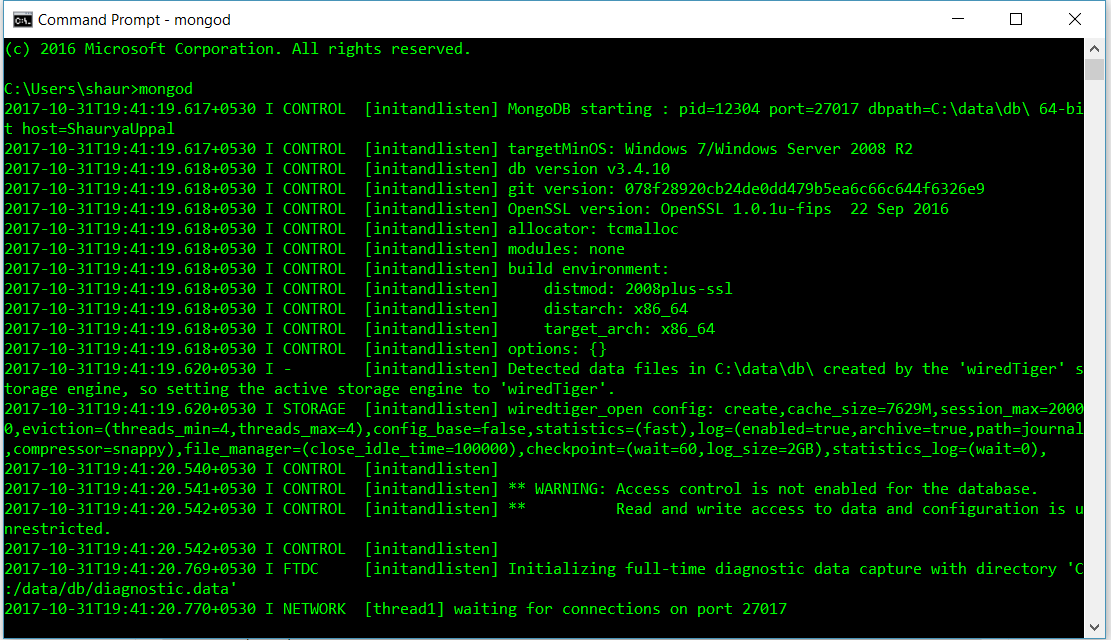
请参阅默认情况下将端口号设置为27017(上图中的最后一行)。
Python具有适用于MongoDB的本机库。可用库的名称为" PyMongo"。要导入此文件, 请执行以下命令:
from pymongo import MongoClient
创建一个连接:
导入模块后的第一个步骤是创建MongoClient。
from pymongo import MongoClient
client = MongoClient()
之后, 连接到默认主机和端口。到主机和端口的连接已明确完成。以下命令用于在端口27017上运行的本地主机上连接MongoClient。
client = MongoClient(‘host’, port_number)
example: - client = MongoClient(‘localhost’, 27017 )
也可以使用以下命令完成此操作:
client = MongoClient("mongodb: //localhost: 27017 /")
访问数据库对象:
要创建数据库或切换到现有数据库, 我们使用:
方法1:词典样式
mydatabase = client[‘name_of_the_database’]
方法2:
mydatabase = client.name_of_the_database
如果以前没有使用该名称创建的数据库, MongoDB将为用户隐式创建一个数据库。
注意:数据库填充的名称不能使用其中的任何破折号(-)。像my-Table这样的名称将引发错误。因此, 允许在名称中使用下划线。
访问集合:
集合等效于RDBMS中的表。我们在PyMongo中访问集合的方式与在RDBMS中访问表的方式相同。要访问该表, 请说数据库的表名" myTable", 说" mydatabase"。
方法1:
mycollection = mydatabase[‘myTable’]
方法2:
mycollection = mydatabase.myTable
> MongoDB以字典的形式存储数据库, 如下所示:
record = {
title: 'MongoDB and Python', description: 'MongoDB is no SQL database', tags: ['mongodb', 'database', 'NoSQL'], viewers: 104
}
" _id"是特殊键, 如果程序员忘记显式添加, 则会自动添加。 _id是12个字节的十六进制数字, 可确保每个插入的文档的唯一性。
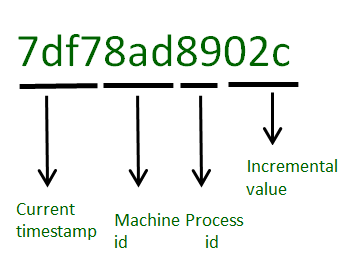
将数据插入集合中:
使用的方法:
insert_one() or insert_many()
我们通常在我们的集合中使用insert_one()方法文档。假设我们希望将名为记录的数据输入到" mydatabase"的" myTable"中。
rec = myTable.insert_one(record)
需要执行时, 整个代码看起来像这样。
# importing module
from pymongo import MongoClient
# creation of MongoClient
client = MongoClient()
# Connect with the portnumber and host
client = MongoClient("mongodb: //localhost: 27017 /")
# Access database
mydatabase = client[‘name_of_the_database’]
# Access collection of the database
mycollection = mydatabase[‘myTable’]
# dictionary to be added in the database
rec = {
title: 'MongoDB and Python' , description: 'MongoDB is no SQL database' , tags: [ 'mongodb' , 'database' , 'NoSQL' ], viewers: 104
}
# inserting the data in the database
rec = mydatabase.myTable.insert(record)
在MongoDB中查询:
有某些查询函数可用于归档数据库中的数据。两个最常用的功能是:
find()
find()用于获取多个单个文档作为查询结果。
for i in mydatabase.myTable.find({title: 'MongoDB and Python' })
print (i)
这将输出mydatabase的myTable中标题为" MongoDB and Python"的所有文档。
count()
count()用于获取通过参数传入名称的文档数量。
print (mydatabase.myTable.count({title: 'MongoDB and Python' }))
这将输出mydatabase的myTable中标题为" MongoDB and Python"的文档数。
可以对这两个查询函数求和, 以得出过滤效果最好的结果, 如下所示。
print (mydatabase.myTable.find({title: 'MongoDB and Python' }).count())
要打印数据库" mydatabase"的" myTable"内的所有文档/条目:
使用以下代码:
from pymongo import MongoClient
try :
conn = MongoClient()
print ( "Connected successfully!!!" )
except :
print ( "Could not connect to MongoDB" )
# database name: mydatabase
db = conn.mydatabase
# Created or Switched to collection names: myTable
collection = db.myTable
# To find() all the entries inside collection name 'myTable'
cursor = collection.find()
for record in cursor:
print (record)
本文作者:里沙卜·班萨尔(Rishabh Bansal)和莎莉娅·乌帕(Shaurya Uppal).
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。