包是一种结构化许多包和模块的方法, 有助于组织数据集的层次结构, 使目录和模块易于访问。就像操作系统中有不同的驱动器和文件夹来帮助我们存储文件一样, 类似的包也可以帮助我们存储其他子包和模块, 以便用户在必要时可以使用它。
创建和探索包
为了告诉Python特定目录是一个包, 我们在其中创建一个名为__init__.py的文件, 然后将其视为包, 并且可以在其中创建其他模块和子包。该__init__.py文件可以保留为空白, 或者可以使用程序包的初始化代码进行编码。
要使用Python创建一个包, 我们需要遵循以下三个简单步骤:
- 首先, 我们创建一个目录并给它一个程序包名称, 最好与它的操作有关。
- 然后, 我们将类和所需的函数放入其中。
- 最后, 我们在目录内创建一个__init__.py文件, 以使Python知道该目录是一个包。
创建包的示例
让我们看一下这个例子, 看看如何创建一个包。让我们创建一个名为Cars的程序包, 并在其中构建三个模块, 分别是宝马, 奥迪和日产。
首先, 我们创建一个目录并将其命名为Cars。
然后我们需要创建模块
。为此, 我们需要创建一个名称为Bmw.py的文件, 并通过将该代码放入其中来创建其内容。
# Python code to illustrate the Modules
class Bmw:
# First we create a constructor for this class
# and add members to it, here models
def __init__( self ):
self .models = [ 'i8' , 'x1' , 'x5' , 'x6' ]
# A normal print function
def outModels( self ):
print ( 'These are the available models for BMW' )
for model in self .models:
print ( '\t%s ' % model)
然后, 我们创建另一个名为Audi.py的文件, 并使用不同的成员向其中添加相似类型的代码。
# Python code to illustrate the Module
class Audi:
# First we create a constructor for this class
# and add members to it, here models
def __init__( self ):
self .models = [ 'q7' , 'a6' , 'a8' , 'a3' ]
# A normal print function
def outModels( self ):
print ( 'These are the available models for Audi' )
for model in self .models:
print ( '\t%s ' % model)
然后, 我们创建另一个名为Nissan.py的文件, 并使用不同的成员向其添加相似类型的代码。
# Python code to illustrate the Module
class Nissan:
# First we create a constructor for this class
# and add members to it, here models
def __init__( self ):
self .models = [ 'altima' , '370z' , 'cube' , 'rogue' ]
# A normal print function
def outModels( self ):
print ( 'These are the available models for Nissan' )
for model in self .models:
print ( '\t%s ' % model)
最后, 我们创建__init__.py文件。
该文件将放置在Cars目录中, 可以留空, 也可以将初始化代码放入其中。
from Bmw import Bmw
from Audi import Audi
from Nissan import Nissan
现在, 让我们使用我们创建的包。为此, 请在Cars软件包所在的目录中创建一个sample.py文件, 并在其中添加以下代码:
# Import classes from your brand new package
from Cars import Bmw
from Cars import Audi
from Cars import Nissan
# Create an object of Bmw class & call its method
ModBMW = Bmw()
ModBMW.outModels()
# Create an object of Audi class & call its method
ModAudi = Audi()
ModAudi.outModels()
# Create an object of Nissan class & call its method
ModNissan = Nissan()
ModNissan.outModels()
各种访问软件包的方式
让我们看一下这个示例, 并尝试将其与软件包相关联, 以及如何访问它。
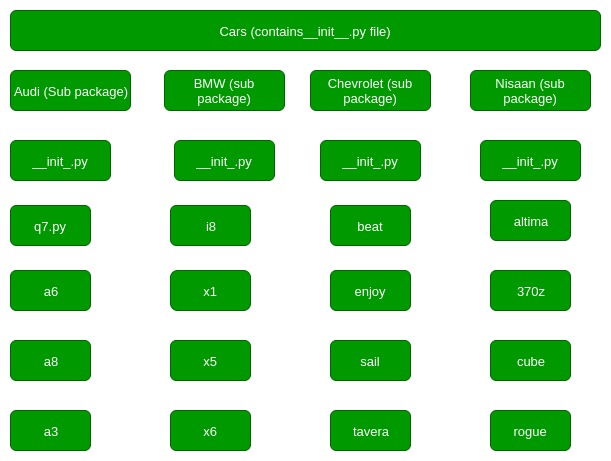
导入包
假设汽车和品牌目录是包裹。为了使它们成为一个包, 它们都必须在其中包含__init__.py文件, 该文件可以为空白, 也可以带有一些初始化代码。假设所有汽车模型都是模块。使用软件包有助于单个或全部导入任何模块。
假设我们要购买宝马i8。其语法为:
'import' Cars.Bmw.x5
导入包或子包或模块时, Python会搜索整个目录树以查找特定的包, 并按点运算符的编程进行系统地处理。
如果任何模块包含一个函数, 我们想将其导入。例如, a8具有函数get_buy(1), 而我们想导入该函数, 则语法为:
import Cars.Audi.a8
Cars.Audi.a8.get_buy(1)
仅使用导入语法时, 必须记住最后一个属性必须是子包或模块, 而不能是任何函数或类名。
包中的"from...import"
现在, 无论何时需要使用此类函数, 我们都需要在导入父包后写完整的长行。为了更简单地解决这个问题, 我们使用" from"关键字。为此, 我们首先需要使用'from'和'import'引入模块:
from Cars.Audi import a8
现在我们可以在任何地方使用
a8.get_buy(1)
还有另一种方法, 它不那么冗长。我们可以直接导入该函数, 并在必要时使用它。首先使用以下命令导入它:
from Cars.Audi.a8 import get_buy
现在从任何地方调用该函数:
get_buy(1)
包中的" from ... import *"
在使用
from import
语法, 我们可以将任何东西从子模块导入到在同一模块中定义的类或函数或变量。如果在包中未定义导入部分中提到的属性, 则编译器将引发ImportError异常。
导入子模块可能会导致在显式导入子模块时发生不必要的副作用。因此, 我们可以使用*语法一次导入各种模块。语法为:
from Cars.Chevrolet import *
这将从子包中导入所有内容, 即模块, 子模块, 功能, 类。
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。