本文概述
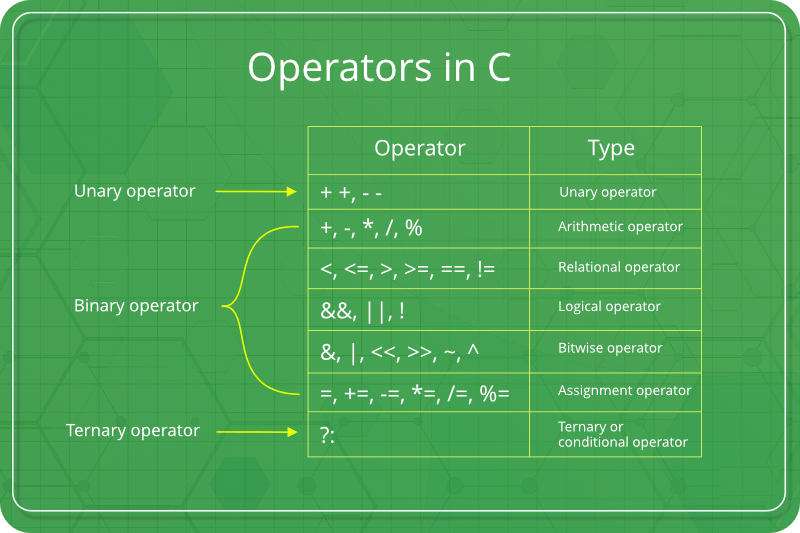
运算符是任何编程语言的基础。因此, 如果不使用运算符, C语言的功能是不完整的。运算符允许我们对操作数执行不同类型的运算。在C中, 可以将操作符归类为以下类别:
- 算术运算符s(+, -, *, /, %, 后递增, 前递增, 后递减, 前递减)
- 关系运算符(==, !=, >, <, > =&<=)逻辑运算符(&&, ||和!)
- 按位运算符(&, |, ^, ~, >>和<<)
- 赋值运算符s(=, + =, -=, * =等)
- 其他运营商(条件, 逗号, sizeof, 地址, 重定向)
算术运算符:这些用于对操作数执行算术/数学运算。属于此类的二进制运算符是:
- 加成:的‘+’运算符将两个操作数相加。例如, x + y.
- 减法:的‘-’运算符减去两个操作数。例如, 设.
- 乘法:的‘*’运算符将两个操作数相乘。例如, x * y.
- 师:的‘/’运算符将第一个操作数除以第二个。例如, x / y.
- 模量:的‘%’当第一个操作数除以第二个操作数时, 运算符将返回余数。例如, x%y.
C
//C program to demonstrate working of binary arithmetic operators
#include <stdio.h>
int main()
{
int a = 10, b = 4, res;
//printing a and b
printf ( "a is %d and b is %d\n" , a, b);
res = a + b; //addition
printf ( "a+b is %d\n" , res);
res = a - b; //subtraction
printf ( "a-b is %d\n" , res);
res = a * b; //multiplication
printf ( "a*b is %d\n" , res);
res = a /b; //division
printf ( "a/b is %d\n" , res);
res = a % b; //modulus
printf ( "a%b is %d\n" , res);
return 0;
}
C ++
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 4, res;
//printing a and b
cout<<"a is " <<a<<" and b is " <<b<<"\n" ;
//addition
res = a + b;
cout <<"a+b is: " <<res <<"\n" ;
//subtraction
res = a - b;
cout <<"a-b is: " <<res <<"\n" ;
//multiplication
res = a * b;
cout <<"a*b is: " <<res <<"\n" ;
//division
res = a /b;
cout <<"a/b is: " <<res <<"\n" ;
//modulus
res = a % b;
cout <<"a%b is: " <<res <<"\n" ;
return 0;
}
输出如下:
a is 10 and b is: 4
a+b is: 14
a-b is: 6
a*b is: 40
a/b is: 2
a%b is: 2
属于一元算术运算符类别的是:
- 增量:的‘++’运算符用于递增整数值。当放置在变量名称(也称为预增量运算符)之前时, 其值会立即增加。例如, ++ x.
当将其放在变量名之后(也称为后递增运算符)时, 其值将暂时保留, 直到执行该语句为止, 并且在下一个语句执行之前对其进行更新。例如, x ++. - 减量:的‘– –’运算符用于减少整数值。当放置在变量名称(也称为预减运算符)之前时, 其值将立即减小。例如, - - X.
当将其放在变量名(也称为后减运算符)之后时, 其值将暂时保留, 直到执行该语句为止, 并且在下一个语句执行之前对其进行更新。例如, X - -.
C
//C program to demonstrate working of Unary arithmetic operators
#include <stdio.h>
int main()
{
int a = 10, b = 4, res;
//post-increment example:
//res is assigned 10 only, a is not updated yet
res = a++;
printf ( "a is %d and res is %d\n" , a, res); //a becomes 11 now
//post-decrement example:
//res is assigned 11 only, a is not updated yet
res = a--;
printf ( "a is %d and res is %d\n" , a, res); //a becomes 10 now
//pre-increment example:
//res is assigned 11 now since a is updated here itself
res = ++a;
//a and res have same values = 11
printf ( "a is %d and res is %d\n" , a, res);
//pre-decrement example:
//res is assigned 10 only since a is updated here itself
res = --a;
//a and res have same values = 10
printf ( "a is %d and res is %d\n" , a, res);
return 0;
}
C ++
#include <iostream>
using namespace std;
int main() {
int a = 10, b = 4, res;
//post-increment example:
//res is assigned 10 only, a is not updated yet
res = a++;
//a becomes 11 now
cout <<"a is " <<a<<" and res is " <<res<<"\n" ;
//post-decrement example:
//res is assigned 11 only, a is not updated yet
res = a--;
//a becomes 10 now
cout <<"a is " <<a<<" and res is " <<res<<"\n" ;
//pre-increment example:
//res is assigned 11 now since a is updated here itself
res = ++a;
//a and res have same values = 11
cout <<"a is " <<a<<" and res is " <<res<<"\n" ;
//pre-decrement example:
//res is assigned 10 only since a is updated here itself
res = --a;
//a and res have same values = 10
cout <<"a is " <<a<<" and res is " <<res<<"\n" ;
return 0;
}
输出如下:
a is 11 and res is 10
a is 10 and res is 11
a is 11 and res is 11
a is 10 and res is 10
我们很快将在不同职位上讨论其他类别的运营商。
要知道运算符优先级和关联性, 请参阅这个链接:
C语言中的运算符测验
本文由Ayush Jaggi贡献。如果发现任何不正确的地方, 或者你想分享有关上述主题的更多信息, 请发表评论