继承是OOP(面向对象编程)的重要支柱。这是Scala中的一种机制, 通过该机制, 一个类可以继承另一类的功能(字段和方法)。
重要术语:
- 超类:继承了其功能的类称为超类(或基类或父类)。
- 子类:继承另一个类的类称为子类(或派生类, 扩展类或子类)。除了超类的字段和方法, 子类还可以添加自己的字段和方法。
- 可重用性:继承支持"可重用性"的概念, 即, 当我们要创建一个新类并且已经有一个包含某些所需代码的类时, 我们可以从现有类中派生新类。通过这样做, 我们可以重用现有类的字段和方法。
如何在Scala中使用继承
用于继承的关键字是extends
语法如下:
class parent_class_name extends child_class_name{
//Methods and fields
}
例子:
//Scala program to illustrate the
//implementation of inheritance
//Base class
class Geeks 1 {
var Name : String = "Ankita"
}
//Derived class
//Using extends keyword
class Geeks 2 extends Geeks 1
{
var Article _ no : Int = 130
//Method
def details()
{
println( "Author name: " +Name);
println( "Total numbers of articles: " +Article _ no);
}
}
object Main
{
//Driver code
def main(args : Array[String])
{
//Creating object of derived class
val ob = new Geeks 2 ();
ob.details();
}
}
输出如下:
Author name: Ankita
Total numbers of articles: 130
说明:在上面的示例中, Geeks1是基类, 而Geeks2是派生类, 该派生类是使用Extended关键字从Geeks1派生的。在创建Geeks2类的对象的主要方法中, 基类的所有方法和字段的副本都会在此对象中获取内存。这就是为什么通过使用派生类的对象, 我们还可以访问基类的成员。
继承类型
以下是Scala支持的不同类型的继承。
单一继承:
在单继承中, 派生类继承一个基类的功能。在下图中, 类A用作派生类B的基类。
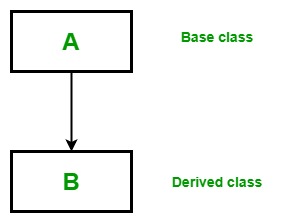
例子:
//Scala program to illustrate the
//Single inheritance
//Base class
class Parent
{
var Name : String = "Ankita"
}
//Derived class
//Using extends keyword
class Child extends Parent
{
var Age : Int = 22
//Method
def details()
{
println( "Name: " +Name);
println( "Age: " +Age);
}
}
object Main
{
//Driver code
def main(args : Array[String])
{
//Creating object of the derived class
val ob = new Child();
ob.details();
}
}
输出如下:
Name: Ankita
Age: 22
多级继承:
在"多级继承"中, 派生类将继承基类, 并且派生类还充当另一个类的基类。在下图中, 类A用作派生类B的基类, 而基类又充当派生类C的基类。
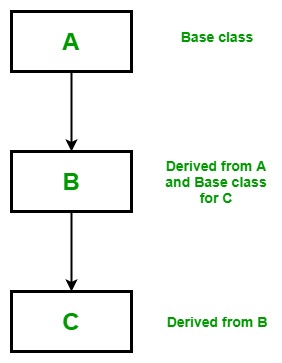
例子:
//Scala program to illustrate the
//Multilevel inheritance
//Base class
class Parent
{
var Name : String = "Soniya"
}
//Derived from parent class
//Base class for Child2 class
class Child 1 extends Parent
{
var Age : Int = 32
}
//Derived from Child1 class
class Child 2 extends Child 1
{
//Method
def details(){
println( "Name: " +Name);
println( "Age: " +Age);
}
}
object Main
{
//Drived Code
def main(args : Array[String])
{
//Creating object of the derived class
val ob = new Child 2 ();
ob.details();
}
}
输出如下:
Name: Soniya
Age: 32
层次继承:
在层次继承中, 一个类充当多个子类的超类(基类)。在下图中, 类A充当派生类B, C和D的基类。
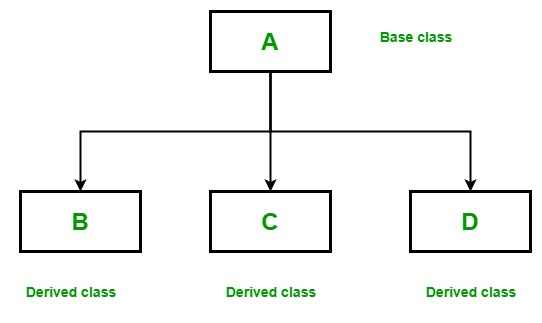
例子:
//Scala program to illustrate the
//Hierarchical inheritance
//Base class
class Parent
{
var Name 1 : String = "Siya"
var Name 2 : String = "Soniya"
}
//Derived from the parent class
class Child 1 extends Parent
{
var Age : Int = 32
def details 1 ()
{
println( " Name: " +Name 1 );
println( " Age: " +Age);
}
}
//Derived from Parent class
class Child 2 extends Parent
{
var Height : Int = 164
//Method
def details 2 ()
{
println( " Name: " +Name 2 );
println( " Height: " +Height);
}
}
object Main
{
//Driver code
def main(args : Array[String])
{
//Creating objects of both derived classes
val ob 1 = new Child 1 ();
val ob 2 = new Child 2 ();
ob 1 .details 1 ();
ob 2 .details 2 ();
}
}
输出如下:
Name: Siya
Age: 32
Name: Soniya
Height: 164
多重继承:
在多重继承中, 一个类可以具有多个父类, 并且可以从所有父类继承功能。 Scala不支持类的多重继承, 但是可以通过特征来实现。
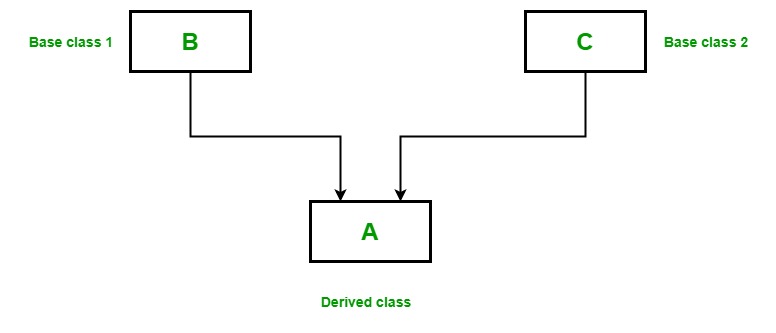
例子:
//Scala program to illustrate the
//multiple inheritance using traits
//Trait 1
trait Geeks 1
{
def method 1 ()
}
//Trait 2
trait Geeks 2
{
def method 2 ()
}
//Class that implement both Geeks1 and Geeks2 traits
class GFG extends Geeks 1 with Geeks 2
{
//method1 from Geeks1
def method 1 ()
{
println( "Trait 1" );
}
//method2 from Geeks2
def method 2 ()
{
println( "Trait 2" );
}
}
object Main
{
//Driver code
def main(args : Array[String])
{
//Creating object of GFG class
var obj = new GFG();
obj.method 1 ();
obj.method 2 ();
}
}
输出如下:
Trait 1
Trait 2
混合继承:
它是上述继承类型中的两种或多种的混合。由于Scala不支持类的多重继承, 因此混合继承也无法用于类。在Scala中, 我们只能通过特征实现混合继承。
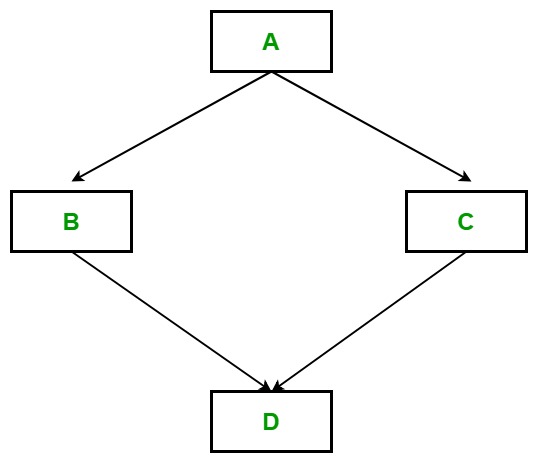