多态性一词意味着具有多种形式。简而言之, 我们可以将多态定义为消息以多种形式显示的能力。一个真实的多态示例, 一个人同时可以具有不同的特征。像男人一样, 是父亲, 丈夫, 雇员。因此, 同一个人在不同情况下具有不同的行为。这称为多态。多态被认为是面向对象编程的重要特征之一。
在C++中, 多态性主要分为两种类型:
- 编译时多态
- 运行时多态
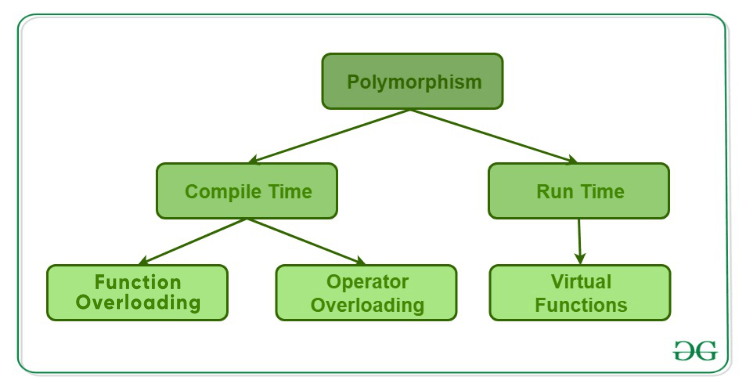
编译时多态:这种类型的多态性是通过函数重载或运算符重载来实现的。
函数重载:当有多个同名但形参不同的函数时,这些函数被称为重载。函数可以通过改变实参数量或/和实参类型来重载。
函数重载规则
// C++ program for function overloading
#include <bits/stdc++.h>
using namespace std;
class Geeks
{
public :
// function with 1 int parameter
void func( int x)
{
cout << "value of x is " << x << endl;
}
// function with same name but 1 double parameter
void func( double x)
{
cout << "value of x is " << x << endl;
}
// function with same name and 2 int parameters
void func( int x, int y)
{
cout << "value of x and y is " << x << ", " << y << endl;
}
};
int main() {
Geeks obj1;
// Which function is called will depend on the parameters passed
// The first 'func' is called
obj1.func(7);
// The second 'func' is called
obj1.func(9.132);
// The third 'func' is called
obj1.func(85, 64);
return 0;
}
输出如下:
value of x is 7
value of x is 9.132
value of x and y is 85, 64
在上面的例子中,一个名为func的函数在三种不同的情况下有不同的作用,这就是多态性的特性。
运算符重载:C++还提供了重载运算符的选项。例如, 我们可以使字符串类的运算符(" +")连接两个字符串。我们知道这是加法运算符, 其任务是将两个操作数相加。因此, 将单个运算符" +"放在整数操作数之间时, 将它们相加, 而放在字符串操作数之间时, 则将它们连接起来。
例子:
// CPP program to illustrate
// Operator Overloading
#include<iostream>
using namespace std;
class Complex {
private :
int real, imag;
public :
Complex( int r = 0, int i =0) {real = r; imag = i;}
// This is automatically called when '+' is used with
// between two Complex objects
Complex operator + (Complex const &obj) {
Complex res;
res.real = real + obj.real;
res.imag = imag + obj.imag;
return res;
}
void print() { cout << real << " + i" << imag << endl; }
};
int main()
{
Complex c1(10, 5), c2(2, 4);
Complex c3 = c1 + c2; // An example call to "operator+"
c3.print();
}
输出如下:
12 + i9
在上面的示例中, 运算符" +"超载。运算符" +"是加法运算符, 可以将两个数字(整数或浮点数)相加, 但此处使该运算符执行两个虚数或复数的加法运算。要了解操作员重载的详细信息, 请访问这个链接。
运行时多态:这种类型的多态性是通过功能覆盖实现的。
函数覆盖:另一方面,当派生类具有基类成员函数之一的定义时,则发生函数重写。我们说这个基函数被覆盖了。
// C++ program for function overriding
#include <bits/stdc++.h>
using namespace std;
class base
{
public :
virtual void print ()
{ cout<< "print base class" <<endl; }
void show ()
{ cout<< "show base class" <<endl; }
};
class derived: public base
{
public :
void print () //print () is already virtual function in derived class, we could also declared as virtual void print () explicitly
{ cout<< "print derived class" <<endl; }
void show ()
{ cout<< "show derived class" <<endl; }
};
//main function
int main()
{
base *bptr;
derived d;
bptr = &d;
//virtual function, binded at runtime (Runtime polymorphism)
bptr->print();
// Non-virtual function, binded at compile time
bptr->show();
return 0;
}
输出如下:
print derived class
show base class
要详细了解运行时多态性, 请访问这个链接。
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。
被认为是行业中最受欢迎的技能之一, 我们拥有自己的编码基础C++ STL通过激烈的问题解决过程来训练和掌握这些概念。