本文概述
封装是面向对象编程(OOP)中的基本概念之一。它描述了包装数据的思想以及在一个单元中处理数据的方法。这对直接访问变量和方法施加了限制, 并可以防止意外修改数据。为防止意外更改, 只能通过对象的方法更改对象的变量。这些类型的变量称为
私人变量
.
类是封装的一个示例, 因为它封装了所有数据, 这些数据是成员函数, 变量等。
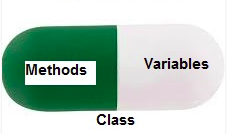
考虑一个封装的真实示例, 在公司中, 有不同的部分, 例如帐户部分, 财务部分, 销售部分等。财务部分处理所有财务交易并保留与财务相关的所有数据的记录。同样, 销售部门处理所有与销售有关的活动, 并保留所有销售记录。现在可能会出现这样的情况:出于某种原因, 财务部门的官员需要特定月份的所有销售数据。在这种情况下, 不允许他直接访问销售部分的数据。他将首先必须联系销售部门的其他人员, 然后要求他提供特定数据。这就是封装。在这里, 销售部门和可以操纵它们的员工的数据被包装在一个单独的名称"销售部门"下。使用封装还会隐藏数据。在此示例中, 诸如销售, 财务或帐户之类的部分的数据对于任何其他部分都是隐藏的。
受保护的成员
受保护的成员(在C ++和JAVA中)是不能在类外部访问但可以从类及其子类内部访问的那些成员。要在Python中完成此操作, 只需遵循
惯例
通过在成员名称前面加上一个
单下划线" _"
.
注意:
__init__方法是一个构造函数, 并在实例化一个类的对象后立即运行。
Python3
# Python program to
# demonstrate protected members
# Creating a base class
class Base:
def __init__( self ):
# Protected member
self ._a = 2
# Creating a derived class
class Derived(Base):
def __init__( self ):
# Calling constructor of
# Base class
Base.__init__( self )
print ( "Calling protected member of base class: " )
print ( self ._a)
obj1 = Derived()
obj2 = Base()
# Calling protected member
# Outside class will result in
# AttributeError
print (obj2.a)
输出如下:
Calling protected member of base class:
2
Traceback (most recent call last):
File "/home/6fb1b95dfba0e198298f9dd02469eb4a.py", line 25, in
print(obj1.a)
AttributeError: 'Base' object has no attribute 'a'
私人会员
私有成员与受保护的成员相似, 不同之处在于, 声明为私有的类成员既不应在类外部也不能被任何基类访问。在Python中, 不存在
private
实例变量, 只能在类内部访问。但是, 要定义私有成员, 请在成员名称前加上双下划线" __"。
注意:
可以在课程外通过以下方式访问Python的私有成员和受保护成员
python名称修改
.
Python3
# Python program to
# demonstrate private members
# Creating a Base class
class Base:
def __init__( self ):
self .a = "lsbin"
self .__c = "lsbin"
# Creating a derived class
class Derived(Base):
def __init__( self ):
# Calling constructor of
# Base class
Base.__init__( self )
print ( "Calling private member of base class: " )
print ( self .__c)
# Driver code
obj1 = Base()
print (obj1.a)
# Uncommenting print(obj1.c) will
# raise an AttributeError
# Uncommenting obj2 = Derived() will
# also raise an AtrributeError as
# private member of base class
# is called inside derived class
输出如下:
lsbin
Traceback (most recent call last):
File "/home/f4905b43bfcf29567e360c709d3c52bd.py", line 25, in <module>
print(obj1.c)
AttributeError: 'Base' object has no attribute 'c'
Traceback (most recent call last):
File "/home/4d97a4efe3ea68e55f48f1e7c7ed39cf.py", line 27, in <module>
obj2 = Derived()
File "/home/4d97a4efe3ea68e55f48f1e7c7ed39cf.py", line 20, in __init__
print(self.__c)
AttributeError: 'Derived' object has no attribute '_Derived__c'
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。