先决条件: Python GUI – Tkinter
YouTube是一个非常受欢迎的视频共享网站。从YouTube下载视频/播放列表是一项繁琐的任务。通过Downloader下载该视频或尝试从随机网站下载该视频, 这会增加舔你个人数据的风险。使用Python Tkinter包, 此任务非常简单, 高效, 安全。几乎没有群码可以为你下载视频。为此, 有两个Python库–Tkinter和pytube.
所需模块
pytube:
pytube是一个轻量级, 易于使用, 无依赖的Python库, 用于从web.pytube下载视频, 而不是自动配置的库。你需要先安装它, 然后才能使用它。当你拥有pip时, 安装pytube很容易。在终端或命令提示符中, 键入以下命令以安装pytube。
如果你使用的是Mac OS X或Linux, 则以下两个命令之一可能会为你工作:
pip安装pytube git clone git://github.com/NFicano/pytube.git pytube | cd pytube | python setup.py安装
如果你在Window的
pip install pytube3
Tkinter
:
Tkinter是Python与Tk GUI工具包的绑定。它是Tk GUI工具包的标准Python界面, 或者简单地说Tkinter用作Python图形用户界面。 Tkinter是本机库, 使用时无需外部安装, 只需导入即可。
下面是实现。
# Importing necessary packages
import tkinter as tk
from tkinter import *
from pytube import YouTube
from tkinter import messagebox, filedialog
# Defining CreateWidgets() function
# to create necessary tkinter widgets
def Widgets():
link_label = Label(root, text = "YouTube link :" , bg = "#E8D579" )
link_label.grid(row = 1 , column = 0 , pady = 5 , padx = 5 )
root.linkText = Entry(root, width = 55 , textvariable = video_Link)
root.linkText.grid(row = 1 , column = 1 , pady = 5 , padx = 5 , columnspan = 2 )
destination_label = Label(root, text = "Destination :" , bg = "#E8D579" )
destination_label.grid(row = 2 , column = 0 , pady = 5 , padx = 5 )
root.destinationText = Entry(root, width = 40 , textvariable = download_Path)
root.destinationText.grid(row = 2 , column = 1 , pady = 5 , padx = 5 )
browse_B = Button(root, text = "Browse" , command = Browse, width = 10 , bg = "#05E8E0" )
browse_B.grid(row = 2 , column = 2 , pady = 1 , padx = 1 )
Download_B = Button(root, text = "Download" , command = Download, width = 20 , bg = "#05E8E0" )
Download_B.grid(row = 3 , column = 1 , pady = 3 , padx = 3 )
# Defining Browse() to select a
# destination folder to save the video
def Browse():
# Presenting user with a pop-up for
# directory selection. initialdir
# argument is optional Retrieving the
# user-input destination directory and
# storing it in downloadDirectory
download_Directory = filedialog.askdirectory(initialdir = "YOUR DIRECTORY PATH" )
# Displaying the directory in the directory
# textbox
download_Path. set (download_Directory)
# Defining Download() to download the video
def Download():
# getting user-input Youtube Link
Youtube_link = video_Link.get()
# select the optimal location for
# saving file's
download_Folder = download_Path.get()
# Creating object of YouTube()
getVideo = YouTube(Youtube_link)
# Getting all the available streams of the
# youtube video and selecting the first
# from the
videoStream = getVideo.streams.first()
# Downloading the video to destination
# directory
videoStream.download(download_Folder)
# Displaying the message
messagebox.showinfo( "SUCCESSFULLY" , "DOWNLOADED AND SAVED IN\n"
+ download_Folder)
# Creating object of tk class
root = tk.Tk()
# Setting the title, background color
# and size of the tkinter window and
# disabling the resizing property
root.geometry( "600x120" )
root.resizable( False , False )
root.title( "YouTube_Video_Downloader" )
root.config(background = "#000000" )
# Creating the tkinter Variables
video_Link = StringVar()
download_Path = StringVar()
# Calling the Widgets() function
Widgets()
# Defining infinite loop to run
# application
root.mainloop()
输出如下:
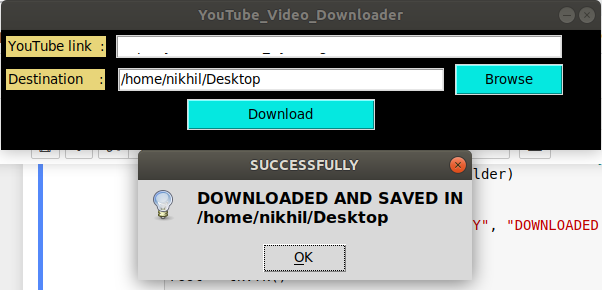
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。