JavaScript提供了许多字符串方法, 用于检查一个字符串是否为其他字符串的子字符串。因此, jQuery根本不需要执行此任务。但是, 我们将介绍检查字符串是否以字符串开头或结尾的所有不同方法:
- startsWith()和endsWith()方法
- search()方法
- indexOf()方法
- substring()方法
- substr()方法
- slice()方法
让我们考虑一个字符串str ="欢迎使用GEEKS!。现在我们要确定字符串str是否以startword ="欢迎"并以endword =" !!!".
方法:
tartsWith()和endsWith()方法:检查字符串是以特定子字符串开始还是以特定子字符串结束。
例子:
if (str.startsWith(startword) && str.endsWith(endword)) {
// case sensitive
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
search()方法:它检查另一个字符串中是否包含特定字符串, 并返回该子字符串的起始索引。
例子:
if (str.search(startword)==0 &&
str.search(endword)==stringlength-endwordlength ) {
// case sensitive
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
indexOf()方法:顾名思义, 它在字符串中找到子字符串的起始索引。
例子:
if (str.indexOf(startword)==0 &&
str.indexOf(endword)==stringlength-endwordlength) {
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
substring()方法:它返回起始索引和结束索引之间存在的字符串。
例子:
if (str.substring(0, startwordlength)==startword
&& str.substring(stringlength-endwordlength, stringlength)==endword) {
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
substr()方法:它类似于substring()方法, 但以起始索引和子字符串的长度作为参数。
范例:
if (str.substr(0, startwordlength)==startword
&& str.substr(stringlength-endwordlength, endwordlength)==endword) {
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
slice()方法:此方法返回字符串中任意两个索引之间的字符串切片。
例子:
if (str.slice(0, startwordlength)==startword
&& str.slice(stringlength-endwordlength, stringlength)==endword) {
console.log( "Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword);
}
让我们以h1元素中的文本为例, 检查它是否以给定的子字符串开头和结尾。
<!DOCTYPE html>
<html>
<head>
<title>
How to know that a string starts/ends
with a specific string in jQuery?
</title>
<script src= "https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js" >
</script>
</head>
<body>
<h1 id= "text" >
Welcome To GEEKS FOR GEEKS!!!
</h1>
<h2>
Check whether a word is present
in the above sentence
</h2>
<input type= "text" id= "startkey" >
<input type= "text" id= "endkey" >
<button onclick= "MyFunction()" > Check </button>
<h2> startsWith() and endsWith() method </h2>
<h2 id= "startswith" > </h2>
<h2> indexOf() method </h2>
<h2 id= "indexOf" ></h2>
<h2> search() method </h2>
<h2 id= "search" ></h2>
<h2> substring() method </h2>
<h2 id= "substring" ></h2>
<h2> substr() method </h2>
<h2 id= "substr" > </h2>
<h2> slice() method </h2>
<h2 id= "slice" ></h2>
<script>
var str = document.getElementById( "text" ).innerText;
stringlength = str.length;
var startword = "" ;
var endword = "" ;
function MyFunction() {
startword = document.getElementById( "startkey" ).value;
endword = document.getElementById( "endkey" ).value;
console.log(startword);
console.log(endword)
startwordlength = startword.length;
endwordlength = endword.length;
if (str.startsWith(startword) && str.endsWith(endword)) {
// case sensitive
var h2 = document.getElementById( "startswith" );
h2.innerHTML =
"Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword;
}
else {
var h2 = document.getElementById( "startswith" );
h2.innerHTML =
"No, The string " + str +
" doesn't start and endwith the given words" ;
}
//Js
if (str.indexOf(startword) == 0 && str.indexOf(
endword) == stringlength - endwordlength) {
var h2 = document.getElementById( "indexOf" );
h2.innerHTML =
"Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword;
}
else {
var h2 = document.getElementById( "indexOf" );
h2.innerHTML =
"No, The string " + str +
" doesn't start and endwith the given words" ;
}
//Js
if (str.search(
startword) == 0 && str.search(endword)
== stringlength - endwordlength) {
// case sensitive
var h2 = document.getElementById( "search" );
h2.innerHTML = "Yes, The string " + str +
" starts with " + startword +
" and ends with " + endword;
}
else {
var h2 = document.getElementById( "search" );
h2.innerHTML = "No, The string " + str +
" doesn't start and endwith the given words" ;
}
//Js
if (str.substring(
0, startwordlength) == startword && str.substring(
stringlength - endwordlength, stringlength) == endword) {
var h2 = document.getElementById( "substring" );
h2.innerHTML =
"Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword;
}
else {
var h2 = document.getElementById( "substring" );
h2.innerHTML =
"No, The string " + str +
" doesn't start and endwith the given words" ;
}
if (str.substr(
0, startwordlength) == startword && str.substr(
stringlength - endwordlength, endwordlength) == endword) {
var h2 = document.getElementById( "substr" );
h2.innerHTML =
"Yes, The string " + str + " starts with "
+ startword + " and ends with " + endword;
}
else {
var h2 = document.getElementById( "substr" );
h2.innerHTML =
"No, The string " + str +
" doesn't start and endwith the given words" ;
}
if (str.slice(
0, startwordlength) == startword && str.slice(
stringlength - endwordlength, stringlength) == endword) {
var h2 = document.getElementById( "slice" );
h2.innerHTML =
"Yes, The string " + str + " starts with " +
startword + " and ends with " + endword;
}
else {
var h2 = document.getElementById( "slice" );
h2.innerHTML =
"No, The string " + str +
" doesn't start and endwith the given words" ;
}
}
</script>
</body>
</html>
输出如下:
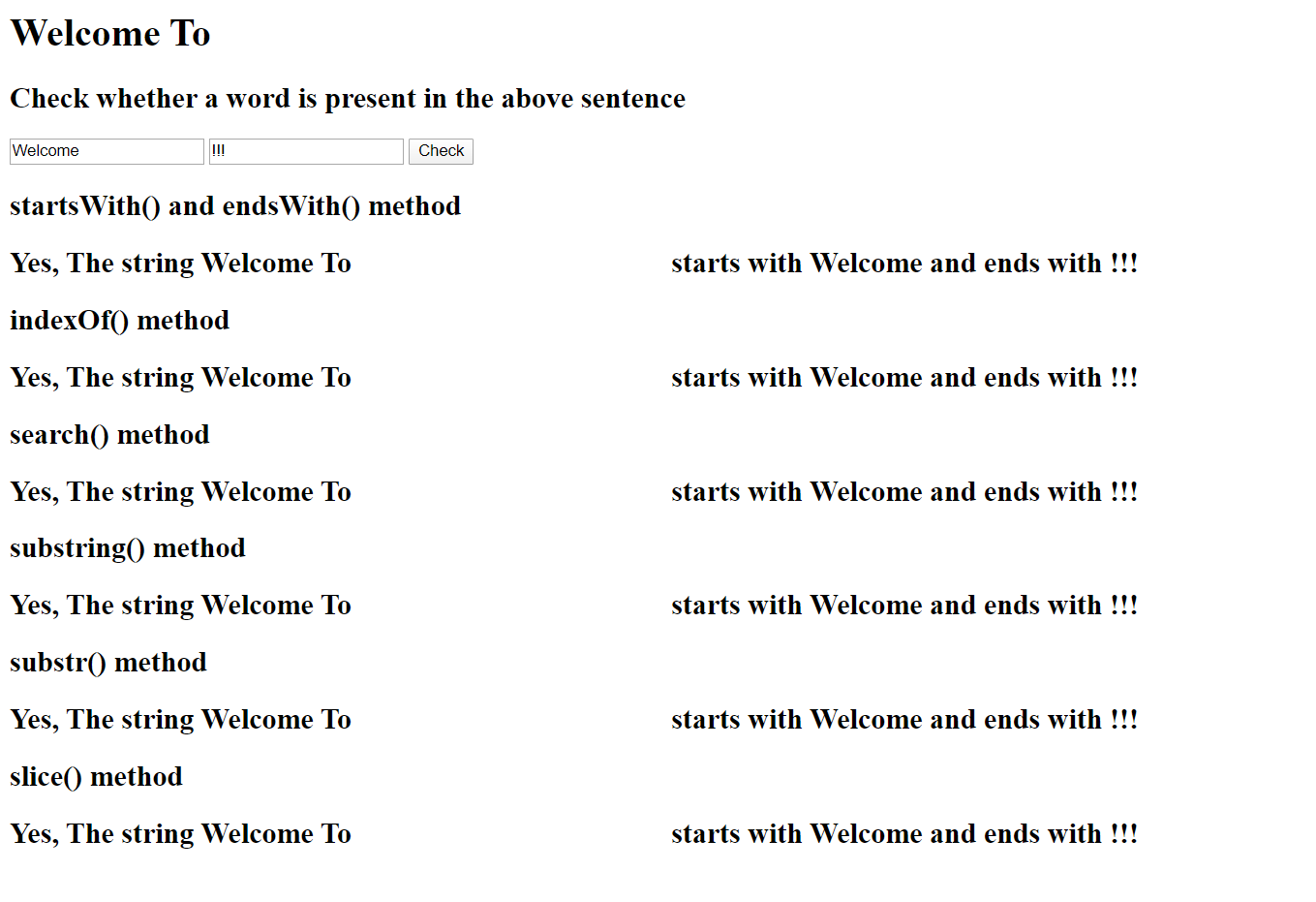
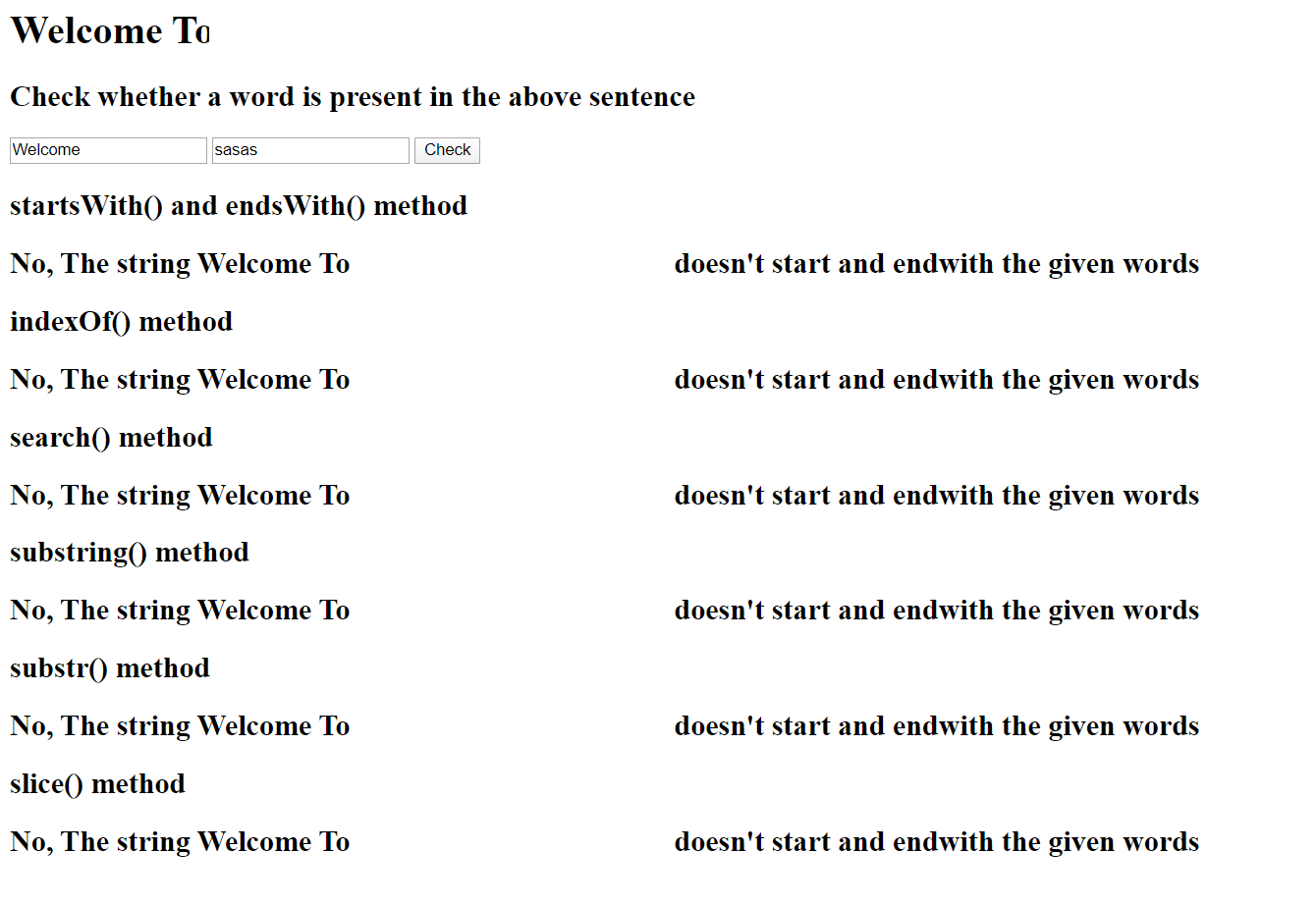