本文概述
tuple
是Python对象的集合, 非常类似于列表。存储在元组中的值的序列可以是任何类型, 并由整数索引。
元组的值在语法上以"逗号"分隔。尽管不是必需的, 但更常见的是通过关闭括号中的值序列来定义元组。这有助于更轻松地理解Python元组。
创建一个元组
在Python中, 元组是通过放置值序列(用"逗号"分隔)(使用或不使用括号将数据序列分组)来创建的。
注意 -不使用括号创建Python元组称为Tuple Packing。
Python程序, 用于演示在元组中添加元素。
#Creating an empty Tuple
Tuple1 = ()
print ( "Initial empty Tuple: " )
print (Tuple1)
#Creatting a Tuple
#with the use of string
Tuple1 = ( 'Geeks' , 'For' )
print ( "\nTuple with the use of String: " )
print (Tuple1)
# Creating a Tuple with
# the use of list
list1 = [ 1 , 2 , 4 , 5 , 6 ]
print ( "\nTuple using List: " )
print ( tuple (list1))
#Creating a Tuple
#with the use of built-in function
Tuple1 = tuple ( 'Geeks' )
print ( "\nTuple with the use of function: " )
print (Tuple1)
输出如下:
Initial empty Tuple:
()
Tuple with the use of String:
('Geeks', 'For')
Tuple using List:
(1, 2, 4, 5, 6)
Tuple with the use of function:
('G', 'e', 'e', 'k', 's')
创建具有混合数据类型的元组。
元组可以包含任意数量的元素和任何数据类型(例如字符串, 整数, 列表等)。元组也可以用单个元素创建, 但是有点棘手。括号中仅包含一个元素是不够的, 必须在其后加上一个"逗号"以使其成为一个元组。
#Creating a Tuple
#with Mixed Datatype
Tuple1 = ( 5 , 'Welcome' , 7 , 'Geeks' )
print ( "\nTuple with Mixed Datatypes: " )
print (Tuple1)
#Creating a Tuple
#with nested tuples
Tuple1 = ( 0 , 1 , 2 , 3 )
Tuple2 = ( 'python' , 'geek' )
Tuple3 = (Tuple1, Tuple2)
print ( "\nTuple with nested tuples: " )
print (Tuple3)
#Creating a Tuple
#with repetition
Tuple1 = ( 'Geeks' , ) * 3
print ( "\nTuple with repetition: " )
print (Tuple1)
#Creating a Tuple
#with the use of loop
Tuple1 = ( 'Geeks' )
n = 5
print ( "\nTuple with a loop" )
for i in range ( int (n)):
Tuple1 = (Tuple1, )
print (Tuple1)
输出如下:
Tuple with Mixed Datatypes:
(5, 'Welcome', 7, 'Geeks')
Tuple with nested tuples:
((0, 1, 2, 3), ('python', 'geek'))
Tuple with repetition:
('Geeks', 'Geeks', 'Geeks')
Tuple with a loop
('Geeks', )
(('Geeks', ), )
((('Geeks', ), ), )
(((('Geeks', ), ), ), )
((((('Geeks', ), ), ), ), )
访问元组
元组是不可变的, 通常, 它们包含一系列异类元素, 可通过开箱或索引(对于命名元组, 甚至是按属性)。列表是可变的, 并且它们的元素通常是同类的, 并且可以通过遍历列表来访问。
注意:在对元组进行解包时, 左侧的变量数应等于给定元组a中的值数。
#Accessing Tuple
#with Indexing
Tuple1 = tuple ( "Geeks" )
print ( "\nFirst element of Tuple: " )
print (Tuple1[ 1 ])
#Tuple unpacking
Tuple1 = ( "Geeks" , "For" , "Geeks" )
#This line unpack
#values of Tuple1
a, b, c = Tuple1
print ( "\nValues after unpacking: " )
print (a)
print (b)
print (c)
输出如下:
First element of Tuple:
e
Values after unpacking:
Geeks
For
Geeks
元组的串联
元组的串联是两个或多个元组的连接过程。串联是使用" +"运算符完成的。元组的连接总是从原始元组的末尾开始。其他算术运算不适用于元组。
注意-
只能将相同的数据类型与串联组合, 如果将列表和元组组合, 则会出现错误。
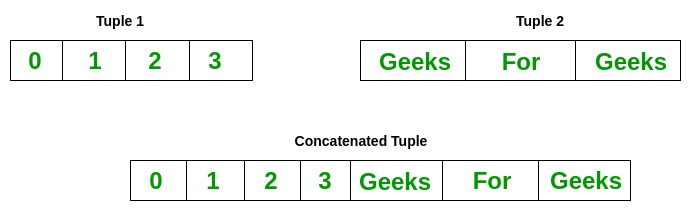
# Concatenaton of tuples
Tuple1 = ( 0 , 1 , 2 , 3 )
Tuple2 = ( 'Geeks' , 'For' , 'Geeks' )
Tuple3 = Tuple1 + Tuple2
# Printing first Tuple
print ( "Tuple 1: " )
print (Tuple1)
# Printing Second Tuple
print ( "\nTuple2: " )
print (Tuple2)
# Printing Final Tuple
print ( "\nTuples after Concatenaton: " )
print (Tuple3)
输出如下:
Tuple 1:
(0, 1, 2, 3)
Tuple2:
('Geeks', 'For', 'Geeks')
Tuples after Concatenaton:
(0, 1, 2, 3, 'Geeks', 'For', 'Geeks')
元组切片
完成元组的切片以从元组中获取特定范围或子元素的切片。也可以对列表和数组进行切片。在列表中建立索引导致获取单个元素, 而切片允许获取一组元素。
注意-
负增量值也可以用于反转元组的顺序
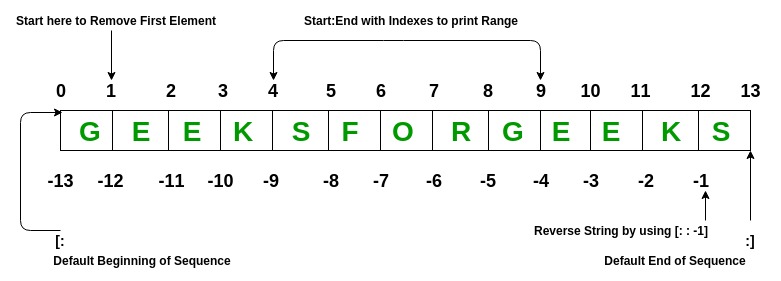
# Slicing of a Tuple
# Slicing of a Tuple
# with Numbers
Tuple1 = tuple ( 'lsbin' )
# Removing First element
print ( "Removal of First Element: " )
print (Tuple1[ 1 :])
# Reversing the Tuple
print ( "\nTuple after sequence of Element is reversed: " )
print (Tuple1[:: - 1 ])
# Printing elements of a Range
print ( "\nPrinting elements between Range 4-9: " )
print (Tuple1[ 4 : 9 ])
输出如下:
Removal of First Element:
('E', 'E', 'K', 'S', 'F', 'O', 'R', 'G', 'E', 'E', 'K', 'S')
Tuple after sequence of Element is reversed:
('S', 'K', 'E', 'E', 'G', 'R', 'O', 'F', 'S', 'K', 'E', 'E', 'G')
Printing elements between Range 4-9:
('S', 'F', 'O', 'R', 'G')
删除元组
元组是不可变的, 因此不允许删除其中的一部分。通过使用del()方法可以删除整个元组。
注意-
删除后打印元组将导致错误。
# Deleting a Tuple
Tuple1 = ( 0 , 1 , 2 , 3 , 4 )
del Tuple1
print (Tuple1)
追溯(最近一次通话最近):文件" /home/efa50fd0709dec08434191f32275928a.py", 第7行, 在print(Tuple1)中显示名称错误:未定义名称" Tuple1"
内置方法
内建功能 | 描述 |
---|---|
所有() | 如果所有元素都为true或tuple为空, 则返回true |
任何() | 如果元组的任何元素为true, 则返回true。如果元组为空, 则返回false |
len() | 返回元组的长度或元组的大小 |
枚举() | 返回元组的枚举对象 |
max() | 返回给定元组的最大元素 |
min() | 返回给定元组的最小元素 |
和() | 对元组中的数字求和 |
sorted() | 在元组中输入元素并返回新的排序列表 |
元组() | 将iterable转换为元组。 |
关于元组的最新文章
元组程序
- 在给定的布尔字符串中打印唯一行
- 程序根据给定的字符串生成所有可能的有效IP地址
- Python字典查找字符串中的镜像字符
- 根据Python中输入字符串中字符的出现情况生成两个输出字符串
- Python groupby方法删除所有连续的重复项
- 将字符列表转换为字符串
- 从列表中删除空元组
- 反转元组
- Python设置symmetric_difference()
- 将元组列表转换成字典
- 按其float元素对元组进行排序
- 计算元组中元素的出现
- 计算列表中的元素, 直到元素是元组为止
- 按任意键按升序对元组进行排序
- Python中的命名元组
有用的链接
- Python程序输出
- 关于Python元组的最新文章
- 多项选择题– Python
- Python类别中的所有文章
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。