本文概述
Vigenere密码是一种加密字母文本的方法。它使用一种简单的形式多字母替代。多字母密码是任何基于替换的密码, 使用多个替换字母。原始文本的加密使用维吉涅广场或维吉涅桌.
- 该表由在不同行中写出26次的字母组成, 每个字母与上一个字母相比循环向左移动, 对应于26个可能的字母凯撒密码.
- 在加密过程的不同点, 密码使用的密码与行之一不同。
- 在每个点使用的字母取决于重复的关键字。
例子:
Input : Plaintext : lsbin
Keyword : AYUSH
Output : Ciphertext : GCYCZFMLYLEIM
For generating key, the given keyword is repeated
in a circular manner until it matches the length of
the plain text.
The keyword "AYUSH" generates the key "AYUSHAYUSHAYU"
The plain text is then encrypted using the process
explained below.
加密
明文的第一个字母G与密钥的第一个字母A配对。因此, 请使用Vigenère正方形的G行和A列, 即G。类似地, 对于明文的第二个字母, 使用键的第二个字母, E行和Y列的字母为C。其余的明文以类似的方式被加密。
加密表–极客
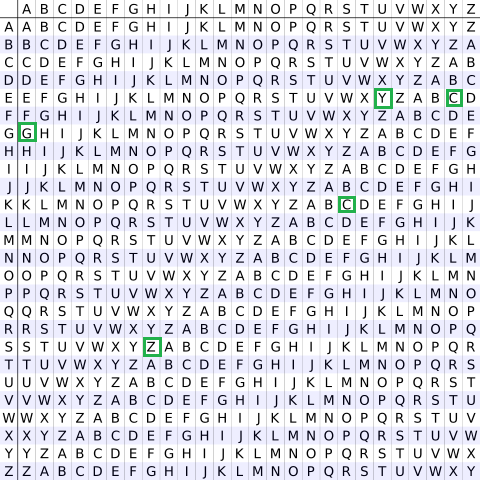
解密
解密是通过以下步骤进行的:转到表中与密钥对应的行, 找到密文字母在该行中的位置, 然后将该列的标签用作纯文本。例如, 在A行(来自AYUSH)中, 密文G出现在G列中, 这是第一个明文字母。接下来我们转到Y行(来自AYUSH), 找到在E列中找到的密文C, 因此E是第二个明文字母。
更多易于实施通过将[A-Z]转换为数字[0-25], 可以可视化Vigenère代数。
Encryption
The plaintext(P) and key(K) are added modulo 26.
Ei = (Pi + Ki) mod 26
Decryption
Di = (Ei - Ki + 26) mod 26
注意:d一世表示明文的第i个字符的偏移量。像偏移一种是0和乙是1, 依此类推。
以下是该想法的实现。
C ++
// C++ code to implement Vigenere Cipher
#include<bits/stdc++.h>
using namespace std;
// This function generates the key in
// a cyclic manner until it's length isi'nt
// equal to the length of original text
string generateKey(string str, string key)
{
int x = str.size();
for ( int i = 0; ; i++)
{
if (x == i)
i = 0;
if (key.size() == str.size())
break ;
key.push_back(key[i]);
}
return key;
}
// This function returns the encrypted text
// generated with the help of the key
string cipherText(string str, string key)
{
string cipher_text;
for ( int i = 0; i < str.size(); i++)
{
// converting in range 0-25
char x = (str[i] + key[i]) %26;
// convert into alphabets(ASCII)
x += 'A' ;
cipher_text.push_back(x);
}
return cipher_text;
}
// This function decrypts the encrypted text
// and returns the original text
string originalText(string cipher_text, string key)
{
string orig_text;
for ( int i = 0 ; i < cipher_text.size(); i++)
{
// converting in range 0-25
char x = (cipher_text[i] - key[i] + 26) %26;
// convert into alphabets(ASCII)
x += 'A' ;
orig_text.push_back(x);
}
return orig_text;
}
// Driver program to test the above function
int main()
{
string str = "lsbin" ;
string keyword = "AYUSH" ;
string key = generateKey(str, keyword);
string cipher_text = cipherText(str, key);
cout << "Ciphertext : "
<< cipher_text << "\n" ;
cout << "Original/Decrypted Text : "
<< originalText(cipher_text, key);
return 0;
}
Java
// Java code to implement Vigenere Cipher
class GFG
{
// This function generates the key in
// a cyclic manner until it's length isi'nt
// equal to the length of original text
static String generateKey(String str, String key)
{
int x = str.length();
for ( int i = 0 ; ; i++)
{
if (x == i)
i = 0 ;
if (key.length() == str.length())
break ;
key+=(key.charAt(i));
}
return key;
}
// This function returns the encrypted text
// generated with the help of the key
static String cipherText(String str, String key)
{
String cipher_text= "" ;
for ( int i = 0 ; i < str.length(); i++)
{
// converting in range 0-25
int x = (str.charAt(i) + key.charAt(i)) % 26 ;
// convert into alphabets(ASCII)
x += 'A' ;
cipher_text+=( char )(x);
}
return cipher_text;
}
// This function decrypts the encrypted text
// and returns the original text
static String originalText(String cipher_text, String key)
{
String orig_text= "" ;
for ( int i = 0 ; i < cipher_text.length() &&
i < key.length(); i++)
{
// converting in range 0-25
int x = (cipher_text.charAt(i) -
key.charAt(i) + 26 ) % 26 ;
// convert into alphabets(ASCII)
x += 'A' ;
orig_text+=( char )(x);
}
return orig_text;
}
// Driver code
public static void main(String[] args)
{
String str = "lsbin" ;
String keyword = "AYUSH" ;
String key = generateKey(str, keyword);
String cipher_text = cipherText(str, key);
System.out.println( "Ciphertext : "
+ cipher_text + "\n" );
System.out.println( "Original/Decrypted Text : "
+ originalText(cipher_text, key));
}
}
// This code has been contributed by 29AjayKumar
Python3
# Python code to implement
# Vigenere Cipher
# This function generates the
# key in a cyclic manner until
# it's length isn't equal to
# the length of original text
def generateKey(string, key):
key = list (key)
if len (string) = = len (key):
return (key)
else :
for i in range ( len (string) -
len (key)):
key.append(key[i % len (key)])
return ("" . join(key))
# This function returns the
# encrypted text generated
# with the help of the key
def cipherText(string, key):
cipher_text = []
for i in range ( len (string)):
x = ( ord (string[i]) +
ord (key[i])) % 26
x + = ord ( 'A' )
cipher_text.append( chr (x))
return ("" . join(cipher_text))
# This function decrypts the
# encrypted text and returns
# the original text
def originalText(cipher_text, key):
orig_text = []
for i in range ( len (cipher_text)):
x = ( ord (cipher_text[i]) -
ord (key[i]) + 26 ) % 26
x + = ord ( 'A' )
orig_text.append( chr (x))
return ("" . join(orig_text))
# Driver code
if __name__ = = "__main__" :
string = "lsbin"
keyword = "AYUSH"
key = generateKey(string, keyword)
cipher_text = cipherText(string, key)
print ( "Ciphertext :" , cipher_text)
print ( "Original/Decrypted Text :" , originalText(cipher_text, key))
# This code is contributed
# by Pratik Somwanshi
C#
// C# code to implement Vigenere Cipher
using System;
class GFG
{
// This function generates the key in
// a cyclic manner until it's length isi'nt
// equal to the length of original text
static String generateKey(String str, String key)
{
int x = str.Length;
for ( int i = 0; ; i++)
{
if (x == i)
i = 0;
if (key.Length == str.Length)
break ;
key+=(key[i]);
}
return key;
}
// This function returns the encrypted text
// generated with the help of the key
static String cipherText(String str, String key)
{
String cipher_text= "" ;
for ( int i = 0; i < str.Length; i++)
{
// converting in range 0-25
int x = (str[i] + key[i]) %26;
// convert into alphabets(ASCII)
x += 'A' ;
cipher_text+=( char )(x);
}
return cipher_text;
}
// This function decrypts the encrypted text
// and returns the original text
static String originalText(String cipher_text, String key)
{
String orig_text= "" ;
for ( int i = 0 ; i < cipher_text.Length &&
i < key.Length; i++)
{
// converting in range 0-25
int x = (cipher_text[i] -
key[i] + 26) %26;
// convert into alphabets(ASCII)
x += 'A' ;
orig_text+=( char )(x);
}
return orig_text;
}
// Driver code
public static void Main(String[] args)
{
String str = "lsbin" ;
String keyword = "AYUSH" ;
String key = generateKey(str, keyword);
String cipher_text = cipherText(str, key);
Console.WriteLine( "Ciphertext : "
+ cipher_text + "\n" );
Console.WriteLine( "Original/Decrypted Text : "
+ originalText(cipher_text, key));
}
}
/* This code contributed by PrinciRaj1992 */
输出如下:
Ciphertext : GCYCZFMLYLEIM
Original/Decrypted Text : lsbin
参考:https://en.wikipedia.org/wiki/Vigen%C3%A8re_cipher