数组是存储在连续内存位置的项目的集合。想法是将相同类型的多个项目存储在一起。通过简单地将偏移量添加到基本值(即数组的第一个元素的存储位置(通常由数组的名称表示)), 可以更轻松地计算每个元素的位置。
为简单起见, 我们可以考虑一系列楼梯, 在每个楼梯上都放置一个值(假设你的一个朋友)。在这里, 你只需知道朋友走过的步数即可确定他们的位置。数组可以在Python中由名为的模块处理
Array
。当我们只需要操作特定的数据类型值时, 它们就很有用。用户可以治疗
列表
作为数组。但是, 用户不能限制列表中存储的元素的类型。如果你使用
Array
模块, 数组的所有元素必须是同一类型。
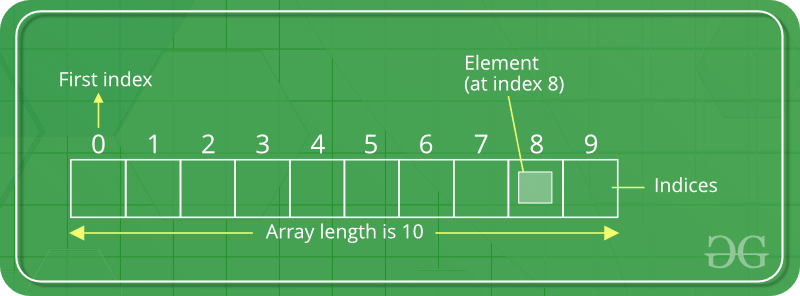
创建一个数组
Python中的数组可以通过导入来创建Array模块。数组(数据类型, value_list)用于创建具有在其参数中指定的数据类型和值列表的数组。
# Python program to demonstrate
# Creation of Array
# importing "array" for array creations
import array as arr
# creating an array with integer type
a = arr.array( 'i' , [ 1 , 2 , 3 ])
# printing original array
print ( "The new created array is : " , end = " " )
for i in range ( 0 , 3 ):
print (a[i], end = " " )
print ()
# creating an array with float type
b = arr.array( 'd' , [ 2.5 , 3.2 , 3.3 ])
# printing original array
print ( "The new created array is : " , end = " " )
for i in range ( 0 , 3 ):
print (b[i], end = " " )
输出:
The new created array is : 1 2 3
The new created array is : 2.5 3.2 3.3
下面提到一些数据类型, 这将有助于创建不同数据类型的数组。
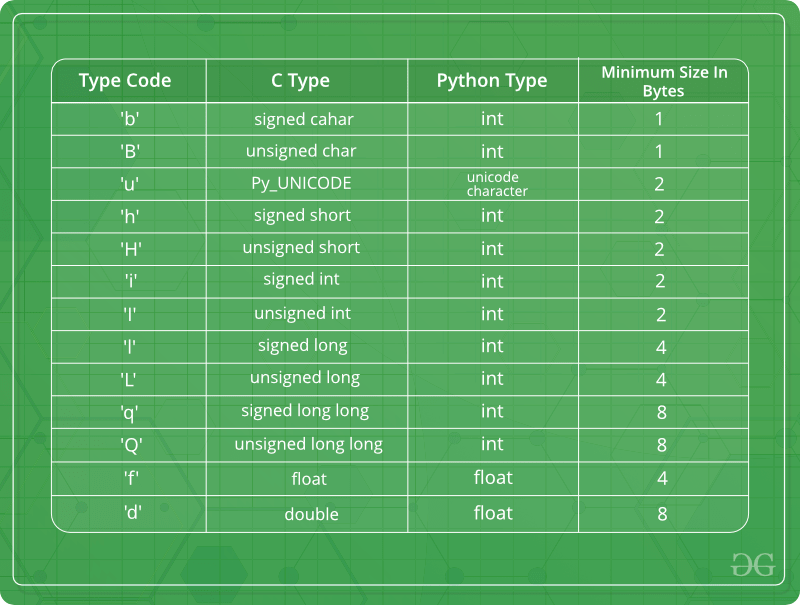
将元素添加到数组
可以使用内置元素将元素添加到数组插入()功能。插入用于将一个或多个数据元素插入数组。根据要求, 可以在数组的开始, 结尾或任何给定的索引处添加新元素。附加()还用于在数组末尾添加其参数中提到的值。
# Python program to demonstrate
# Adding Elements to a Array
# importing "array" for array creations
import array as arr
# array with int type
a = arr.array( 'i' , [ 1 , 2 , 3 ])
print ( "Array before insertion : " , end = " " )
for i in range ( 0 , 3 ):
print (a[i], end = " " )
print ()
# inserting array using
# insert() function
a.insert( 1 , 4 )
print ( "Array after insertion : " , end = " " )
for i in (a):
print (i, end = " " )
print ()
# array with float type
b = arr.array( 'd' , [ 2.5 , 3.2 , 3.3 ])
print ( "Array before insertion : " , end = " " )
for i in range ( 0 , 3 ):
print (b[i], end = " " )
print ()
# adding an element using append()
b.append( 4.4 )
print ( "Array after insertion : " , end = " " )
for i in (b):
print (i, end = " " )
print ()
输出:
Array before insertion : 1 2 3
Array after insertion : 1 4 2 3
Array before insertion : 2.5 3.2 3.3
Array after insertion : 2.5 3.2 3.3 4.4
从数组访问元素
为了访问数组项, 请参考索引号。使用索引运算符[]访问数组中的项目。索引必须是整数。
# Python program to demonstrate
# accessing of element from list
# importing array module
import array as arr
# array with int type
a = arr.array( 'i' , [ 1 , 2 , 3 , 4 , 5 , 6 ])
# accessing element of array
print ( "Access element is: " , a[ 0 ])
# accessing element of array
print ( "Access element is: " , a[ 3 ])
# array with float type
b = arr.array( 'd' , [ 2.5 , 3.2 , 3.3 ])
# accessing element of array
print ( "Access element is: " , b[ 1 ])
# accessing element of array
print ( "Access element is: " , b[ 2 ])
输出:
Access element is: 1
Access element is: 4
Access element is: 3.2
Access element is: 3.3
从阵列中删除元素
可以使用内置从数组中删除元素去掉()函数, 但如果集合中不存在元素, 则会发生错误。去掉()方法一次只删除一个元素, 要删除元素范围, 则使用迭代器。pop()函数也可以用于从数组中删除并返回一个元素, 但是默认情况下, 它仅删除数组中的最后一个元素, 以从数组的特定位置删除元素, 该元素的索引作为参数传递给pop()方法。
注意 -List中的Remove方法将仅删除搜索到的元素的第一个匹配项。
# Python program to demonstrate
# Removal of elements in a Array
# importing "array" for array operations
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array( 'i' , [ 1 , 2 , 3 , 1 , 5 ])
# printing original array
print ( "The new created array is : " , end = "")
for i in range ( 0 , 5 ):
print (arr[i], end = " " )
print ( "\r" )
# using pop() to remove element at 2nd position
print ( "The popped element is : " , end = "")
print (arr.pop( 2 ))
# printing array after popping
print ( "The array after popping is : " , end = "")
for i in range ( 0 , 4 ):
print (arr[i], end = " " )
print ( "\r" )
# using remove() to remove 1st occurrence of 1
arr.remove( 1 )
# printing array after removing
print ( "The array after removing is : " , end = "")
for i in range ( 0 , 3 ):
print (arr[i], end = " " )
输出如下:
The new created array is : 1 2 3 1 5
The popped element is : 3
The array after popping is : 1 2 1 5
The array after removing is : 2 1 5
数组切片
在Python数组中, 有多种方法可以打印包含所有元素的整个数组, 但是要从数组中打印特定范围的元素, 我们使用
切片操作
。使用冒号(:)对数组执行切片操作。要从开始到范围内打印元素, 请使用[:Index], 要从最终位置使用[:-Index]打印元素, 要从特定索引开始打印直到最后, 使用[Index:], 要打印范围内的元素, 请使用[开始索引:结束索引], 并使用切片操作打印整个列表, 请使用[:]。此外, 要以相反的顺序打印整个数组, 请使用[::-1]。
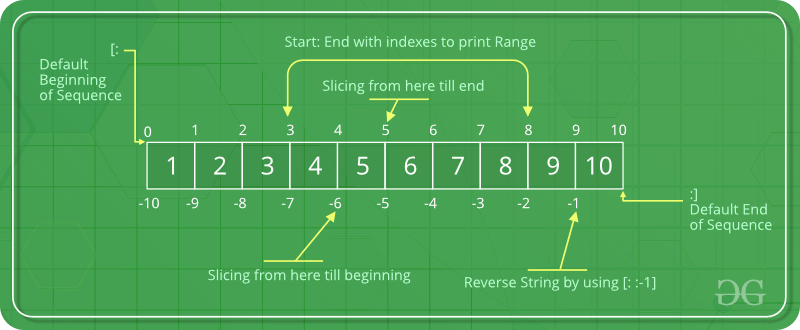
# Python program to demonstrate
# silicing of elements in a Array
# importing array module
import array as arr
# creating a list
l = [ 1 , 2 , 3 , 4 , 5 , 6 , 7 , 8 , 9 , 10 ]
a = arr.array( 'i' , l)
print ( "Intial Array: " )
for i in (a):
print (i, end = " " )
# Print elements of a range
# using Slice operation
Sliced_array = a[ 3 : 8 ]
print ( "\nSlicing elements in a range 3-8: " )
print (Sliced_array)
# Print elements from a
# pre-defined point to end
Sliced_array = a[ 5 :]
print ( "\nElements sliced from 5th "
"element till the end: " )
print (Sliced_array)
# Printing elements from
# beginning till end
Sliced_array = a[:]
print ( "\nPrinting all elements using slice operation: " )
print (Sliced_array)
输出:
Intial Array:
1 2 3 4 5 6 7 8 9 10
Slicing elements in a range 3-8:
array('i', [4, 5, 6, 7, 8])
Elements sliced from 5th element till the end:
array('i', [6, 7, 8, 9, 10])
Printing all elements using slice operation:
array('i', [1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
在数组中搜索元素
为了搜索数组中的元素, 我们使用内置的python指数()方法。此函数返回参数中提到的第一次出现的值的索引。
# Python code to demonstrate
# searching an element in array
# importing array module
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array( 'i' , [ 1 , 2 , 3 , 1 , 2 , 5 ])
# printing original array
print ( "The new created array is : " , end = "")
for i in range ( 0 , 6 ):
print (arr[i], end = " " )
print ( "\r" )
# using index() to print index of 1st occurrenece of 2
print ( "The index of 1st occurrence of 2 is : " , end = "")
print (arr.index( 2 ))
# using index() to print index of 1st occurrenece of 1
print ( "The index of 1st occurrence of 1 is : " , end = "")
print (arr.index( 1 ))
输出如下:
The new created array is : 1 2 3 1 2 5
The index of 1st occurrence of 2 is : 1
The index of 1st occurrence of 1 is : 0
更新数组中的元素
为了更新数组中的元素, 我们只需将新值重新分配给想要更新的所需索引即可。
# Python code to demonstrate
# how to update an element in array
# importing array module
import array
# initializing array with array values
# initializes array with signed integers
arr = array.array( 'i' , [ 1 , 2 , 3 , 1 , 2 , 5 ])
# printing original array
print ( "Array before updation : " , end = "")
for i in range ( 0 , 6 ):
print (arr[i], end = " " )
print ( "\r" )
# updating a element in a array
arr[ 2 ] = 6
print ( "Array after updation : " , end = "")
for i in range ( 0 , 6 ):
print (arr[i], end = " " )
print ()
# updating a element in a array
arr[ 4 ] = 8
print ( "Array after updation : " , end = "")
for i in range ( 0 , 6 ):
print (arr[i], end = " " )
输出如下:
Array before updation : 1 2 3 1 2 5
Array after updation : 1 2 6 1 2 5
Array after updation : 1 2 6 1 8 5
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。