如何实现Python json格式化输出到文件?PrettyPrint 可用于以易于阅读(Python json美化)的格式显示 JSON。当我们在 Python 中说 PrettyPrinting JSON 时,我们主要考虑缩进、键值分隔符和空格,下面详细介绍Python pretty json保存到文件及其相关内容。
我们需要在 Python 中使用 JSON PrettyPrinting 的场景:
- Python json格式化输出到文件:将 PrettyPrinted JSON 格式的数据写入文件
- Prettyprint JSON 文件和Python json美化:假设您有一个很大且缩进不正确的 JSON 文件,并且您想对其进行漂亮打印
- Prettyprint JSON 格式化的字符串,以及Python json格式化保存到文件。
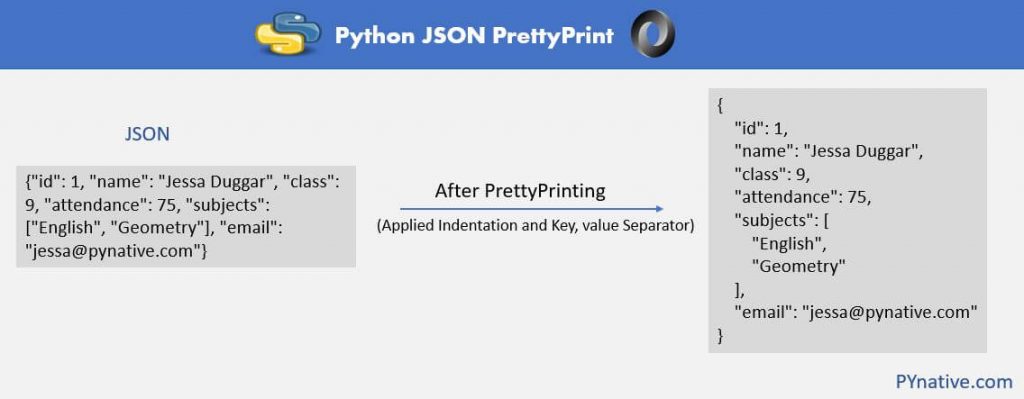
Python json格式化输出到文件:带缩进的pretty json
在现实世界中,我们处理大量 JSON 数据。当我们将 JSON 数据写入文件时,我们必须确保 JSON 数据可读且组织良好,以便使用它的人更好地了解 JSON 的结构。
Python pretty json保存到文件:使用Python json 模块的json.dump()方法,我们可以将漂亮打印的 JSON 写入文件。该json.dump()
方法提供以下参数来漂亮地打印 JSON 数据。
该缩进参数指定在一行的开头使用的空间。我们可以使用 json.dump() 的 indent 参数来指定缩进值。默认情况下,当您将 JSON 数据写入文件时,Python 不使用缩进并将所有数据写入一行,这是不可读的。
该分离器参数:可以指定JSON键和值之间的任何分隔符。默认分隔符是(', ', ': ')
. 例如,如果您指定分隔符,例如(', ', ' : ')
,Python json美化实例:
{
“key1”:“value2”,
“key2”:“value2”
}
sort_keys
按字母顺序对 JSON 键进行排序的 参数。
现在,让我们看看这个Python json格式化输出到文件的Python json美化例子。
import json
print("Writing Indented and Pretty-printed JSON formatted data into a file")
student = {
"id": 1,
"name": "Jessa Duggar",
"class": 9,
"attendance": 75,
"subjects": ["English", "Geometry"],
"email": "jessa@pynative.com"
}
with open("studentWithoutPrettyPrint.json", "w") as write_file:
json.dump(student, write_file)
print("Done writing JSON data into file without Pretty Printing it")
with open("studentWithPrettyPrint1.json", "w") as write_file:
json.dump(student, write_file, indent=4)
print("Done writing PrettyPrinted JSON data into file with indent=4")
with open("studentWithPrettyPrint2.json", "w") as write_file:
json.dump(student, write_file, indent=0)
print("Done writing PrettyPrinted JSON data into file with indent=0")
with open("studentWithPrettyPrint3.json", "w") as write_file:
json.dump(student, write_file, indent=4, sort_keys=True)
print("Done writing Sorted and PrettyPrinted JSON data into file")
输出:
Writing Indented and Pretty-printed JSON formatted data into a file
Done writing JSON data into file without Pretty Printing it
Done writing PrettyPrinted JSON data into file with indent=4
Done writing PrettyPrinted JSON data into file with indent=0
Done writing Sorted and PrettyPrinted JSON data into file
没有Python json美化的例子:
{"id": 1, "name": "Jessa Duggar", "class": 9, "attendance": 75, "subjects": ["English", "Geometry"], "email": "jessa@pynative.com"}
将 JSON 数据漂亮地打印到indent=0的文件中
{
"id": 1,
"name": "Jessa Duggar",
"class": 9,
"attendance": 75,
"subjects": [
"English",
"Geometry"
],
"email": "jessa@pynative.com"
}
将 JSON 数据漂亮地打印到indent=4的文件中
{
"id": 1,
"name": "Jessa Duggar",
"class": 9,
"attendance": 75,
"subjects": [
"English",
"Geometry"
],
"email": "jessa@pynative.com"
}
将 Pretty-Printed JSON 数据写入具有indent=4和排序键的文件后。
{
"attendance": 75,
"class": 9,
"email": "jessa@pynative.com",
"id": 1,
"name": "Jessa Duggar",
"subjects": [
"English",
"Geometry"
]
}
Python json美化注意:
如果缩进是非负整数或字符串,那么 JSON 数组元素和对象成员将使用该缩进级别进行漂亮打印。例如,当我们指定 indent=4 时,Python 使用四个空格进行缩进。
缩进级别 0、负数或""
仅插入换行符。无(默认)选择最紧凑的表示。使用正整数缩进每级缩进多少个空格。如果 indent 是一个字符串(例如\t
),则该字符串用于缩进每个级别。
Python 读取和 PrettyPrint JSON 文件
假设您有一个未正确缩进的大型 JSON 文件,并且您想对其进行漂亮打印。您可以使用两种方式在 Python 中显示漂亮打印的 JSON。让我们一一看看。
在这个例子中,我们使用存储在我电脑上的“ student.json ”文件。该文件包含以下数据。
{"id": 1, "name": "Jessa Duggar", "class": 9, "attendance": 75, "subjects": ["English", "Geometry", "Physics", "World History"], "email": "jess@example.com"}
我们需要遵循以下Python json格式化输出到文件步骤:
- 首先使用json.load() 方法读取 JSON 文件。
- 使用json.dumps() 方法通过指定缩进和分隔符来正确打印 JSON。该
json.dumps()
方法以字符串格式返回漂亮打印的 JSON 数据。 - 打印最终的 JSON
现在让我们看看这个Python json格式化输出到文件例子。
import json
with open("student.json", "r") as read_file:
print("Read JSON file")
student = json.load(read_file)
print("Before Pretty Printing JSON Data")
print(student)
print("\n")
PrettyJson = json.dumps(student, indent=4, separators=(',', ': '), sort_keys=True)
print("Displaying Pretty Printed JSON Data")
print(PrettyJson)
Python json美化输出:
读取 JSON 文件
在漂亮地打印 JSON 数据之前
{'id': 1, 'name': 'Jessa Duggar', 'class': 9, 'attendance': 75, 'subjects': ['English', 'Geometry', 'Physics', 'World History'], 'email': 'jess@example.com'}
显示漂亮的打印 JSON 数据
{
"attendance": 75,
"class": 9,
"email": "jess@example.com",
"id": 1,
"name": "Jessa Duggar",
"subjects": [
"English",
"Geometry",
"Physics",
"World History"
]
}
使用 pprint 模块漂亮地打印 JSON
该pprint
模块提供了“漂亮打印”任何 Python 数据结构的能力。现在,让我们看看如何使用该pprint
模块来漂亮地打印 JSON 数据。
该pprint.pprint()
函数在配置的流上打印 JSON 的格式化表示,后跟换行符,Python json格式化保存到文件步骤:
- 首先通过配置缩进和宽度值构造一个 PrettyPrinter 实例。另外,设置
compact=False
. - 使用
json.load()
方法读取 JSON 文件。 - 将 JSON 数据传递给
pprint.pprint()
函数
现在,让我们看Python json格式化输出到文件演示。
import json
import pprint
with open("studentWithoutPrettyPrint.json", "r") as read_file:
print("Read JSON file")
student = json.load(read_file)
print("Before Pretty Printing JSON Data")
print(student)
print("\n")
# construct PrettyPrinter first
pp = pprint.PrettyPrinter(indent=4, width=80, compact=False)
print("Pretty Printing JSON Data using pprint module")
pp.pprint(student)
输出:
读取 JSON 文件
在漂亮地打印 JSON 数据之前
{'id': 1, 'name': 'Jessa Duggar', 'class': 9, 'attendance': 75, 'subjects': ['English', 'Geometry', 'Physics', 'World History'], 'email': 'jess@example.com'}
使用 pprint 模块漂亮地打印 JSON 数据后
{ 'attendance': 75,
'class': 9,
'email': 'jess@example.com',
'id': 1,
'name': 'Jessa Duggar',
'subjects': ['English', 'Geometry', 'Physics', 'World History']}
注意:使用 print 仅在控制台上显示 JSON 数据。切勿使用其输出将 JSON 写入文件。
从命令行打印pretty JSON
Python 提供了json.tool模块来从命令行验证和漂亮地打印 JSON 对象。我们可以使用其模块的命令行界面执行以下任务。
在 sys.stdout 上将 JSON 字符串显示为漂亮打印的 JSON
在命令行上运行以下命令。在这里,我们正在验证 Python 字典并将其漂亮地打印为 JSON 格式的字符串。
echo {"id": 1, "name": "Jessa Duggar"} | python -m json.tool
输出:
{
"id": 1,
"name": "Jessa Duggar"
}
Python json格式化保存到文件:我们还可以使用json.tool
模块的不同命令行选项来进行 Prettyprint 和验证 JSON。让我们看看那些。
传递 JSON 文件名,在 sys.stdoutth 上漂亮地打印
该json.tool
模块提供了一个infile
命令行选项来指定要漂亮打印的 JSON 文件名。
运行以下命令之前的文件内容,Python json格式化输出到文件:
{"id": 1, "name": "Jessa Duggar", "class": 9, "attendance": 75, "subjects": ["English", "Geometry", "Physics", "World History"], "email": "jess@example.com"}
python -m json.tool studentWithoutPrettyPrint.json
输出:
{
"id": 1,
"name": "Jessa Duggar",
"class": 9,
"attendance": 75,
"subjects": [
"English",
"Geometry",
"Physics",
"World History"
],
"email": "jess@example.com"
}
Python pretty json保存到文件:正如您在命令输出中看到的,文件内容打印得很漂亮。
传递 JSON 文件,PrettyPrint 并将其写入另一个文件
该json.tool
模块还提供了一个outfile
命令行选项,用于将经过验证且打印精美的 JSON 数据写入新文件。
Python json格式化输出到文件命令:
python -m json.tool studentWithoutPrettyPrint.json newFile.json
Python json格式化保存到文件结果:
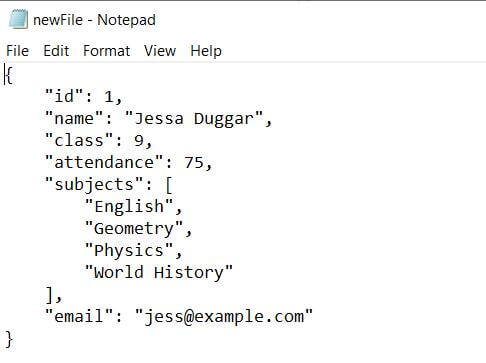
Python pretty json保存到文件:创建了一个新文件,其中包含漂亮打印的 JSON 数据。
Python json格式化输出到文件总结
如何实现Python json格式化保存到文件?在这里,我在 Python 中使用PrettyPrint JSON 数据处理。无论哪种方式,请在下面发表评论让我知道或分享Python json美化的其它方式。