在多重继承中, 一个类可以具有多个父类, 并且可以从其所有父类继承功能。如下图所示, 类C继承了类A和B的功能。
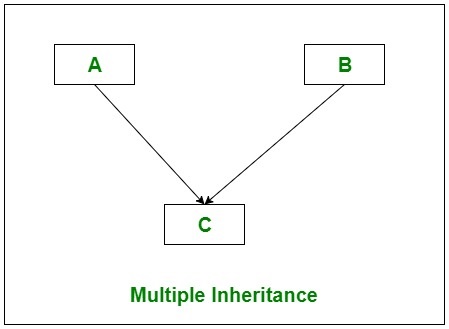
但是C#不支持多类继承。为了克服这个问题, 我们使用接口来实现多个类的继承。借助接口, 类C(如上图所示)可以获得类A和B的功能。
示例1:首先, 我们尝试继承极客1和极客2类别转换为lsbin类别, 则编译器将给出错误信息, 因为C#直接不支持多个类继承。
//C# program to illustrate
//multiple class inheritance
using System;
using System.Collections;
//Parent class 1
class Geeks1 {
//Providing the implementation
//of languages() method
public void languages()
{
//Creating ArrayList
ArrayList My_list = new ArrayList();
//Adding elements in the
//My_list ArrayList
My_list.Add( "C" );
My_list.Add( "C++" );
My_list.Add( "C#" );
My_list.Add( "Java" );
Console.WriteLine( "Languages provided by lsbin:" );
foreach ( var elements in My_list)
{
Console.WriteLine(elements);
}
}
}
//Parent class 2
class Geeks2 {
//Providing the implementation
//of courses() method
public void courses()
{
//Creating ArrayList
ArrayList My_list = new ArrayList();
//Adding elements in the
//My_list ArrayList
My_list.Add( "System Design" );
My_list.Add( "Fork Python" );
My_list.Add( "Geeks Classes DSA" );
My_list.Add( "Fork Java" );
Console.WriteLine( "\nCourses provided by lsbin:" );
foreach ( var elements in My_list)
{
Console.WriteLine(elements);
}
}
}
//Child class
class lsbin : Geeks1, Geeks2 {
}
public class GFG {
//Main method
static public void Main()
{
//Creating object of lsbin class
lsbin obj = new lsbin();
obj.languages();
obj.courses();
}
}
运行时错误:
prog.cs(61, 30):错误CS1721:'lsbin':类不能具有多个基类('Geeks1'和'Geeks2')prog.cs(35, 7):(与先前错误相关的符号位置)
但是我们可以使用接口将Geeks1和Geek2类的功能间接继承到lsbin类中。如下图所示。
示例2:都GFG1和GFG2接口由Geeks1和Geeks2类实现。现在, Geeks1和Geeks2类定义了language()和courses()方法。当lsbin类继承GFG1和GFG2接口时, 你无需重新定义语言()和培训班()方法只是简单地创建对象极客1和极客2上课并访问语言()和培训班()lsbin类中使用这些对象的方法。
//C# program to illustrate how to
//implement multiple class inheritance
//using interfaces
using System;
using System.Collections;
//Interface 1
interface GFG1 {
void languages();
}
//Parent class 1
class Geeks1 : GFG1 {
//Providing the implementation
//of languages() method
public void languages()
{
//Creating ArrayList
ArrayList My_list = new ArrayList();
//Adding elements in the
//My_list ArrayList
My_list.Add( "C" );
My_list.Add( "C++" );
My_list.Add( "C#" );
My_list.Add( "Java" );
Console.WriteLine( "Languages provided by lsbin:" );
foreach ( var elements in My_list)
{
Console.WriteLine(elements);
}
}
}
//Interface 2
interface GFG2 {
void courses();
}
//Parent class 2
class Geeks2 : GFG2 {
//Providing the implementation
//of courses() method
public void courses()
{
//Creating ArrayList
ArrayList My_list = new ArrayList();
//Adding elements in the
//My_list ArrayList
My_list.Add( "System Design" );
My_list.Add( "Fork Python" );
My_list.Add( "Geeks Classes DSA" );
My_list.Add( "Fork Java" );
Console.WriteLine( "\nCourses provided by lsbin:" );
foreach ( var elements in My_list)
{
Console.WriteLine(elements);
}
}
}
//Child class
class lsbin : GFG1, GFG2 {
//Creating objects of Geeks1 and Geeks2 class
Geeks1 obj1 = new Geeks1();
Geeks2 obj2 = new Geeks2();
public void languages()
{
obj1.languages();
}
public void courses()
{
obj2.courses();
}
}
//Driver Class
public class GFG {
//Main method
static public void Main()
{
//Creating object of lsbin class
lsbin obj = new lsbin();
obj.languages();
obj.courses();
}
}
输出如下:
Languages provided by lsbin:
C
C++
C#
Java
Courses provided by lsbin:
System Design
Fork Python
Geeks Classes DSA
Fork Java