多任务处理是指多个任务或进程在一定时间间隔内同时执行。Windows操作系统是一个多任务的例子,因为它能够在一个时间运行多个进程,如运行谷歌Chrome,记事本,VLC播放器等在同一时间。操作系统使用一个称为进程的术语来同时执行所有这些应用程序。进程是操作系统的一部分,负责执行应用程序。在系统上执行的每个程序都是一个进程,要在应用程序内运行代码,进程使用一个术语称为线程。
一个线程是一个轻量级的进程,或者换句话说,一个线程是一个执行程序下的代码的单元。所以每个程序都有逻辑,一个线程负责执行这个逻辑。每个程序默认携带一个线程来执行程序的逻辑,这个线程被称为主线程,所以每个程序或应用程序默认是单线程模型。这种单线程模型有一个缺点。单线程以同步的方式运行程序中存在的所有进程,意味着一个接一个。因此,第二个进程会一直等待,直到第一个进程完成它的执行,它在处理过程中会消耗更多的时间。
例如,我们有一个名为Geek的类,这个类包含两个不同的方法,即method1和method2。现在主线程负责执行所有这些方法,所以主线程一个接一个地执行所有这些方法。
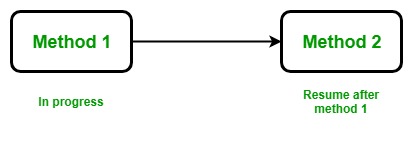
例子:
//C# program to illustrate the
//concept of single threaded model
using System;
using System.Threading;
public class Geek {
//static method one
public static void method1()
{
//It prints numbers from 0 to 10
for ( int I = 0; I <= 10; I++) {
Console.WriteLine( "Method1 is : {0}" , I);
//When the value of I is equal to
//5 then this method sleeps for
//6 seconds and after 6 seconds
//it resumes its working
if (I == 5) {
Thread.Sleep(6000);
}
}
}
//static method two
public static void method2()
{
//It prints numbers from 0 to 10
for ( int J = 0; J <= 10; J++) {
Console.WriteLine( "Method2 is : {0}" , J);
}
}
}
//Driver Class
public class GFG {
//Main Method
static public void Main()
{
//Calling static methods
Geek.method1();
Geek.method2();
}
}
输出如下:
Method1 is : 0
Method1 is : 1
Method1 is : 2
Method1 is : 3
Method1 is : 4
Method1 is : 5
Method1 is : 6
Method1 is : 7
Method1 is : 8
Method1 is : 9
Method1 is : 10
Method2 is : 0
Method2 is : 1
Method2 is : 2
Method2 is : 3
Method2 is : 4
Method2 is : 5
Method2 is : 6
Method2 is : 7
Method2 is : 8
Method2 is : 9
Method2 is : 10
说明:在这里, 首先方法1执行。在方法1, 对于当i的值等于5时, 循环从0开始, 然后该方法进入睡眠状态6秒钟, 并在6秒钟后恢复过程并打印剩余值。直到方法2处于等待状态。方法2何时开始工作方法1完成分配的任务。因此克服了单线程模型的弊端多线程介绍。
多线程是一个在单个进程中包含多个线程的进程。在这里, 每个线程执行不同的活动。例如, 我们有一个类, 此调用包含两个不同的方法, 现在使用多线程, 每个方法由一个单独的线程执行。因此, 多线程的主要优点是可以同时工作, 这意味着多个任务可以同时执行。而且, 由于多线程在分时概念上工作, 因此还可以最大程度地提高CPU的利用率, 这意味着每个线程都有自己的执行时间, 并且不影响另一个线程的执行, 该时间间隔由操作系统决定。
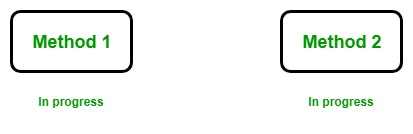
例子:
//C# program to illustrate the
//concept of multithreading
using System;
using System.Threading;
public class GFG {
//static method one
public static void method1()
{
//It prints numbers from 0 to 10
for ( int I = 0; I <= 10; I++) {
Console.WriteLine( "Method1 is : {0}" , I);
//When the value of I is equal to 5 then
//this method sleeps for 6 seconds
if (I == 5) {
Thread.Sleep(6000);
}
}
}
//static method two
public static void method2()
{
//It prints numbers from 0 to 10
for ( int J = 0; J <= 10; J++) {
Console.WriteLine( "Method2 is : {0}" , J);
}
}
//Main Method
static public void Main()
{
//Creating and initializing threads
Thread thr1 = new Thread(method1);
Thread thr2 = new Thread(method2);
thr1.Start();
thr2.Start();
}
}
输出:
Method1 is : 0
Method1 is : 1
Method1 is : 2
Method1 is : 3
Method2 is : 0
Method2 is : 1
Method2 is : 2
Method2 is : 3
Method2 is : 4
Method2 is : 5
Method2 is : 6
Method2 is : 7
Method2 is : 8
Method2 is : 9
Method2 is : 10
Method1 is : 4
Method1 is : 5
Method1 is : 6
Method1 is : 7
Method1 is : 8
Method1 is : 9
Method1 is : 10
说明:在这里, 我们创建并初始化两个线程, 即thr1和thr2使用Thread类。现在使用thr1.Start();和thr2.Start();我们开始执行两个线程。现在, 两个线程同时运行, 并且thr2不依赖于thr1就像在单线程模型中一样。
注意:输出可能因上下文切换而有所不同。
多线程的优点:
- 它同时执行多个过程。
- 最大限度地利用CPU资源。
- 多个进程之间的时间共享。