Django在MVT模式上工作。因此需要创建数据模型(或表)。对于每个表,都会创建一个模型类。
假设有一个表单接受用户的Username、gender和text作为输入,任务是验证数据并保存它。
在django中可以这样做,如下:
from django.db import models
# model named Post
class Post(models.Model):
Male = 'M'
FeMale = 'F'
GENDER_CHOICES = (
(Male, 'Male' ), (FeMale, 'Female' ), )
# define a username filed with bound max length it can have
username = models.CharField( max_length = 20 , blank = False , null = False )
# This is used to write a post
text = models.TextField(blank = False , null = False )
# Values for gender are restricted by giving choices
gender = models.CharField(max_length = 6 , choices = GENDER_CHOICES, default = Male)
time = models.DateTimeField(auto_now_add = True )
创建数据模型后, 需要将更改反映到数据库中, 以执行以下命令:
python manange.py makemigrations
这样做会编译模型, 如果没有发现任何错误, 则会在迁移文件夹中创建一个文件。稍后运行下面给出的命令以最终将保存到迁移文件上的更改反映到数据库中。
python manage.py migrate
现在可以创建一个表格。假设用户名长度不应小于5, 而帖子长度应大于10。然后定义类邮寄表格具有所需的验证规则, 如下所示:
from django.forms import ModelForm
from django import forms
from formValidationApp.models import *
# define the class of a form
class PostForm(ModelForm):
class Meta:
# write the name of models for which the form is made
model = Post
# Custom fields
fields = [ "username" , "gender" , "text" ]
# this function will be used for the validation
def clean( self ):
# data from the form is fetched using super function
super (PostForm, self ).clean()
# extract the username and text field from the data
username = self .cleaned_data.get( 'username' )
text = self .cleaned_data.get( 'text' )
# conditions to be met for the username length
if len (username) <5 :
self ._errors[ 'username' ] = self .error_class([
'Minimum 5 characters required' ])
if len (text) <10 :
self ._errors[ 'text' ] = self .error_class([
'Post Should Contain a minimum of 10 characters' ])
# return any errors if found
return self .cleaned_data
到目前为止, 已经定义了数据模型和Form类。现在, 重点将放在上面定义的这些模块的实际使用上。
首先, 通过此命令在本地主机上运行
python manage.py runserver
打开http://localhost:8000/在浏览器中, 然后它将在urls.py文件, 查找""路径
urls.py文件如下所示:
from django.contrib import admin
from django.urls import path, include
from django.conf.urls import url
from django.shortcuts import HttpResponse
from . import views
urlpatterns = [
path(' ', views.home, name =' index'), ]
基本上, 这会将‘’网址与一个函数相关联家在中定义views.py文件。
views.py文件:
from .models import Post
from .forms import PostForm
from . import views
from django.shortcuts import HttpResponse, render, redirect
def home(request):
# check if the request is post
if request.method = = 'POST' :
# Pass the form data to the form class
details = PostForm(request.POST)
# In the 'form' class the clean function
# is defined, if all the data is correct
# as per the clean function, it returns true
if details.is_valid():
# Temporarily make an object to be add some
# logic into the data if there is such a need
# before writing to the database
post = details.save(commit = False )
# Finally write the changes into database
post.save()
# redirect it to some another page indicating data
# was inserted successfully
return HttpResponse( "data submitted successfully" )
else :
# Redirect back to the same page if the data
# was invalid
return render(request, "home.html" , { 'form' :details})
else :
# If the request is a GET request then, # create an empty form object and
# render it into the page
form = PostForm( None )
return render(request, 'home.html' , { 'form' :form})
home.html模板文件
{% load bootstrap3 %}
{% bootstrap_messages %}
<!DOCTYPE html>
<html lang = "en">
<head>
<title>Basic Form</title>
<meta charset = "utf-8" />
<meta name = "viewport" content = "width=device-width, initial-scale=1, shrink-to-fit=no">
<link rel = "stylesheet" href = "https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css">
<script src = "https://ajax.googleapis.com/ajax/libs/jquery/3.2.1/jquery.min.js">
</script>
<script src = "https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js">
</script>
</head>
<body style = "padding-top: 60px;background-color: #f5f7f8 !important;">
<div class = "container">
<div class = "row">
<div class = "col-md-4 col-md-offset-4">
<h2>Form</h2>
<form action = "" method = "post"><input type = 'hidden' />
{%csrf_token %}
{% bootstrap_form form %}
<!-This is the form variable which we are passing from the function
of home in views.py file. That's the beauty of Django we
don't need to write much codes in this it'll automatically pass
all the form details in here
->
<div class = "form-group">
<button type = "submit" class = "btn btn-default ">
Submit
</button>
</div>
</form>
</div>
</div>
</div>
</body>
</html>
开场http://localhost:8000/在浏览器中显示以下内容,
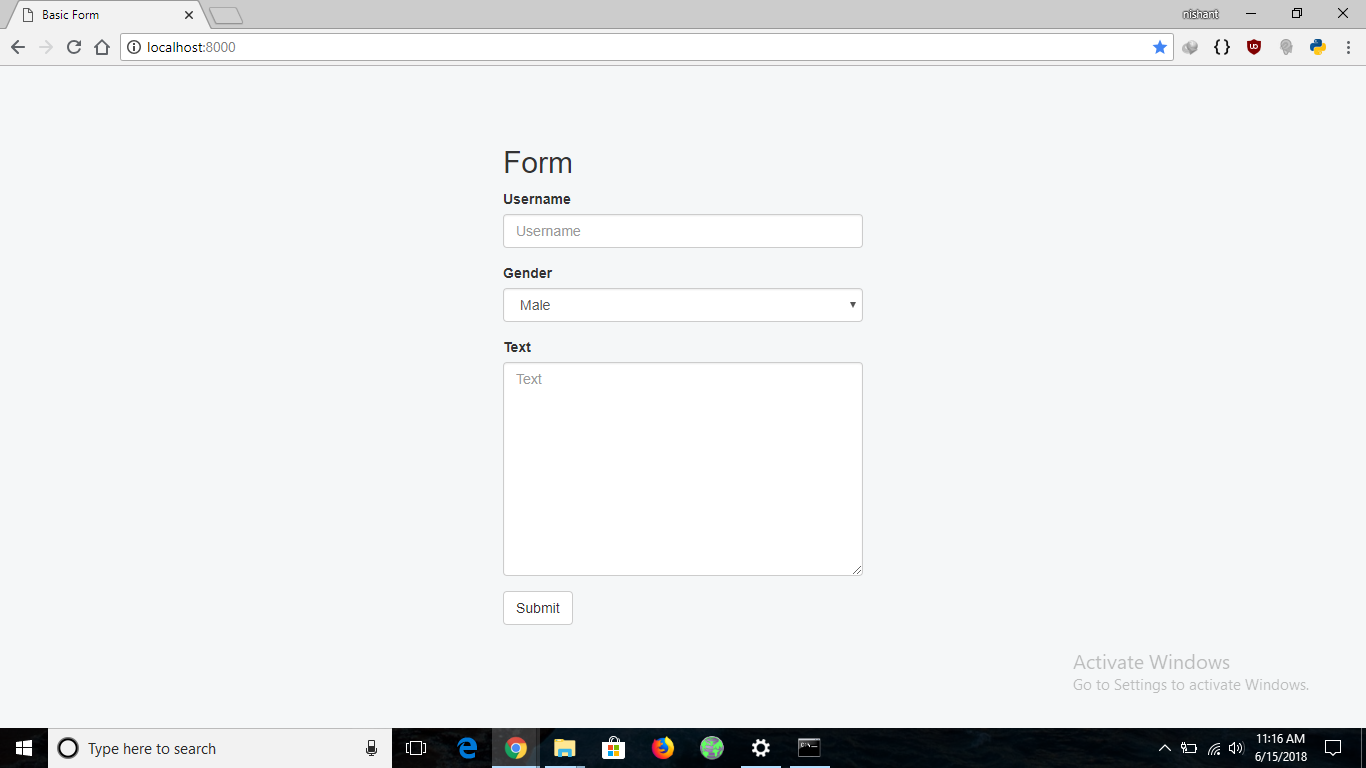
如果提交的用户名长度小于5的表单, 则在提交时会出现错误, 并且填写的"张贴文字"也会类似。下图显示了提交无效表单数据时表单的行为。
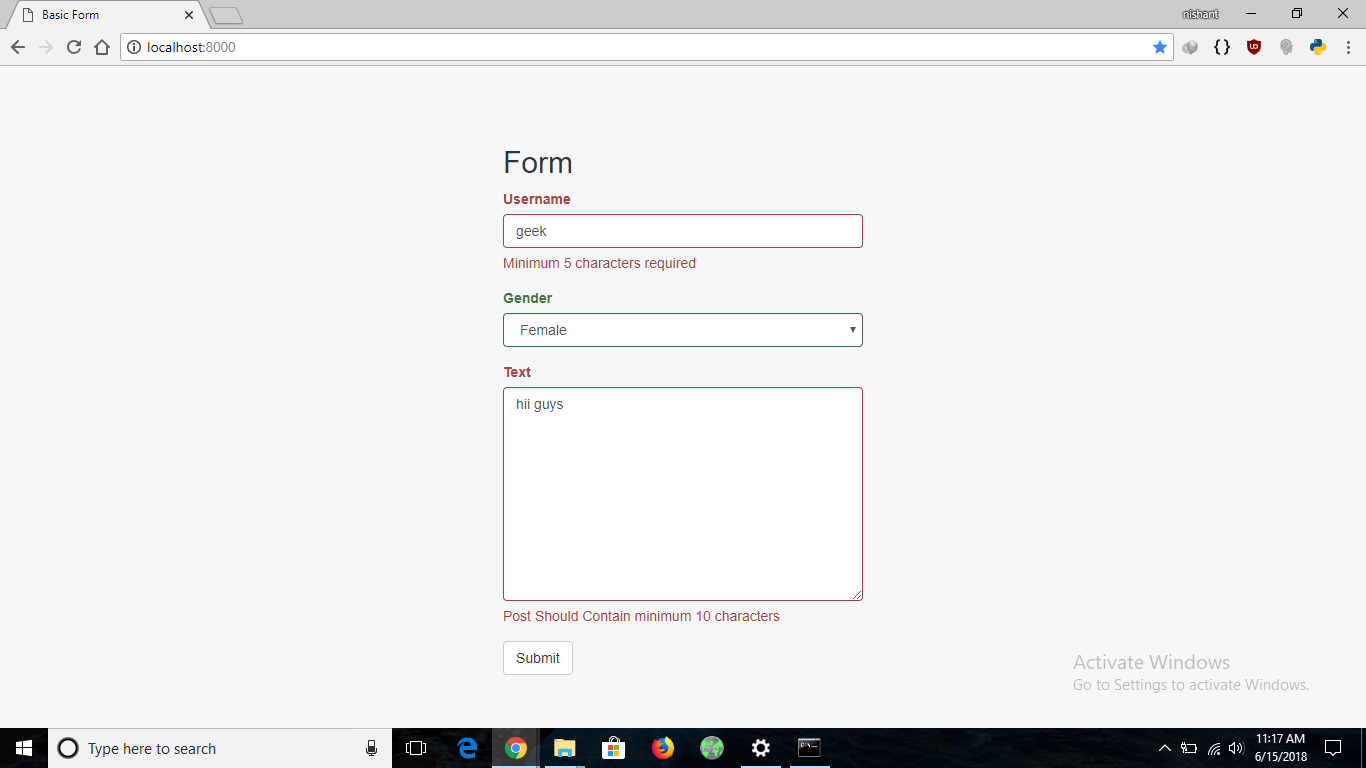
参考文献:https://docs.djangoproject.com/en/2.1/ref/forms/validation/
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。