让我们讨论一下如何向Pandas中的现有DataFrame添加新列。我们可以通过多种方式完成此任务。
方法1:通过将新列表声明为一列。
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = { 'Name' : [ 'Jai' , 'Princi' , 'Gaurav' , 'Anuj' ], 'Height' : [ 5.1 , 6.2 , 5.1 , 5.2 ], 'Qualification' : [ 'Msc' , 'MA' , 'Msc' , 'Msc' ]}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Declare a list that is to be converted into a column
address = [ 'Delhi' , 'Bangalore' , 'Chennai' , 'Patna' ]
# Using 'Address' as the column name
# and equating it to the list
df[ 'Address' ] = address
# Observe the result
df
输出如下:
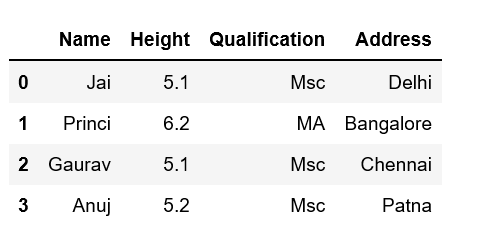
请注意, 列表的长度应与索引列的长度匹配, 否则将显示错误。
方法2:通过使用DataFrame.insert() 它使你可以自由地在我们喜欢的任何位置(而不仅仅是在结尾处)添加一列。它还提供了用于插入列值的不同选项。
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = { 'Name' : [ 'Jai' , 'Princi' , 'Gaurav' , 'Anuj' ], 'Height' : [ 5.1 , 6.2 , 5.1 , 5.2 ], 'Qualification' : [ 'Msc' , 'MA' , 'Msc' , 'Msc' ]}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Using DataFrame.insert() to add a column
df.insert( 2 , "Age" , [ 21 , 23 , 24 , 21 ], True )
# Observe the result
df
输出如下:
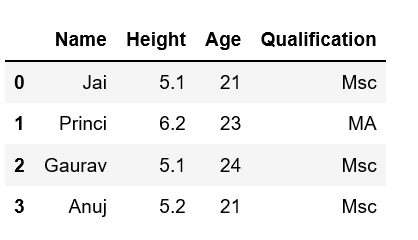
方法3:使用Dataframe.assign()方法
此方法将创建一个新数据框, 并将新列添加到旧数据框。
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = { 'Name' : [ 'Jai' , 'Princi' , 'Gaurav' , 'Anuj' ], 'Height' : [ 5.1 , 6.2 , 5.1 , 5.2 ], 'Qualification' : [ 'Msc' , 'MA' , 'Msc' , 'Msc' ]}
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Using 'Address' as the column name and equating it to the list
df2 = df.assign(address = [ 'Delhi' , 'Bangalore' , 'Chennai' , 'Patna' ])
# Observe the result
df2
输出如下:
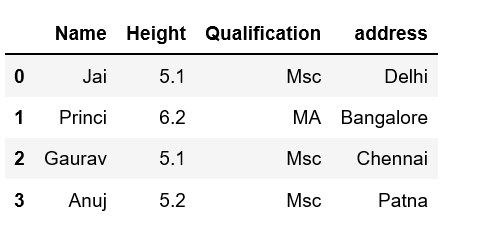
方法4:通过使用字典
我们可以使用Python字典在pandas DataFrame中添加新列。使用现有列作为键值, 它们各自的值将是新列的值。
# Import pandas package
import pandas as pd
# Define a dictionary containing Students data
data = { 'Name' : [ 'Jai' , 'Princi' , 'Gaurav' , 'Anuj' ], 'Height' : [ 5.1 , 6.2 , 5.1 , 5.2 ], 'Qualification' : [ 'Msc' , 'MA' , 'Msc' , 'Msc' ]}
# Define a dictionary with key values of
# an existing column and their respective
# value pairs as the # values for our new column.
address = { 'Delhi' : 'Jai' , 'Bangalore' : 'Princi' , 'Patna' : 'Gaurav' , 'Chennai' : 'Anuj' }
# Convert the dictionary into DataFrame
df = pd.DataFrame(data)
# Provide 'Address' as the column name
df[ 'Address' ] = address
# Observe the output
df
输出如下:
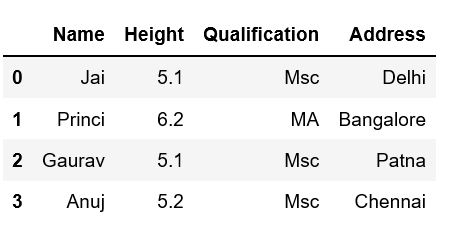
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。