本文概述
使用xlrd模块, 可以从电子表格中检索信息。例如, 可以在Python中完成读取, 写入或修改数据的操作。此外, 用户可能必须浏览各种工作表并根据某些条件检索数据或修改某些行和列并进行大量工作。
rd模块用于从电子表格中提取数据。
安装xlrd模块的命令:
pip install xlrd
输入文件:
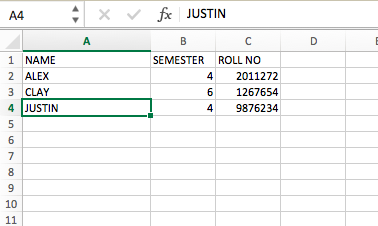
代码1:提取特定的单元格
Python3
# Reading an excel file using Python
import xlrd
# Give the location of the file
loc = ( "path of file" )
# To open Workbook
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
print (sheet.cell_value( 0 , 0 ))
输出:
'NAME'
代码2:提取行数
Python3
# Program to extract number
# of rows using Python
import xlrd
# Give the location of the file
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
# Extracting number of rows
print (sheet.nrows)
输出:
4
代码3:提取列数
Python3
# Program to extract number of
# columns in Python
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
sheet.cell_value( 0 , 0 )
# Extracting number of columns
print (sheet.ncols)
输出:
3
代码4:提取所有列名称
Python3
# Program extracting all columns
# name in Python
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
sheet.cell_value( 0 , 0 )
for i in range (sheet.ncols):
print (sheet.cell_value( 0 , i))
输出:
NAME
SEMESTER
ROLL NO
代码5:提取第一列
Python3
# Program extracting first column
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
for i in range (sheet.nrows):
print (sheet.cell_value(i, 0 ))
输出:
NAME
ALEX
CLAY
JUSTIN
代码6:提取特定的行值
Python3
# Program to extract a particular row value
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
print (sheet.row_values( 1 ))
输出:
['ALEX', 4.0, 2011272.0]
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。