本文概述
- C++
- C
- Java
- Python 3
- C#
- PHP
- C++
- C
- Java
- Python3
- C#
- PHP
- C++
- C
- Java
- Python3
- C#
- PHP
- C++
- C
- Java
- Python3
- PHP
- C++
- C
- Java
- Python3
- C#
- PHP
- C++
给定x和y这两个变量, 不使用第三个变量就交换两个变量。
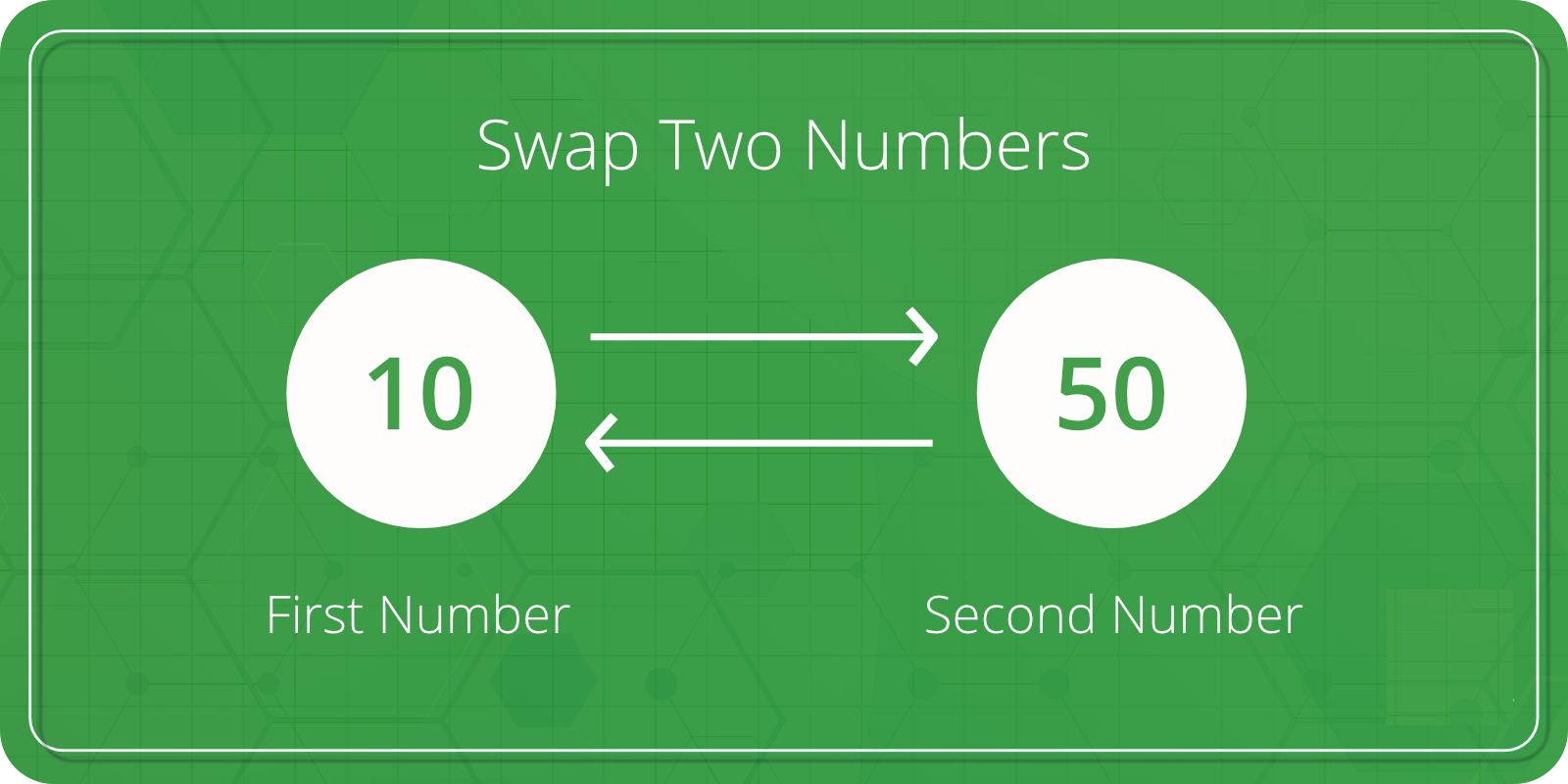
方法1(使用算术运算符)
这个想法是获得两个给定数字之一的和。然后可以使用总和与总和相减来交换数字。
C++
//C++ Program to swap two numbers without
//using temporary variable
#include <bits/stdc++.h>
using namespace std;
int main()
{
int x = 10, y = 5;
//Code to swap 'x' and 'y'
x = x + y; //x now becomes 15
y = x - y; //y becomes 10
x = x - y; //x becomes 5
cout <<"After Swapping: x =" <<x <<", y=" <<y;
}
//This code is contributed by mohit kumar.
C
#include <stdio.h>
int main()
{
int x = 10, y = 5;
//Code to swap 'x' and 'y'
x = x + y; //x now becomes 15
y = x - y; //y becomes 10
x = x - y; //x becomes 5
printf ( "After Swapping: x = %d, y = %d" , x, y);
return 0;
}
Java
//Java Program to swap two numbers without
//using temporary variable
import java.*;
class Geeks {
public static void main(String a[])
{
int x = 10 ;
int y = 5 ;
x = x + y;
y = x - y;
x = x - y;
System.out.println( "After swaping:"
+ " x = " + x + ", y = " + y);
}
}
//This code is contributed by Sam007
Python 3
x = 10
y = 5
# Code to swap 'x' and 'y'
# x now becomes 15
x = x + y
# y becomes 10
y = x - y
# x becomes 5
x = x - y
print ( "After Swapping: x =" , x, " y =" , y);
# This code is contributed
# by Sumit Sudhakar
C#
//Program to swap two numbers without
//using temporary variable
using System;
class GFG {
public static void Main()
{
int x = 10;
int y = 5;
x = x + y;
y = x - y;
x = x - y;
Console.WriteLine( "After swapping: x = "
+ x + ", y = " + y);
}
}
//This code is contributed by Sam007
PHP
<?php
//PHP Program to swap two
//numbers without using
//temporary variable
$x = 10; $y = 5;
//Code to swap 'x' and 'y'
$x = $x + $y ; //x now becomes 15
$y = $x - $y ; //y becomes 10
$x = $x - $y ; //x becomes 5
echo "After Swapping: x = " , $x , ", " , "y = " , $y ;
//This code is contributed by m_kit
?>
输出:
After Swapping: x = 5, y = 10
乘法和除法也可以用于交换。
C++
//C++ Program to swap two numbers
//without using temporary variable
#include <bits/stdc++.h>
using namespace std;
int main()
{ //NOTE - for this code to work in a generalised sense, y !- 0 to prevent zero division
int x = 10, y = 5;
//Code to swap 'x' and 'y'
x = x * y; //x now becomes 15
y = x /y; //y becomes 10
x = x /y; //x becomes 5
cout <<"After Swapping: x =" <<x <<", y=" <<y;
}
//This code is contributed by mohit kumar.
C
#include <stdio.h>
int main()
{
int x = 10, y = 5;
//Code to swap 'x' and 'y'
x = x * y; //x now becomes 50
y = x /y; //y becomes 10
x = x /y; //x becomes 5
printf ( "After Swapping: x = %d, y = %d" , x, y);
return 0;
}
Java
//Java Program to swap two numbers
//without using temporary variable
import java.io.*;
class GFG {
public static void main(String[] args)
{
int x = 10 ;
int y = 5 ;
//Code to swap 'x' and 'y'
x = x * y; //x now becomes 50
y = x /y; //y becomes 10
x = x /y; //x becomes 5
System.out.println( "After swaping:"
+ " x = " + x + ", y = " + y);
}
}
//This code is contributed by ajit
Python3
# Python3 program to
# swap two numbers
# without using
# temporary variable
x = 10
y = 5
# code to swap
# 'x' and 'y'
# x now becomes 50
x = x * y
# y becomes 10
y = x //y;
# x becomes 5
x = x //y;
print ( "After Swapping: x =" , x, " y =" , y);
# This code is contributed
# by @ajit
C#
//C# Program to swap two
//numbers without using
//temporary variable
using System;
class GFG {
static public void Main()
{
int x = 10;
int y = 5;
//Code to swap 'x' and 'y'
x = x * y; //x now becomes 50
y = x /y; //y becomes 10
x = x /y; //x becomes 5
Console.WriteLine( "After swaping:"
+ " x = " + x + ", y = " + y);
}
}
//This code is contributed by ajit.
PHP
<?php
//Driver code
$x = 10;
$y = 5;
//Code to swap 'x' and 'y'
$x = $x * $y ; //x now becomes 50
$y = $x /$y ; //y becomes 10
$x = $x /$y ; //x becomes 5
echo "After Swapping: x = " , $x , " " , "y = " , $y ;
//This code is contributed by m_kit
?>
输出:
After Swapping: x = 5, y = 10
方法2(使用按位XOR)
按位XOR运算符可用于交换两个变量。两个数字x和y的XOR返回一个数字, 其中x和y的位不同时, 所有位都为1。例如, 10(在Binary 1010中)和5(在Binary 0101中)的XOR为1111, 而7(0111)和5(0101)的XOR为(0010)。
C++
//C++ code to swap using XOR
#include <bits/stdc++.h>
using namespace std;
int main()
{
int x = 10, y = 5;
//Code to swap 'x' (1010) and 'y' (0101)
x = x ^ y; //x now becomes 15 (1111)
y = x ^ y; //y becomes 10 (1010)
x = x ^ y; //x becomes 5 (0101)
cout <<"After Swapping: x =" <<x <<", y=" <<y;
return 0;
}
//This code is contributed by mohit kumar.
C
//C code to swap using XOR
#include <stdio.h>
int main()
{
int x = 10, y = 5;
//Code to swap 'x' (1010) and 'y' (0101)
x = x ^ y; //x now becomes 15 (1111)
y = x ^ y; //y becomes 10 (1010)
x = x ^ y; //x becomes 5 (0101)
printf ( "After Swapping: x = %d, y = %d" , x, y);
return 0;
}
Java
//Java code to swap using XOR
import java.*;
public class GFG {
public static void main(String a[])
{
int x = 10 ;
int y = 5 ;
//Code to swap 'x' (1010) and 'y' (0101)
x = x ^ y; //x now becomes 15 (1111)
y = x ^ y; //y becomes 10 (1010)
x = x ^ y; //x becomes 5 (0101)
System.out.println( "After swap: x = "
+ x + ", y = " + y);
}
}
//This code is contributed by Sam007.
Python3
# Python 3 code to swap using XOR
x = 10
y = 5
# Code to swap 'x' and 'y'
x = x ^ y; # x now becomes 15 (1111)
y = x ^ y; # y becomes 10 (1010)
x = x ^ y; # x becomes 5 (0101)
print ( "After Swapping: x = " , x, " y =" , y)
# This code is contributed by
# Sumit Sudhakar
C#
//C# program to swap using XOR
using System;
class GFG {
public static void Main()
{
int x = 10;
int y = 5;
//Code to swap 'x' (1010)
//and 'y' (0101)
//x now becomes 15 (1111)
x = x ^ y;
//y becomes 10 (1010)
y = x ^ y;
//x becomes 5 (0101)
x = x ^ y;
Console.WriteLine( "After swap: x = " + x + ", y = " + y);
}
}
//This code is contributed by ajit
PHP
<?php
//Driver Code
$x = 10;
$y = 5;
//Code to swap 'x' (1010)
//and 'y' (0101)
//x now becomes 15 (1111)
$x = $x ^ $y ;
//y becomes 10 (1010)
$y = $x ^ $y ;
//x becomes 5 (0101)
$x = $x ^ $y ;
echo "After Swapping: x = " , $x , ", " , "y = " , $y ;
//This code is contributed by aj_36
?>
输出:
After Swapping: x = 5, y = 10
上述方法的问题
1)
如果其中一个数字为0, 则乘积和除法将不起作用, 因为乘积将变为0, 而与另一个数字无关。
2)
两种算术解决方案都可能导致算术溢出。如果x和y太大, 则加法和乘法可能超出整数范围。
3)
当我们使用指向变量的指针并进行函数交换时, 当两个指针都指向同一个变量时, 上述所有方法都会失败。让我们看看如果两种情况都指向同一个变量, 将会发生什么情况。
//基于位异或的方法
x = x ^ x; // x变为0
x = x ^ x; // x保持为0
x = x ^ x; // x保持为0
//基于算术的方法
x = x + x; // x变成2x
x = x – x; // x变为0
x = x – x; // x保持为0
让我们看下面的程序。
C++
#include <bits/stdc++.h>
using namespace std;
void swap( int * xp, int * yp)
{
*xp = *xp ^ *yp;
*yp = *xp ^ *yp;
*xp = *xp ^ *yp;
}
//Driver code
int main()
{
int x = 10;
swap(&x, &x);
cout<<"After swap(&x, &x): x = " <<x;
return 0;
}
//This code is contributed by rathbhupendra
C
#include <stdio.h>
void swap( int * xp, int * yp)
{
*xp = *xp ^ *yp;
*yp = *xp ^ *yp;
*xp = *xp ^ *yp;
}
int main()
{
int x = 10;
swap(&x, &x);
printf ( "After swap(&x, &x): x = %d" , x);
return 0;
}
Java
class GFG
{
static void swap( int [] xp, int [] yp)
{
xp[ 0 ] = xp[ 0 ] ^ yp[ 0 ];
yp[ 0 ] = xp[ 0 ] ^ yp[ 0 ];
xp[ 0 ] = xp[ 0 ] ^ yp[ 0 ];
}
//Driver code
public static void main(String[] args)
{
int [] x = { 10 };
swap(x, x);
System.out.println( "After swap(&x, &x): x = " + x[ 0 ]);
}
}
//This code is contributed by Rajput-Ji
Python3
def swap(xp, yp):
xp[ 0 ] = xp[ 0 ] ^ yp[ 0 ]
yp[ 0 ] = xp[ 0 ] ^ yp[ 0 ]
xp[ 0 ] = xp[ 0 ] ^ yp[ 0 ]
# Driver code
x = [ 10 ]
swap(x, x)
print ( "After swap(&x, &x): x = " , x[ 0 ])
# This code is contributed by SHUBHAMSINGH10
PHP
<?php
function swap(& $xp , & $yp )
{
$xp = $xp ^ $yp ;
$yp = $xp ^ $yp ;
$xp = $xp ^ $yp ;
}
//Driver Code
$x = 10;
swap( $x , $x );
print ( "After swap(&x, &x): x = " . $x );
//This code is contributed
//by chandan_jnu
?>
输出:
After swap(&x, &x): x = 0
在许多标准算法中可能需要将变量本身交换。例如看
这个
实施
快速排序
我们可以在其中交换变量。通过在交换之前设置条件可以避免上述问题。
C++
#include <bits/stdc++.h>
using namespace std;
void swap( int * xp, int * yp)
{
//Check if the two addresses are same
if (xp == yp)
return ;
*xp = *xp + *yp;
*yp = *xp - *yp;
*xp = *xp - *yp;
}
//Driver Code
int main()
{
int x = 10;
swap(&x, &x);
cout <<"After swap(&x, &x): x = " <<x;
return 0;
}
//This code is contributed by rathbhupendra
C
#include <stdio.h>
void swap( int * xp, int * yp)
{
if (xp == yp) //Check if the two addresses are same
return ;
*xp = *xp + *yp;
*yp = *xp - *yp;
*xp = *xp - *yp;
}
int main()
{
int x = 10;
swap(&x, &x);
printf ( "After swap(&x, &x): x = %d" , x);
return 0;
}
Java
//Java program of above approach
class GFG {
static void swap( int xp, int yp)
{
if (xp == yp) //Check if the two addresses are same
return ;
xp = xp + yp;
yp = xp - yp;
xp = xp - yp;
}
//Driver code
public static void main(String[] args)
{
int x = 10 ;
swap(x, x);
System.out.println( "After swap(&x, &x): x = " + x);
}
}
//This code is Contributed by Code_Mech.
Python3
# Python3 program of above approach
def swap(xp, yp):
# Check if the two addresses are same
if (xp[ 0 ] = = yp[ 0 ]):
return
xp[ 0 ] = xp[ 0 ] + yp[ 0 ]
yp[ 0 ] = xp[ 0 ] - yp[ 0 ]
xp[ 0 ] = xp[ 0 ] - yp[ 0 ]
# Driver Code
x = [ 10 ]
swap(x, x)
print ( "After swap(&x, &x): x = " , x[ 0 ])
# This code is contributed by SHUBHAMSINGH10
C#
//C# program of above approach
using System;
class GFG {
static void swap( int xp, int yp)
{
if (xp == yp) //Check if the two addresses are same
return ;
xp = xp + yp;
yp = xp - yp;
xp = xp - yp;
}
//Driver code
public static void Main()
{
int x = 10;
swap(x, x);
Console.WriteLine( "After swap(&x, &x): x = " + x);
}
}
//This code is Contributed by Code_Mech.
PHP
<?php
function swap( $xp , $yp )
{
//Check if the two addresses
//are same
if ( $xp == $yp )
return ;
$xp = $xp + $yp ;
$yp = $xp - $yp ;
$xp = $xp - $yp ;
}
//Driver Code
$x = 10;
swap( $x , $x );
echo ( "After swap(&x, &x): x = " . $x );
return 0;
//This code is contributed
//by Code_Mech.
输出:
After swap(&x, &x): x = 10
方法3(按位运算符和算术运算符的混合)
这个想法与在
方法1
但是使用按位加减法进行交换。
下面是上述方法的实现。
C++
//C++ program to swap two numbers.
//Including header file.
#include<bits/stdc++.h>
using namespace std;
//Function to swap the numbers.
void swap( int &a, int &b)
{
//same as a = a + b
a = (a & b) + (a | b);
//same as b = a - b
b = a ^ b;
//same as a = a - b
a = a + (~b) + 1;
}
//Driver Code
int main()
{
int a = 5, b = 10;
//Function Call
swap(a, b);
cout <<"After swapping: a = " <<a <<
", b = " <<b;
return 0;
}
//This code is contributed by yashbeersingh42
输出:
After swapping: a = 10, b = 5
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请发表评论。