Django ModelForm是用于直接将模型转换为Django形式的类。如果你要构建数据库驱动的应用程序, 那么你的表格很可能会与Django模型紧密映射。例如, 用户注册模型和表单将具有相同数量和质量的模型字段和表单字段。因此, 我们可以直接使用ModelForm, 而无需创建多余的代码来先创建表单, 然后将其映射到视图中的模型。它以模型名称作为参数并将其转换为Django Form。不仅如此, ModelForm还提供了许多使整个过程自动化的方法和功能, 并有助于消除代码冗余。
如何将模型转换为Django表单?
为了解释项目的工作, 我们将使用项目极客, 创建一个模型并将其映射到Django表单。
请参阅以下文章, 以检查如何在Django中创建项目和应用。如何在Django中使用MVT创建基本项目?如何在Django中创建应用程序?
现在, 当我们准备好项目后, 在怪胎/models.py,
# import the standard Django Model
# from built-in library
from django.db import models
# declare a new model with a name "GeeksModel"
class GeeksModel(models.Model):
# fields of the model
title = models.CharField(max_length = 200 )
description = models.TextField()
last_modified = models.DateTimeField(auto_now_add = True )
img = models.ImageField(upload_to = "images/" )
# renames the instances of the model
# with their title name
def __str__( self ):
return self .title
现在, 运行以下命令来创建模型,
Python manage.py makemigrations
Python manage.py migrate
我们可以在以下位置检查是否已成功创建模型
http://127.0.0.1:8000/admin/geeks/geeksmodel/add/
,
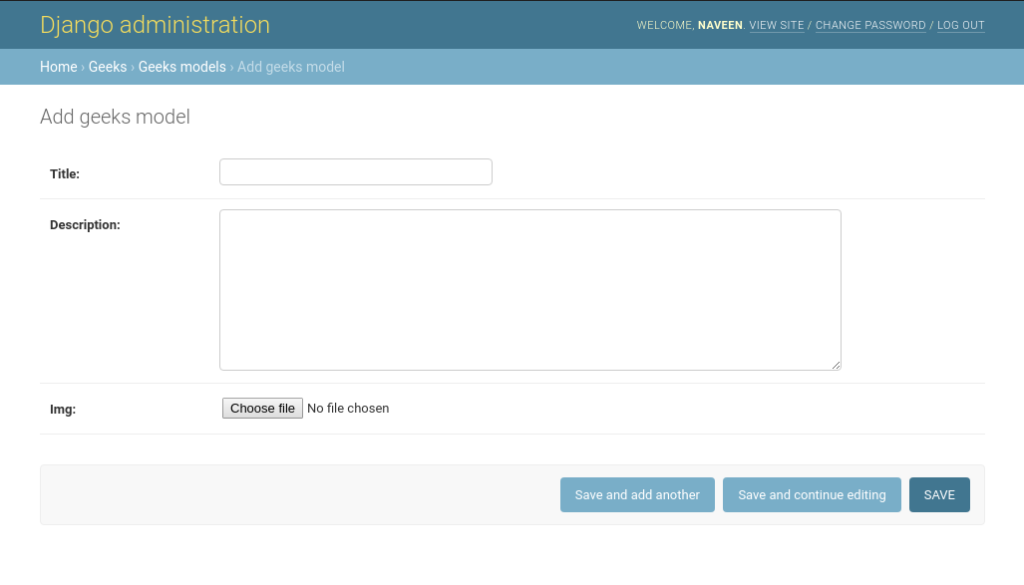
要直接为此模型创建表单, 请深入研究怪胎/forms.py然后输入以下代码
# import form class from django
from django import forms
# import GeeksModel from models.py
from .models import GeeksModel
# create a ModelForm
class GeeksForm(forms.ModelForm):
# specify the name of model to use
class Meta:
model = GeeksModel
fields = "__all__"
这种形式有两个参数领域or排除.
字段–强烈建议你使用fields属性来显式设置应在表单中编辑的所有字段。如果表单意外地允许用户设置某些字段, 特别是在将新字段添加到模型中时, 这样做失败很容易导致安全问题。根据表单的呈现方式, 问题甚至可能在网页上不可见。将fields属性设置为特殊值" __all__", 以指示应使用模型中的所有字段。
排除–
将ModelForm内部Meta类的exclude属性设置为要从表单中排除的字段列表。
例如:
class PartialAuthorForm(ModelForm):
class Meta:
model = Author
exclude = ['title']
最后, 要完成我们的MVT结构, 请创建一个视图, 该视图将呈现表单并将其直接保存到数据库中。在geeks/views.py,
from django.shortcuts import render
from .forms import GeeksForm
def home_view(request):
context = {}
# create object of form
form = GeeksForm(request.POST or None , request.FILES or None )
# check if form data is valid
if form.is_valid():
# save the form data to model
form.save()
context[ 'form' ] = form
return render(request, "home.html" , context)
一切就绪, 现在访问
http://127.0.0.1:8000/
,
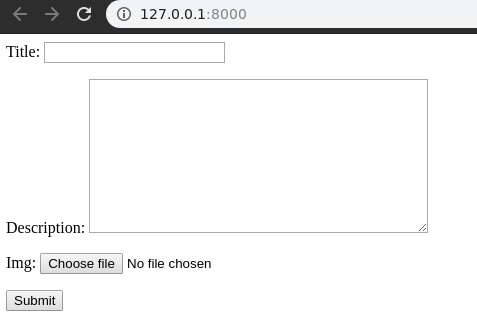
现在, 你可以看到每个模型字段都映射到一个表单字段并相应显示。字段映射将在本文后面讨论。因此, 现在让我们尝试将数据输入表单, 并检查是否将其保存到数据库中。
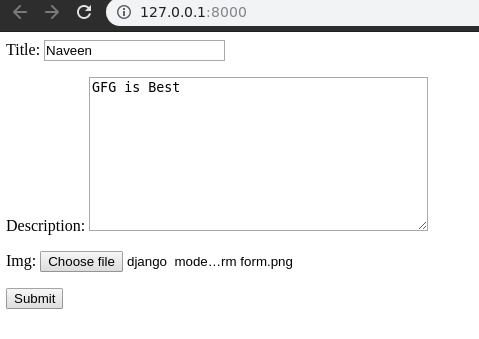
点击提交, 宾果游戏表格自动保存到数据库。我们可以在
http:// localhost:8000/admin/geeks/geeksmodel /
.
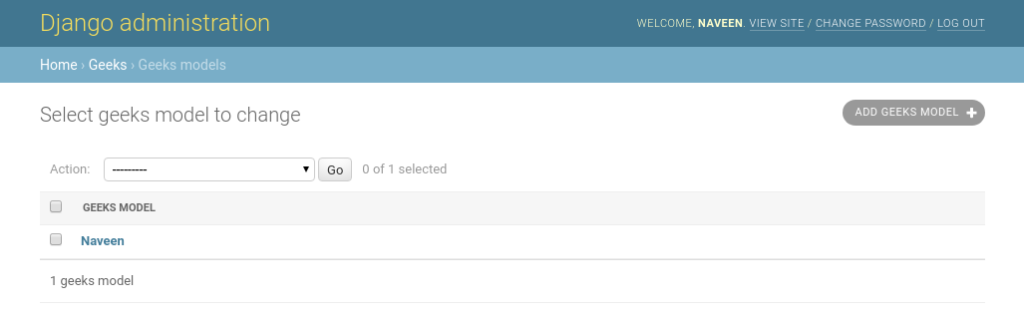
栏位类型
生成的Form类将为每个指定的模型字段都具有一个field字段, 并以fields属性中指定的顺序。每个模型字段都有一个对应的默认表单字段。例如, 模型上的CharField表示为表单上的CharField。模型ManyToManyField表示为MultipleChoiceField。以下是转换的完整列表:
模型领域 | 表格栏位 |
---|---|
AutoField | 不以形式表示 |
BigAutoField | 不以形式表示 |
BigIntegerField | IntegerField, 其min_value设置为-9223372036854775808, max_value设置为9223372036854775807。 |
BinaryField | CharField, 如果在模型字段上将editable设置为True, 则在表单中不表示。 |
BooleanField | BooleanField, 如果null = True, 则为NullBooleanField。 |
CharField | 如果null = True, 则将max_length设置为模型字段的max_length, 并将empty_value设置为None的CharField。 |
DateField | DateField |
DateTimeField | DateTimeField |
DecimalField | DecimalField |
DurationField | DurationField |
EmailField | EmailField |
FileField | FileField |
FilePathField | FilePathField |
FloatField | FloatField |
ForeignKey | ModelChoiceField |
ImageField | ImageField |
IntegerField | IntegerField |
IPAddressField | IPAddressField |
GenericIPAddressField | GenericIPAddressField |
ManyToManyField | ModelMultipleChoiceField |
NullBooleanField | NullBooleanField |
PositiveIntegerField | 整数字段 |
PositiveSmallIntegerField | IntegerField |
SlugField | SlugField |
SmallAutoField | 不以形式表示 |
TextField | 具有widget = forms.Textarea的CharField |
TimeField | TimeField |
URLField | URLField |
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。