本文概述
本文介绍如何使用给定的JSON数据创建Bootstrap表。可以通过导入数据或用JavaScript表示数据来检索数据。下面给出了两种执行此操作的方法。
在不导入任何文件的情况下显示JSON数据:可以使用JavaScript表示必须读取的JSON文件。然后可以将其提供给Bootstrap表来表示数据。
例子:
HTML代码示例
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<meta name = "viewport"
content=" width = device -width, initial-scale = 1 , shrink-to-fit = no ">
<title>
load data from json file
into a bootstrap table
</title>
<!-- Include Bootstrap for styling -->
<link rel = "stylesheet"
href =
"https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<!-- Include the Bootstrap Table CSS
for the table -->
<link rel = "stylesheet"
href =
"https://unpkg.com/bootstrap-table@1.16.0/dist/bootstrap-table.min.css">
</head>
<body>
<div class = "container">
<h1 class = "text text-success text-center ">
lsbin
</h1>
<h6 class = "text text-success text-center">
A computer science portal for geeks
</h6>
<table class = "table-striped border-success">
<thead>
<tr>
<th data-field = "id">
<span class = "text-success">
Employee ID
</span>
</th>
<th data-field = "name">
<span class = "text-success">
Employee Name
</span>
</th>
<th data-field = "date">
<span class = "text-success">
Joining Date
</span>
</th>
</tr>
</thead>
</table>
</div>
<!-- Include jQuery and other required
files for Bootstrap -->
<script src =
"https://code.jquery.com/jquery-3.3.1.min.js">
</script>
<script src =
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src =
"https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
<!-- Include the JavaScript file
for Bootstrap table -->
<script src =
"https://unpkg.com/bootstrap-table@1.16.0/dist/bootstrap-table.min.js">
</script>
<script type = "text/javascript">
$(document).ready(function () {
//Use the given data to create
//the table and display it
$('table').bootstrapTable({
data: mydata
});
});
//Specify the JSON data to be displayed
var mydata =
[
{
"id": "24323", "name": "Mark Smith", "date": "25/5/2020"
}, {
"id": "24564", "name": "Caitlin MacDonald", "date": "17/5/2020"
}, {
"id": "24345", "name": "Jessie Johnson ", "date": "1/5/2020"
}, {
"id": "24874", "name": "Alen Williams", "date": "14/5/2020"
}, {
"id": "24323", "name": "Maria Garcia ", "date": "13/5/2020"
}
];
</script>
</body>
</html>
输出如下:
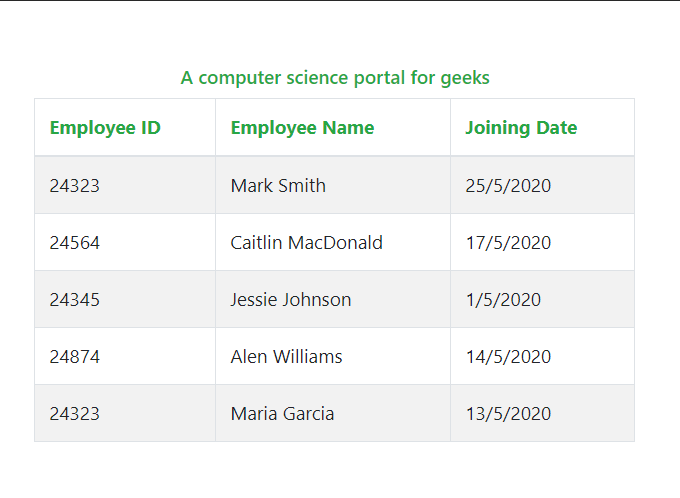
从文件导入后显示JSON数据:要显示的JSON数据存储在导入的JavaScript文件中的本地文件夹中。然后可以将此导入的数据提供给Bootstrap表以表示数据。 ES6功能反引号(``)可用于多行字符串值插值。
例子:
JSON数据:的JSON数据存储在以下示例中:
JavaScript代码
//Contents for test.js
//Represents JSON of the data
var da = `[
{ "id" : "24323" , "name" : "Mark Smith" , "date" : "25/5/2020" }, { "id" : "24564" , "name" : "Caitlin MacDonald" , "date" : "17/5/2020" }
]`;
程序:将数据从JSON加载到Bootstrap表中
HTML代码示例
<!DOCTYPE html>
<html lang = "en">
<head>
<meta charset = "utf-8">
<meta name = "viewport"
content=" width = device -width, initial-scale = 1 , shrink-to-fit = no ">
<title>
load data from json file
into a bootstrap table
</title>
<!-- Include Bootstrap for styling -->
<link rel = "stylesheet"
href =
"https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css">
<!-- Include the Bootstrap Table
CSS for the table -->
<link rel = "stylesheet"
href =
"https://unpkg.com/bootstrap-table@1.16.0/dist/bootstrap-table.min.css">
</head>
<body>
<div class = "container">
<h1 class = "text text-success text-center ">
lsbin
</h1>
<h6 class = "text text-success text-center">
A computer science portal for geeks
</h6>
<table class = "table-striped border-success">
<thead>
<tr>
<th data-field = "id">
<span class = "text-success">
Employee ID
</span>
</th>
<th data-field = "name">
<span class = "text-success">
Employee Name
</span>
</th>
<th data-field = "date">
<span class = "text-success">
Joining Date
</span>
</th>
</tr>
</thead>
</table>
</div>
<!-- Include jQuery and other required files for Bootstrap -->
<script src =
"https://code.jquery.com/jquery-3.3.1.min.js">
</script>
<script src =
"https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js">
</script>
<script src =
"https://stackpath.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js">
</script>
<!-- Include the JavaScript file for Bootstrap table -->
<script src =
"https://unpkg.com/bootstrap-table@1.16.0/dist/bootstrap-table.min.js">
</script>
<!-- Include the file where the JSON data is stored -->
<script type = "text/javascript"
src = "test.js">
</script>
<script type = "text/javascript">
$(document).ready(function () {
//Use the given data to create
//the table and display it
$('table').bootstrapTable({
data: mydata
});
});
//Parse the imported data as JSON
//and store it
var mydata = JSON.parse(da)
</script>
</body>
</html>
输出如下:
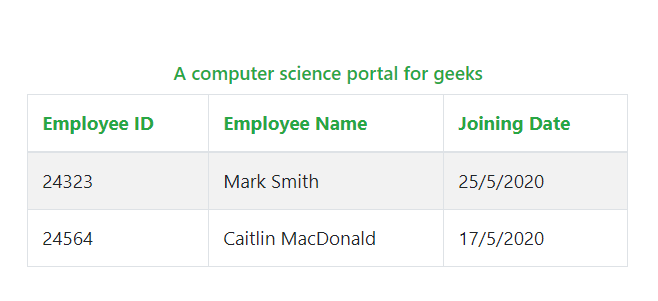