本文概述
给定一个数字n,我们需要找出它的数字和,使:
If n < 10
digSum(n) = n
Else
digSum(n) = Sum(digSum(n))
例子 :
Input : 1234
Output : 1
Explanation : The sum of 1+2+3+4 = 10, digSum(x) == 10
Hence ans will be 1+0 = 1
Input : 5674
Output : 4
蛮力方法是对所有数字求和,直到sum < 10。
流程图:
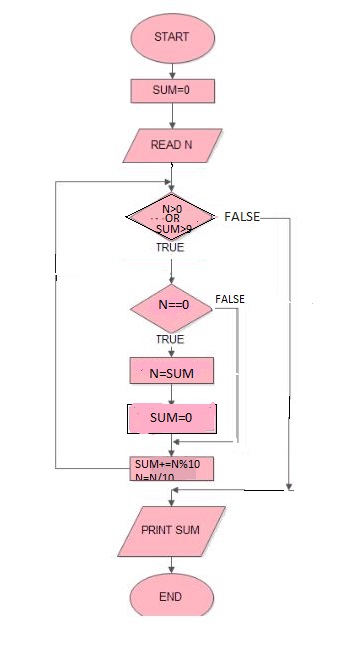
下面是蛮力程序, 用于找到总和。
C ++
// C++ program to find sum of
// digits of a number until
// sum becomes single digit.
#include<bits/stdc++.h>
using namespace std;
int digSum( int n)
{
int sum = 0;
// Loop to do sum while
// sum is not less than
// or equal to 9
while (n > 0 || sum > 9)
{
if (n == 0)
{
n = sum;
sum = 0;
}
sum += n % 10;
n /= 10;
}
return sum;
}
// Driver program to test the above function
int main()
{
int n = 1234;
cout << digSum(n);
return 0;
}
Java
// Java program to find sum of
// digits of a number until
// sum becomes single digit.
import java.util.*;
public class GfG {
static int digSum( int n)
{
int sum = 0 ;
// Loop to do sum while
// sum is not less than
// or equal to 9
while (n > 0 || sum > 9 )
{
if (n == 0 ) {
n = sum;
sum = 0 ;
}
sum += n % 10 ;
n /= 10 ;
}
return sum;
}
// Driver code
public static void main(String argc[])
{
int n = 1234 ;
System.out.println(digSum(n));
}
}
// This code is contributed by Gitanjali.
python
# Python program to find sum of
# digits of a number until
# sum becomes single digit.
import math
# method to find sum of digits
# of a number until sum becomes
# single digit
def digSum( n):
sum = 0
while (n > 0 or sum > 9 ):
if (n = = 0 ):
n = sum
sum = 0
sum + = n % 10
n / = 10
return sum
# Driver method
n = 1234
print (digSum(n))
# This code is contributed by Gitanjali.
C#
// C# program to find sum of
// digits of a number until
// sum becomes single digit.
using System;
class GFG {
static int digSum( int n)
{
int sum = 0;
// Loop to do sum while
// sum is not less than
// or equal to 9
while (n > 0 || sum > 9)
{
if (n == 0)
{
n = sum;
sum = 0;
}
sum += n % 10;
n /= 10;
}
return sum;
}
// Driver code
public static void Main()
{
int n = 1234;
Console.Write(digSum(n));
}
}
// This code is contributed by nitin mittal
的PHP
<?php
// PHP program to find sum of
// digits of a number until
// sum becomes single digit.
function digSum( $n )
{
$sum = 0;
// Loop to do sum while
// sum is not less than
// or equal to 9
while ( $n > 0 || $sum > 9)
{
if ( $n == 0)
{
$n = $sum ;
$sum = 0;
}
$sum += $n % 10;
$n = (int) $n / 10;
}
return $sum ;
}
// Driver Code
$n = 1234;
echo digSum( $n );
// This code is contributed
// by aj_36
?>
输出:
1
存在一个简单而优雅的O(1)解决方案为此。答案很简单:-
If n == 0
return 0;
If n % 9 == 0
digSum(n) = 9
Else
digSum(n) = n % 9
以上逻辑如何运作?
如果数字n可以被9整除, 那么直到位数变成一位数字之前, 其位数的总和始终为9。例如,
设n = 2880
数字总和= 2 + 8 + 8 = 18:18 = 1 + 8 = 9
一个数字可以是9x或9x + k的形式。对于第一种情况, 答案始终为9。对于第二种情况, 答案始终为k。
下面是上述想法的实现:
CPP
#include<bits/stdc++.h>
using namespace std;
int digSum( int n)
{
if (n == 0)
return 0;
return (n % 9 == 0) ? 9 : (n % 9);
}
// Driver program to test the above function
int main()
{
int n = 9999;
cout<<digSum(n);
return 0;
}
Java
import java.io.*;
class GFG {
static int digSum( int n)
{
if (n == 0 )
return 0 ;
return (n % 9 == 0 ) ? 9 : (n % 9 );
}
// Driver program to test the above function
public static void main (String[] args)
{
int n = 9999 ;
System.out.println(digSum(n));
}
}
// This code is contributed by anuj_67.
Python3
def digSum(n):
if (n = = 0 ):
return 0
if (n % 9 = = 0 ):
return 9
else :
(n % 9 )
# Driver program to test the above function
n = 9999
print (digSum(n))
# This code is contributed by
# Smitha Dinesh Semwal
C#
using System;
class GFG
{
static int digSum( int n)
{
if (n == 0)
return 0;
return (n % 9 == 0) ? 9 : (n % 9);
}
// Driver Code
public static void Main ()
{
int n = 9999;
Console.Write(digSum(n));
}
}
// This code is contributed by aj_36
的PHP
<?php
function digSum( $n )
{
if ( $n == 0)
return 0;
return ( $n % 9 == 0) ? 9 : ( $n % 9);
}
// Driver program to test the above function
$n = 9999;
echo digSum( $n );
//This code is contributed by anuj_67.
?>
输出如下:
9
相关文章:
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。