有一些过滤器在AngularJS中添加的代码是为了简化格式设置和处理数据。 AngularJS中有几个内置过滤器。它们与一些示例一起在此处列出, 以使理解更加容易。
基本语法:
通常使用竖线(|)字符将过滤器添加到表达式中。
例如, 过滤器{{fullName |大写}}将fullName格式化为大写格式。
AngularJS中的一些预先构建的过滤器是:
- 货币该数字被格式化为货币格式。
- 日期日期以特定格式指定。
- 过滤根据提供的条件对数组进行过滤。
- 限制于数组或字符串限制为指定数量的元素/字符。
- Number如果格式化为字符串, 则为数字。
- 该数组由表达式排序。
- 小写此过滤器将字符串转换为小写字母。
- 大写此过滤器将字符串转换为大写字母。
- JSON它将JavaScript对象转换为JSON字符串。
该过滤器只是将数字格式化为货币。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "costCtrl">
<h1>Currency Format - lsbin</h1>
<h2>Price: {{ price | currency }}</h2>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('costCtrl', function($scope) {
$scope.price = 20;
});
</script>
</body>
</html>
输出如下:
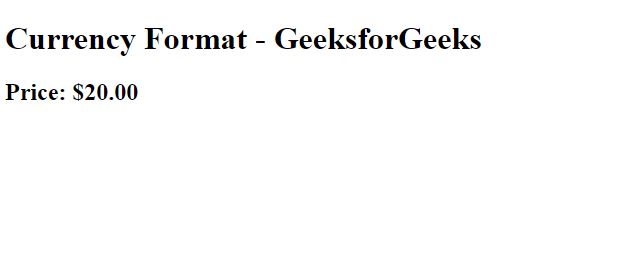
在这里, 美元($)符号已自动添加到数值前面。
日期过滤器:日期过滤器将日期格式化为指定的格式。
语法如下:
{{ date | date : format : timezone }}
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "datCtrl">
<h1>lsbin - Date Filter</h1>
<p>Date = {{ today | date }}</p>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('datCtrl', function($scope) {
$scope.today = new Date();
});
</script>
</body>
</html>
输出如下:
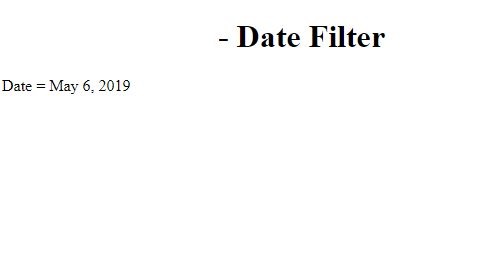
在这里日期()功能已被使用, 并显示当前日期。
过滤:这仅用于显示所需的对象。过滤器选择数组的子集。
例如, 此过滤器只能在数组上使用, 因为它将返回仅包含匹配项的数组(数组中给定的条件)。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "namesCtrl">
<h1>filter - lsbin</h1>
<ul>
<li ng-repeat = "x in names | filter : 'e'">
{{ x }}
</li>
</ul>
</div>
<script>
angular.module('myApp', []).controller('namesCtrl', function($scope) {
$scope.names = [
'Jani', 'Carl', 'Margareth', 'Hege', 'Joe', 'Gustav', 'Birgit', 'Mary', 'Kai'
];
});
</script>
<p>This example displays only the names
containing the letter "e".</p>
</body>
</html>
输出如下:
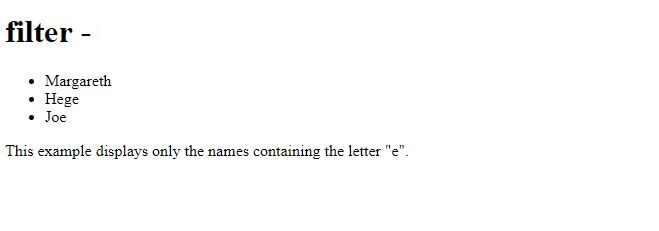
limitTo过滤器:该过滤器返回仅包含指定数量的元素的数组或字符串。输出将取决于提供给程序的输入类型。当用于数组时, 它返回仅包含指定数量的项目的数组。
对于字符串, 它将返回仅包含指定数目的字符的字符串, 而当用于数字时, 它将返回仅包含指定数目的数字的字符串。
语法如下:
{{ object | limitTo : limit : begin }}
在这里, limit指定要显示的元素数量, 而begin指定从何处开始限制。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "sizeCtrl">
<h1>limitTo - lsbin</h1>
<ul>
<li ng-repeat = "x in cars | limitTo : 4 : 1">{{x}}</li>
</ul>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('sizeCtrl', function($scope) {
$scope.cars = ["Audi", "BMW", "Dodge", "Fiat", "Ford", "Volvo", "Lamborghini"];
});
</script>
<p>Filter applied from first
element to the fifth element.</p>
</body>
</html>
输出如下:
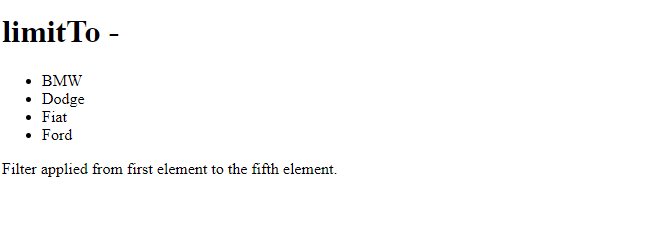
orderBy过滤器:
这用于对数组进行排序。可以使用此过滤器对字符串(默认字母顺序)和数字(默认升序)进行排序。
语法如下:
{{ array | orderBy : expression : reverse }}
在这里, 反转可以用来反转结果数组的顺序。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "orderCtrl">
<h1>orderBy - lsbin</h1>
<ul>
<li ng-repeat = "x in customers | orderBy : 'city'">
{{x.name + ", " + x.city}}
</li>
</ul>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('orderCtrl', function($scope) {
$scope.customers = [{
"name": "Delhi"
}, {
"name": "Mumbai"
}, {
"name": "Patna"
}, {
"name": "Kolkata"
}, {
"name": "Pune"
}, {
"name": "Ranchi"
}, {
"name": "Bhopal"
}];
});
</script>
<p>Sorting in ascending order.</p>
</body>
</html>
输出如下:
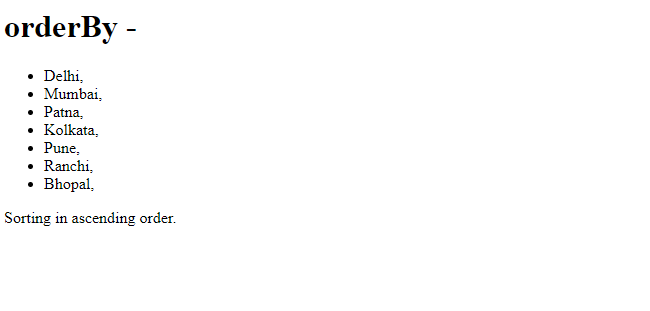
数字过滤器:
可能是最简单的过滤器。它只是将数字格式化为字符串。
语法如下:
{{ string | number : fractionsize}}
这里, "分数大小"指定小数位数。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "nCtrl">
<h1>number Filter - lsbin</h1>
<h2>Rs.{{money | number : 3}}</h2>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('nCtrl', function($scope) {
$scope.money = 999999;
});
</script>
<p>The money is written with three decimals.</p>
</body>
</html>
输出如下:
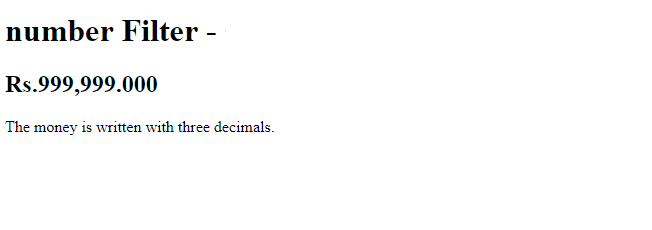
小写过滤器:
该过滤器只是将字符串转换为小写字母。
语法如下:
{{ string | lowercase }}
让我们看一个例子来弄清楚这个过滤器。
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<h2>AngularJS - lowercase</h2>
<br>
<br>
<div ng-app = "myApp" ng-controller = "myCtrl">
<strong>Input:</strong>
<br>
<input type = "text" ng-model = "string">
<br>
<br>
<strong>Output:</strong>
<br> {{string|lowercase}}
</div>
<script>
var app = angular.module('myApp', []);
app.controller('myCtrl', function($scope) {
$scope.string = "";
});
</script>
</body>
</html>
上面提到的代码要求用户输入。用户在输入框中输入术语后, 该术语将存储在
ng-model =""字串"
。现在, AngularJS将解析该表达式, 并精确地将表达式返回的位置返回结果。 AngularJS表达式可以写在双括号内, 如下所示:
{{表达式}}.
输出如下:
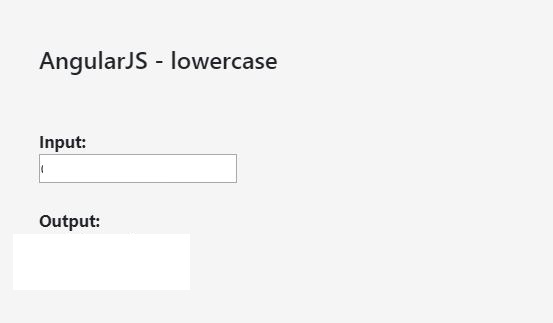
在这段代码中, 输出{{串}}显示在输入框的正下方。但是, 要将输入字符串更改为小写, ‘|小写’必须添加到表达式的名称中。
因此, {{string |小写}}将以小写格式返回输入字符串。
大写过滤器:
AngularJS中的大写过滤器用于将字符串更改为大写字符串或字母。
语法如下:
{{ string | uppercase}}
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "caseCtrl">
<h1>{{txt | uppercase}}</h1>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('caseCtrl', function($scope) {
$scope.txt = "lsbin!";
});
</script>
<p>The text is written in uppercase letters.</p>
</body>
</html>
输出如下:
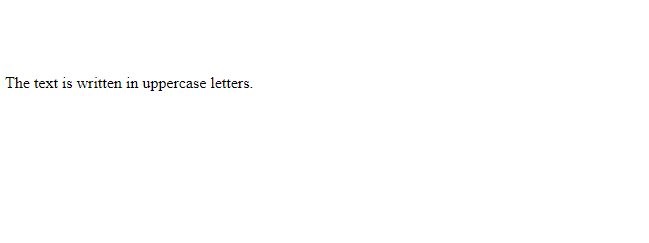
json过滤器:
该过滤器仅将JavaScript对象转换为JSON字符串, 这在调试应用程序时非常有用。
语法如下:
{{ object | json : spacing }}
在此, 间距指定每个缩进使用的空格数。默认值为2, 但是此值是可选的。
看下面的示例代码:
<!DOCTYPE html>
<html>
<script src =
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js">
</script>
<body>
<div ng-app = "myApp" ng-controller = "jsCtrl">
<h1>lsbin</h1>
<pre>{{customer | json : 20}}</pre>
</div>
<script>
var app = angular.module('myApp', []);
app.controller('jsCtrl', function($scope) {
$scope.customer = {
"name" : "Milk", "city" : "Patna", "country" : "India"
};
});
</script>
<p>A JSON string with 20 spaces per indentation.</p>
</body>
</html>
输出如下:
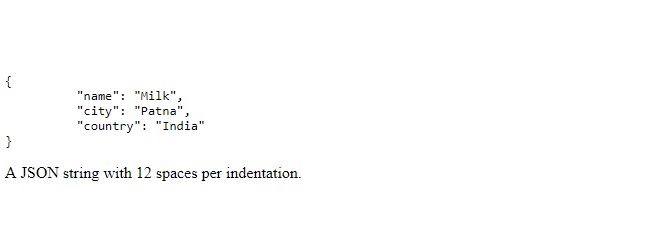
让我们看一下数组过滤器的示例。
<!DOCTYPE html>
<html>
<body>
<h1>lsbin</h1>
<p>Click the button to get every element
in the array that has a value of 38 or more.</p>
<button onclick = "myFunction()">Try it</button>
<p id = "demo"></p>
<script>
var num = [23, 32, 56, 30, 56, 45, 34, 39];
function checkNum(num) {
return num>= 38;
}
function myFunction() {
document.getElementById(
"demo").innerHTML = num.filter(checkNum);
}
</script>
</body>
</html>
在点击
'试试看'
按钮, 数组中所有值大于或等于38的元素都将显示在屏幕上。
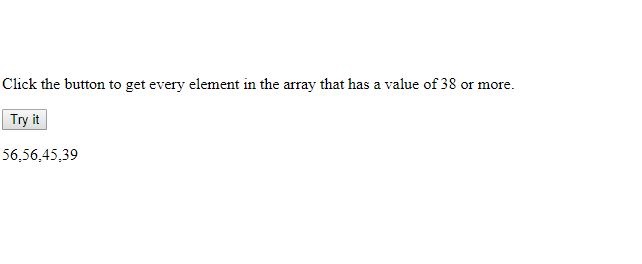