- 功能是对象;它们可以被引用, 传递给变量并从其他函数返回。
- 函数可以在另一个函数中定义, 也可以作为参数传递给另一个函数。
装饰工是Python中非常强大且有用的工具, 因为它允许程序员修改函数或类的行为。装饰器允许我们包装另一个函数, 以扩展包装函数的行为, 而无需对其进行永久性修改。
在Decorators中, 将函数作为另一个函数的参数, 然后在包装函数内部调用。
装饰器的语法:
@gfg_decorator
def hello_decorator():
print ( "Gfg" )
'''Above code is equivalent to -
def hello_decorator():
print("Gfg")
hello_decorator = gfg_decorator(hello_decorator)'''
在上面的代码中, gfg_decorator是一个可调用的函数, 将在另一个可调用函数的顶部添加一些代码, hello_decorator函数并返回包装函数。
装饰者可以修改行为:
# defining a decorator
def hello_decorator(func):
# inner1 is a Wrapper function in
# which the argument is called
# inner function can access the outer local
# functions like in this case "func"
def inner1():
print ( "Hello, this is before function execution" )
# calling the actual function now
# inside the wrapper function.
func()
print ( "This is after function execution" )
return inner1
# defining a function, to be called inside wrapper
def function_to_be_used():
print ( "This is inside the function !!" )
# passing 'function_to_be_used' inside the
# decorator to control its behavior
function_to_be_used = hello_decorator(function_to_be_used)
# calling the function
function_to_be_used()
输出如下:
Hello, this is before function execution
This is inside the function !!
This is after function execution
让我们看看上面的代码在调用" function_to_be_used"时如何逐步运行。
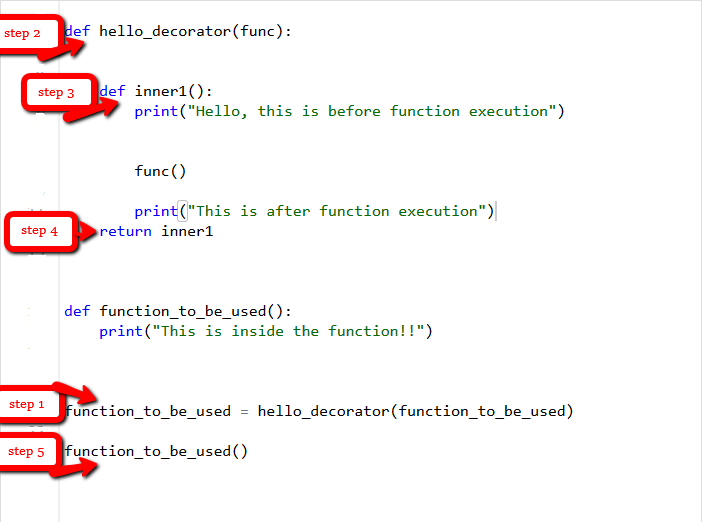
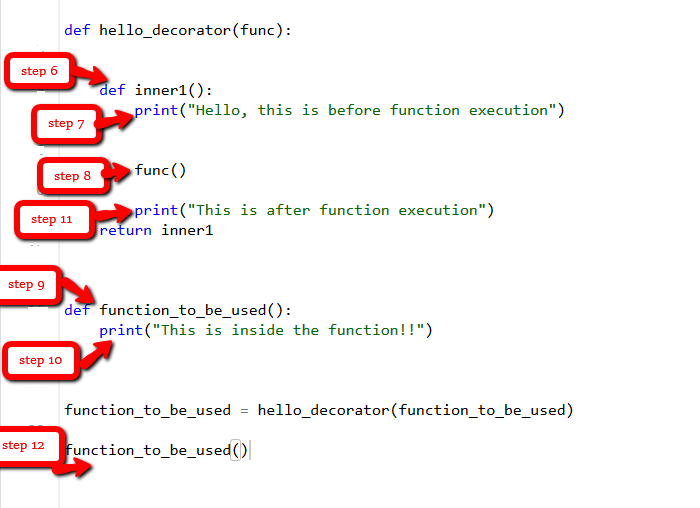
让我们跳到另一个例子, 我们可以轻松地使用装饰器找出函数的执行时间
# importing libraries
import time
import math
# decorator to calculate duration
# taken by any function.
def calculate_time(func):
# added arguments inside the inner1, # if function takes any arguments, # can be added like this.
def inner1( * args, * * kwargs):
# storing time before function execution
begin = time.time()
func( * args, * * kwargs)
# storing time after function execution
end = time.time()
print ( "Total time taken in : " , func.__name__, end - begin)
return inner1
# this can be added to any function present, # in this case to calculate a factorial
@calculate_time
def factorial(num):
# sleep 2 seconds because it takes very less time
# so that you can see the actual difference
time.sleep( 2 )
print (math.factorial(num))
# calling the function.
factorial( 10 )
输出如下:
3628800
Total time taken in : factorial 2.0061802864074707
如果一个函数返回一些东西怎么办–
在以上所有示例中, 这些函数均未返回任何内容, 因此没有任何问题, 但是可能需要返回的值。
def hello_decorator(func):
def inner1( * args, * * kwargs):
print ( "before Execution" )
# getting the returned value
returned_value = func( * args, * * kwargs)
print ( "after Execution" )
# returning the value to the original frame
return returned_value
return inner1
# adding decorator to the function
@hello_decorator
def sum_two_numbers(a, b):
print ( "Inside the function" )
return a + b
a, b = 1 , 2
# getting the value through return of the function
print ( "Sum =" , sum_two_numbers(a, b))
输出如下:
before Execution
Inside the function
after Execution
Sum = 3
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。