Java提供了许多类型的运算符, 可以根据需要使用它们。根据提供的功能对它们进行分类。其中一些类型是-
让我们详细看看它们。
算术运算符:
它们用于对原始数据类型执行简单的算术运算。
- *:乘法
- /:师
- %:模数
- +:加成
- –:减法
// Java program to illustrate
// arithmetic operators
public class operators {
public static void main(String[] args)
{
int a = 20 , b = 10 , c = 0 , d = 20 , e = 40 , f = 30 ;
String x = "Thank" , y = "You" ;
// + and - operator
System.out.println( "a + b = " + (a + b));
System.out.println( "a - b = " + (a - b));
// + operator if used with strings
// concatenates the given strings.
System.out.println( "x + y = " + x + y);
// * and / operator
System.out.println( "a * b = " + (a * b));
System.out.println( "a / b = " + (a / b));
// modulo operator gives remainder
// on dividing first operand with second
System.out.println( "a % b = " + (a % b));
// if denominator is 0 in division
// then Arithmetic exception is thrown.
// uncommenting below line would throw
// an exception
// System.out.println(a/c);
}
}
输出如下:
a + b = 30
a - b = 10
x + y = ThankYou
a * b = 200
a / b = 2
a % b = 0
一元运算符:
一元运算符只需要一个操作数。它们用于递增, 递减或取反值。
- –:一元减, 用于取反值。
- +:一元加, 用于给出正值。仅在故意将负值转换为正值时使用。
- ++:增量运算符, 用于将值递增1。递增运算符有两种。
- 后递增:值首先用于计算结果, 然后递增。
- 预递增:值先增加, 然后计算结果。
- —:减量运算符, 用于将值减1。减数运算符有两种。
- 递减后:值首先用于计算结果, 然后递减。
- 递减前:值先递减, 然后计算结果。
- ! :逻辑非运算符, 用于反转布尔值。
// Java program to illustrate
// unary operators
public class operators {
public static void main(String[] args)
{
int a = 20 , b = 10 , c = 0 , d = 20 , e = 40 , f = 30 ;
boolean condition = true ;
// pre-increment operator
// a = a+1 and then c = a;
c = ++a;
System.out.println( "Value of c (++a) = " + c);
// post increment operator
// c=b then b=b+1
c = b++;
System.out.println( "Value of c (b++) = " + c);
// pre-decrement operator
// d=d-1 then c=d
c = --d;
System.out.println( "Value of c (--d) = " + c);
// post-decrement operator
// c=e then e=e-1
c = e--;
System.out.println( "Value of c (e--) = " + c);
// Logical not operator
System.out.println( "Value of !condition ="
+ !condition);
}
}
输出如下:
Value of c (++a) = 21
Value of c (b++) = 10
Value of c (--d) = 19
Value of c (e--) = 40
Value of !condition =false
分配运算符:" ="
赋值运算符用于为任何变量赋值。它具有从右到左的关联性, 即在运算符的右侧给出的值被分配给左侧的变量, 因此, 在使用它之前必须声明右侧的值, 或者应该是一个常数。
赋值运算符的一般格式是
variable = value;
在许多情况下, 赋值运算符可以与其他运算符结合使用, 以构建称为以下内容的较短版本的语句复合陈述。例如, 代替=a + 5, 我们可以写一个+ =5.
- + =, 用于将左侧操作数与右侧操作数相加, 然后将其分配给左侧的变量。
- -=, 用于用右侧操作数减去左侧操作数, 然后将其分配给左侧的变量。
- * =, 用于将左操作数与右操作数相乘, 然后将其分配给左侧的变量。
- / =, 用于将左操作数除以右操作数, 然后将其分配给左侧的变量。
- %=, 用于为左操作数与右操作数分配模, 然后将其分配给左侧的变量。
int a = 5;
a += 5; //a = a+5;
// Java program to illustrate
// assignment operators
public class operators {
public static void main(String[] args)
{
int a = 20 , b = 10 , c, d, e = 10 , f = 4 , g = 9 ;
// simple assignment operator
c = b;
System.out.println( "Value of c = " + c);
// This following statement would throw an exception
// as value of right operand must be initialised
// before assignment, and the program would not
// compile.
// c = d;
// instead of below statements, shorthand
// assignment operators can be used to
// provide same functionality.
a = a + 1 ;
b = b - 1 ;
e = e * 2 ;
f = f / 2 ;
System.out.println( "a, b, e, f = " + a + ", "
+ b + ", " + e + ", " + f);
a = a - 1 ;
b = b + 1 ;
e = e / 2 ;
f = f * 2 ;
// shorthand assignment operator
a += 1 ;
b -= 1 ;
e *= 2 ;
f /= 2 ;
System.out.println( "a, b, e, f ("
+ "using shorthand operators)= "
+ a + ", " + b + ", "
+ e + ", " + f);
}
}
输出如下:
Value of c = 10
a, b, e, f = 21, 9, 20, 2
a, b, e, f (using shorthand operators)= 21, 9, 20, 2
这些运算符用于检查诸如相等, 大于, 小于等关系。它们在比较之后返回布尔结果, 并广泛用于循环语句以及条件if语句中。通用格式是
variable relation_operator value
一些关系运算符是-
- ==, 等于:左侧的true等于右侧的true。
- !=, 不等于:左边的返回值不等于右边的返回值true。
- <, 小于:左手边小于右手边返回true。
- <=, 小于或等于:左侧的小于或等于右侧的返回true。
- >, 大于:左手侧大于右手侧返回true。
- > =, 大于或等于:左侧大于或等于右侧的返回true。
// Java program to illustrate
// relational operators
public class operators {
public static void main(String[] args)
{
int a = 20 , b = 10 ;
String x = "Thank" , y = "Thank" ;
int ar[] = { 1 , 2 , 3 };
int br[] = { 1 , 2 , 3 };
boolean condition = true ;
// various conditional operators
System.out.println( "a == b :" + (a == b));
System.out.println( "a < b :" + (a < b));
System.out.println( "a <= b :" + (a <= b));
System.out.println( "a > b :" + (a > b));
System.out.println( "a >= b :" + (a >= b));
System.out.println( "a != b :" + (a != b));
// Arrays cannot be compared with
// relational operators because objects
// store references not the value
System.out.println( "x == y : " + (ar == br));
System.out.println( "condition==true :"
+ (condition == true ));
}
}
输出如下:
a == b :false
a < b :false
a <= b :false
a > b :true
a >= b :true
a != b :true
x == y : false
condition==true :true
这些运算符用于执行"逻辑与"和"逻辑或"操作, 即类似于数字电子设备中的"与"门和"或"门的功能。要记住的一件事是, 如果第一个条件为假, 则不评估第二个条件, 即它具有短路作用。广泛用于测试决策的几个条件。
条件运算符是-
- &&, 逻辑与:当两个条件都成立时, 返回true。
- ||, 逻辑或:如果至少一个条件为true, 则返回true。
// Java program to illustrate
// logical operators
import java.util.*;
public class operators {
public static void main(String[] args)
{
String x = "Sher" ;
String y = "Locked" ;
Scanner s = new Scanner(System.in);
System.out.print( "Enter username:" );
String uuid = s.next();
System.out.print( "Enter password:" );
String upwd = s.next();
// Check if user-name and password match or not.
if ((uuid.equals(x) && upwd.equals(y))
|| (uuid.equals(y) && upwd.equals(x))) {
System.out.println( "Welcome user." );
}
else {
System.out.println( "Wrong uid or password" );
}
}
}
输出如下:
Enter username:Sher
Enter password:Locked
Welcome user.
三元运算符:
三元运算符是if-else语句的简写形式。它具有三个操作数, 因此名称为三元。通用格式为-
condition ? if true : if false
上面的语句表示如果条件的计算结果为true, 则在'?'之后执行语句, 否则在':'之后执行语句。
// Java program to illustrate
// max of three numbers using
// ternary operator.
public class operators {
public static void main(String[] args)
{
int a = 20 , b = 10 , c = 30 , result;
// result holds max of three
// numbers
result = ((a > b)
? (a > c)
? a
: c
: (b > c)
? b
: c);
System.out.println( "Max of three numbers = "
+ result);
}
}
输出如下:
Max of three numbers = 30
按位运算符:
这些运算符用于对数字的各个位进行操作。它们可以与任何整数类型一起使用。在执行二进制索引树的更新和查询操作时使用它们。
- &, 按位AND运算符:返回输入值的位AND。
- |, 按位或运算符:返回输入值的位或。
- ^, 按位XOR运算符:返回输入值的XOR。
- 〜, 按位补码运算符:这是一元运算符, 用于返回输入值的补码表示形式, 即所有位都取反。
// Java program to illustrate
// bitwise operators
public class operators {
public static void main(String[] args)
{
// if int a = 010
// Java considers it as octal value
// of 8 as number starts with 0.
int a = 0x0005 ;
int b = 0x0007 ;
// bitwise and
// 0101 & 0111=0101
System.out.println( "a&b = " + (a & b));
// bitwise and
// 0101 | 0111=0111
System.out.println( "a|b = " + (a | b));
// bitwise xor
// 0101 ^ 0111=0010
System.out.println( "a^b = " + (a ^ b));
// bitwise and
// ~0101=1010
System.out.println( "~a = " + ~a);
// can also be combined with
// assignment operator to provide shorthand
// assignment
// a=a&b
a &= b;
System.out.println( "a= " + a);
}
}
输出如下:
a&b = 5
a|b = 7
a^b = 2
~a = -6
a= 5
移位运算符:
这些运算符用于将数字的位向左或向右移位, 从而分别将数字乘以或除以二。当我们必须将数字乘以或除以2时, 可以使用它们。通用格式
number shift_op number_of_places_to_shift;
- <<, 左移运算符:将数字的位向左移动, 结果在空白处填充0。与将数字乘以2的乘方相似的效果。
- >>, 带符号的右移运算符:将数字的位数向右移动, 结果在左侧的空白处填充0。最左边的位取决于初始编号的符号。将数字除以二的幂具有相似的效果。
- >>>, 无符号右移运算符:将数字的位数向右移动, 结果在左侧的空白处填充0。最左边的位设置为0。
// Java program to illustrate
// shift operators
public class operators {
public static void main(String[] args)
{
int a = 0x0005 ;
int b = - 10 ;
// left shift operator
// 0000 0101<<2 =0001 0100(20)
// similar to 5*(2^2)
System.out.println( "a<<2 = " + (a << 2 ));
// right shift operator
// 0000 0101 >> 2 =0000 0001(1)
// similar to 5/(2^2)
System.out.println( "a>>2 = " + (a >> 2 ));
// unsigned right shift operator
System.out.println( "b>>>2 = " + (b >>> 2 ));
}
}
输出如下:
a<<2 = 20
a>>2 = 1
b>>>2 = 1073741821
运算符实例
:
运算符的实例用于类型检查。它可用于测试对象是否是类, 子类或接口的实例。通用格式
object instance of class/subclass/interface
// Java program to illustrate
// instance of operator
class operators {
public static void main(String[] args)
{
Person obj1 = new Person();
Person obj2 = new Boy();
// As obj is of type person, it is not an
// instance of Boy or interface
System.out.println( "obj1 instanceof Person: "
+ (obj1 instanceof Person));
System.out.println( "obj1 instanceof Boy: "
+ (obj1 instanceof Boy));
System.out.println( "obj1 instanceof MyInterface: "
+ (obj1 instanceof MyInterface));
// Since obj2 is of type boy, // whose parent class is person
// and it implements the interface Myinterface
// it is instance of all of these classes
System.out.println( "obj2 instanceof Person: "
+ (obj2 instanceof Person));
System.out.println( "obj2 instanceof Boy: "
+ (obj2 instanceof Boy));
System.out.println( "obj2 instanceof MyInterface: "
+ (obj2 instanceof MyInterface));
}
}
class Person {
}
class Boy extends Person implements MyInterface {
}
interface MyInterface {
}
输出如下:
obj1 instanceof Person: true
obj1 instanceof Boy: false
obj1 instanceof MyInterface: false
obj2 instanceof Person: true
obj2 instanceof Boy: true
obj2 instanceof MyInterface: true
运算符的优先级和关联性
在处理涉及一种以上类型算子的混合方程时, 将使用优先规则和关联规则。在这种情况下, 这些规则将确定首先考虑方程式的哪一部分, 因为同一方程式可能会有许多不同的评估。下表按大小降序描述了运算符的优先级, 其幅度最高, 最高的代表最低, 最低的代表最低。
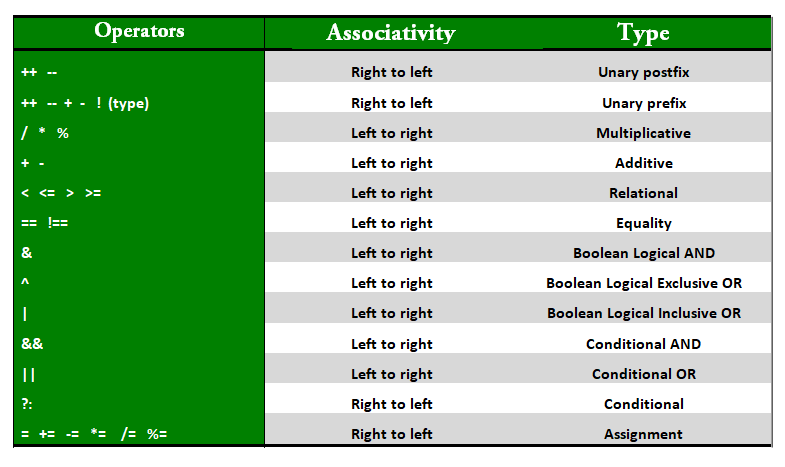
运算符有趣的问题
优先顺序和关联性:当涉及混合方程时, 通常会产生混淆, 混合方程是具有多个运算符的方程。问题是首先要解决哪一部分。在这些情况下, 有一条黄金法则可以遵循。如果运算符具有不同的优先级, 请首先解决较高的优先级。如果它们具有相同的优先级, 请根据关联性进行求解, 即从右到左或从左到右。以下程序的解释很好地写在了程序本身的注释中。
public class operators {
public static void main(String[] args)
{
int a = 20, b = 10, c = 0, d = 20, e = 40, f = 30;
// precedence rules for arithmetic operators.
// (* = / = %) > (+ = -)
// prints a+(b/d)
System.out.println("a+b/d = " + (a + b / d));
// if same precendence then associative
// rules are followed.
// e/f -> b*d -> a+(b*d) -> a+(b*d)-(e/f)
System.out.println("a+b*d-e/f = "
+ (a + b * d - e / f));
}
}
a+b/d = 20
a+b*d-e/f = 219
做一个编译器:在我们的系统中,编译器使用lex工具在生成令牌时匹配最大的匹配项。如果忽视了这一点,就会产生一些问题。例如,考虑语句a=b+++c;,对于许多读者来说,这可能会创建编译器错误。但是这个语句是绝对正确的,因为lex创建的令牌是a, =, b, ++, +, c。因此,这个语句具有类似的效果,首先将b+c赋值给a,然后递增b。将生成错误,因为生成的标记是a, =, b, ++, ++, +, c。这实际上是一个错误,因为在第二个一元操作数之后没有操作数。
public class operators {
public static void main(String[] args)
{
int a = 20, b = 10, c = 0;
// a=b+++c is compiled as
// b++ +c
// a=b+c then b=b+1
a = b++ + c;
System.out.println("Value of a(b+c), "
+ " b(b+1), c = "
+ a + ", " + b
+ ", " + c);
// a=b+++++c is compiled as
// b++ ++ +c
// which gives error.
// a=b+++++c;
// System.out.println(b+++++c);
}
}
输出:
Value of a(b+c), b(b+1), c = 10, 11, 0
Using + over():当在system.out.println()中使用+操作符时,确保使用圆括号进行加法。如果我们在做加法之前写了一些东西,那么字符串加法就会发生,也就是说,加法的结合性是从左到右的,因此整数先添加到字符串中产生字符串,而字符串对象在使用+时连接,因此它可能会产生不想要的结果。
public class operators {
public static void main(String[] args)
{
int x = 5, y = 8;
// concatenates x and y as
// first x is added to "concatenation (x+y) = "
// producing "concatenation (x+y) = 5"
// and then 8 is further concatenated.
System.out.println("Concatenation (x+y)= "
+ x + y);
// addition of x and y
System.out.println("Addition (x+y) = "
+ (x + y));
}
}
输出
Concatenation (x+y)= 58
Addition (x+y) = 13
参考文献: 官方Java文档 - https://docs.oracle.com/javase/tutorial/java/nutsandbolts/op1.html
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。