指令是文档对象模型(DOM)中的标记。指令可与任何控制器或HTML标记一起使用, 这将告诉编译器预期的确切操作或行为。存在一些预定义的指令, 但是如果开发人员希望他可以创建新指令(自定义指令)。
下表列出了重要的内置AngularJS指令。
指令 | 描述 |
---|---|
ng-app | AngularJS应用程序的启动。 |
ng-init | 用于初始化变量 |
ng-model | ng-model用于绑定到HTML控件 |
ng-controller | 将控制器附加到视图 |
ng-bind | 将值与HTML元素绑定 |
ng-repeat | 对指定集合中的每个项目重复一次HTML模板。 |
ng-show | 显示或隐藏关联的HTML元素 |
ng-readonly | 将HTML元素设置为只读 |
ng-disabled | 用于动态禁用或启用按钮 |
ng-if | 删除或重新创建HTML元素 |
ng-click | 自定义点击步骤 |
ng-app:
ng-app指令
AngularJS中的"语言"用于定义AngularJS应用程序的根元素。该指令在页面加载时自动初始化AngularJS应用程序。它可以用来在AngularJS应用程序中加载各种模块。
例子:
本示例使用ng-app指令定义默认的AngularJS应用程序。
<html>
<head>
<title>AngularJS ng-app Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body style= "text-align:center" >
<h2 style = "color:green" >ng-app directive</h2>
<div ng-app= "" ng-init= "name='lsbin'" >
<p>{{ name }} is the portal for geeks.</p>
</div>
</body>
</html>
输出如下:
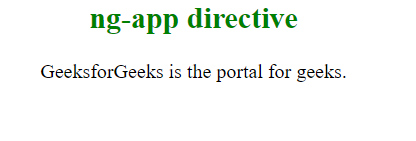
ng-init:
ng-init指令用于初始化AngularJS应用程序数据。它为AngularJS应用程序定义了初始值, 并为变量分配了值。
ng-init指令定义AngularJS应用程序的初始值和变量。
例子:
在此示例中, 我们初始化一个字符串数组。
<html>
<script src= "https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/
angular.min.js" ></script>
<head>
<title>AngularJS ng-init Directive</title>
</head>
<body>
<h1 style = "color:green" >lsbin
<h2>ng-init directive</h2>
<div ng-app= "" ng-init= "sort=['quick sort', 'merge sort', 'bubble sort']" >
Sorting techniques:
<ul>
<li>{{ sort[0] }} </li>
<li>{{ sort[1] }} </li>
<li>{{ sort[2] }} </li>
</ul>
</div>
</body>
</html>
输出如下:
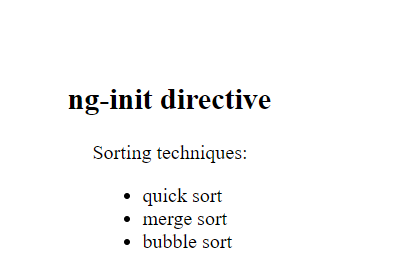
ng-model:
ngModel是一个指令, 它绑定输入, 选择和文本区域, 并将所需的用户值存储在变量中, 并且我们可以在需要该值时使用该变量。
在验证过程中也以某种形式使用它。
例子:
<!DOCTYPE html>
<html>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
<style>
.column {
float: left;
text-align: left;
width: 49%;
}
.row {
content: "" ;
display: table;
}
</style>
<body ng-app= "myApp"
ng-controller= "myController" >
<h4>Input Box-</h4>
<div class= "row" >
<div class= "column" >
Name-
<input type= "text"
ng-model= "name" >
<pre> {{ name }} </pre> Checkbox-
<input type= "checkbox"
ng-model= "check" >
<pre> {{ check }} </pre> Radiobox-
<input type= "radio"
ng-model= "choice" >
<pre> {{ choice }} </pre> Number-
<input type= "number"
ng-model= "num" >
<pre> {{ num }} </pre> Email-
<input type= "email"
ng-model= "mail" >
<pre> {{ mail }} </pre> Url-
<input type= "url"
ng-model= "url" >
<pre> {{ url }} </pre>
</div>
<div class= "column" >
Date:
<input type= "date" ng-model= "date1" (change)= "log(date1)" >
<pre> Todays date:{{ date1+1 }}</pre> Datetime-local-
<input type= "datetime-local" ng-model= "date2" >
<pre> {{ date2+1 }} </pre> Time-
<input type= "time" ng-model= "time1" >
<pre> {{ time1+1 }} </pre> Month-
<input type= "month" ng-model= "mon" >
<pre> {{ mon+1 }} </pre> Week-
<input type= "week" ng-model= "we" >
<pre> {{ we+1 }} </pre>
</div>
</div>
</body>
<script>
var app = angular.module( 'myApp' , []);
app.controller( 'myController' , function ($scope) {
$scope.name = "Hello Geeks!" ;
$scope.check = "" ;
$scope.rad = "" ;
$scope.num = "" ;
$scope.mail = "" ;
$scope.url = "" ;
$scope.date1 = "" ;
$scope.date2 = "" ;
$scope.time1 = "" ;
$scope.mon = "" ;
$scope.we = "" ;
$scope.choice = "" ;
$scope.c = function () {
$scope.choice = true ;
};
});
</script>
</html>
输出如下:
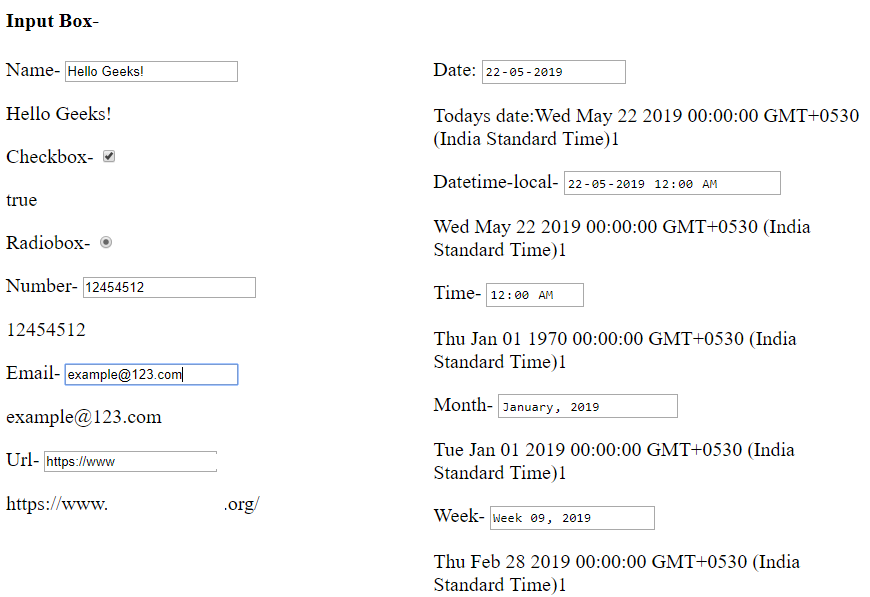
ng-controller:
AngularJS中的ng-controller指令用于将控制器添加到应用程序。它可用于添加可以在某些事件(例如click等)上调用的方法, 函数和变量, 以执行某些操作。
例子:
<!DOCTYPE html>
<html>
<head>
<title>ng-controller Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.4.2/angular.min.js" >
</script>
</head>
<body ng-app= "app" style= "text-align:center" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-controller Directive</h2><br>
<div ng-controller= "geek" >
Name: <input class= "form-control" type= "text"
ng-model= "name" >
<br><br>
You entered: <b><span>{{name}}</span></b>
</div>
<script>
var app = angular.module( 'app' , []);
app.controller( 'geek' , function ($scope) {
$scope.name = "lsbin" ;
});
</script>
</body>
</html>
输出如下:
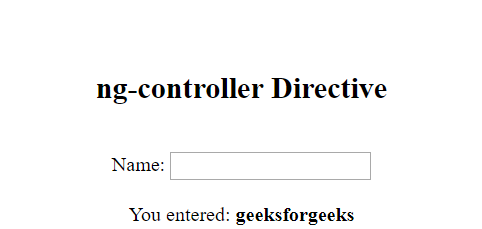
ng-bind:
AngularJS中的ng-bind指令用于将任何特定HTML元素的文本内容绑定/替换为在给定表达式中输入的值。每当表达式的值在以下位置更改时, 指定的HTML内容的值就会更新ng-bind指令
<!DOCTYPE html>
<html>
<head>
<title>ng-checked Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body ng-app= "gfg" style= "text-align:center" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-bind Directive</h2>
<div ng-controller= "app" >
num1: <input type= "number" ng-model= "num1"
ng-change= "product()" />
<br><br>
num2: <input type= "number" ng-model= "num2"
ng-change= "product()" />
<br><br>
<b>Product:</b> <span ng-bind= "result" ></span>
</div>
<script>
var app = angular.module( "gfg" , []);
app.controller( 'app' , [ '$scope' , function ($app) {
$app.num1 = 1;
$app.num2 = 1;
$app.product = function () {
$app.result = ($app.num1 * $app.num2);
}
}]);
</script>
</body>
</html>
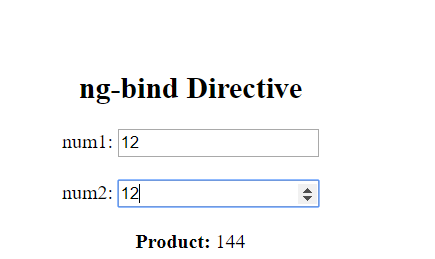
ng-repeat:
Angular-JS ng-repeat指令是一种方便的工具, 可以将一组HTML代码重复多次或对一组项目中的每个项目重复一次。 ng-repeat主要用于数组和对象。
ng-repeat类似于我们使用C, C ++或其他语言编写的循环, 但是从技术上讲, 它为我们正在访问的集合中的每个元素实例化一个模板(通常是一组HTML结构)。 Angular维护$ index变量作为当前正在访问的元素的键, 用户也可以访问此变量。
例子:
为该应用创建一个app.js文件。
var app = angular.module( 'myApp' , []);
app.controller( 'MainCtrl' , function ($scope){
$scope.names = [ 'Adam' , 'Steve' , 'George' , 'James' , 'Armin' ];
console.log($scope.names);
});
第1行-创建了一个名为“myApp”的应用模块,没有依赖项。
Line 3-我们应用程序的主控制器。
第4行-字符串数组“名称”。
创建index.html页面
<!DOCTYPE html>
<html ng-app= "myApp" >
<head>
<title>Angular ng-repeat</title>
<script> type= "text/javascript" src= "jquery-3.2.1.min.js" >
</script>
<script> type= "text/javascript" src= "angular.js" ></script>
<script> type= "text/javascript" src= "app.js" ></script>
</head>
<body ng-controller= "MainCtrl" >
<h2>Here is the name list</h2>
<ul>
<li ng-repeat= "name in names" >
{{name}}
</li>
</ul>
</body>
</html>
第5行-包括所有的依赖,比如jquery、angular-js和app.js文件
12行-使用ng-repeat指令一次从names数组中获取一个名称并显示它。
输出如下:
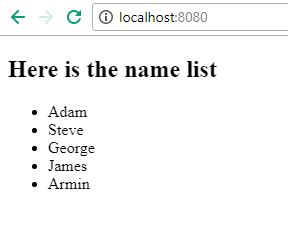
ng-show:
AngluarJS中的ng-show指令用于显示或隐藏指定的HTML元素。如果ng-show属性中的给定表达式为true, 则将显示HTML元素, 否则将隐藏HTML元素。所有HTML元素都支持它。
范例1:
此示例使用ng-show Directive选中复选框后显示HTML元素。
<!DOCTYPE html>
<html>
<head>
<title>ng-show Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body>
<div ng-app= "app" ng-controller= "geek" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-show Directive</h2>
<input id= "chshow" type= "checkbox" ng-model= "show" />
<label for = "chshow" >
Show Paragraph
</label>
<p ng-show= "show" style= "background: green; color: white;
font-size: 14px; width:35%; padding: 10px;" >
Show this paragraph using ng-show
</p>
</div>
<script>
var myapp = angular.module( "app" , []);
myapp.controller( "geek" , function ($scope) {
$scope.show = false ;
});
</script>
</body>
</html>
输出如下:
在选中复选框之前:
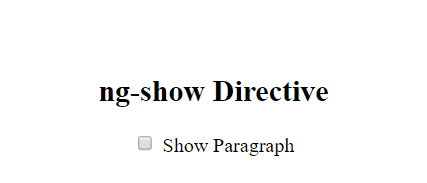
选中复选框后:
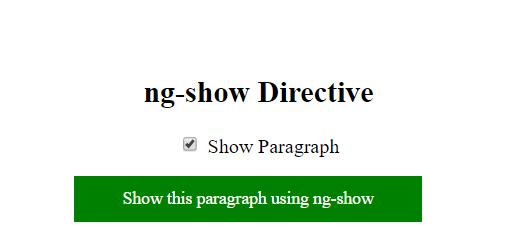
ng-readonly:
AngularJS中的ng-readonly指令用于指定HTML元素的readonly属性。仅当ng-readonly指令中的表达式返回true时, HTML元素才是只读的。
例子:
本示例使用ng-readonly指令启用只读属性。
<!DOCTYPE html>
<html>
<head>
<title>ng-readonly Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.4.2/angular.min.js" >
</script>
</head>
<body ng-app style= "text-align:center" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-readonly Directive</h2>
<div>
<label>Check to make month readonly: <input type= "checkbox"
ng-model= "open" ></label>
<br><br>
Input Month:<input ng-readonly= "open" type= "month"
ng-model= "month" >
</div>
</body>
</html>
输出如下:
在选中复选框之前:
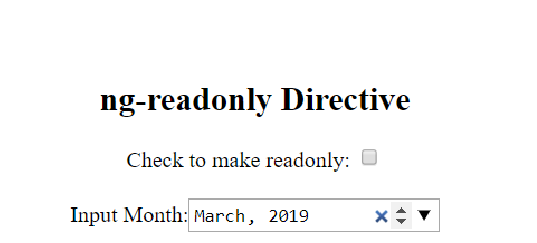
选中复选框后:
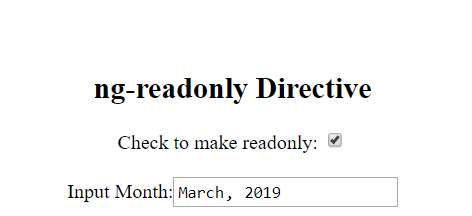
ng-disabled:
AngularJS中的ng-disabled指令用于启用或禁用HTML元素。如果ng-disabled属性内的表达式返回true, 则form字段将被禁用, 反之亦然。它通常应用于表单字段(输入, 选择, 按钮等)。
范例1:
本示例使用ng-disabled指令禁用按钮。
<!DOCTYPE html>
<html>
<head>
<title>ng-disabled Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body ng-app= "app" style= "text-align:center" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-disabled Directive</h2>
<div ng-controller= "app" ng-init= "disable=false" >
<button ng-click= "geek(disable)" ng-disabled= "disable" >
Click to Disable
</button>
<button ng-click= "geek(disable)" ng-show= "disable" >
Click to Enable
</button>
</div>
<script>
var app = angular.module( "app" , []);
app.controller( 'app' , [ '$scope' , function ($app) {
$app.geek = function (disable) {
$app.disable = !disable;
}
}]);
</script>
</body>
</html>
输出如下:
在单击按钮之前:
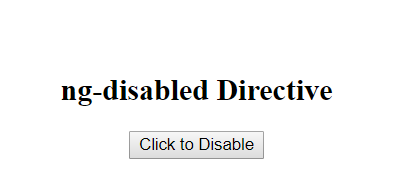
单击按钮后:
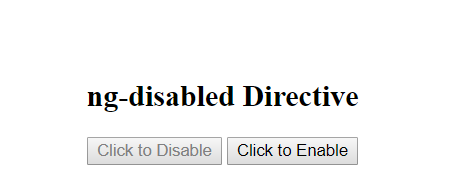
ng-if:
AngularJS中的ng-if指令用于根据表达式删除或重新创建HTML元素的一部分。 ng-if与ng-hide有所不同, 因为它可以完全删除DOM中的元素, 而不仅仅是隐藏元素的显示。如果其中的表达式为false, 则删除该元素;如果为true, 则将该元素添加到DOM。
例子:
本示例在单击按钮后更改内容。
<!DOCTYPE html>
<html>
<head>
<title>ng-hide Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body ng-app= "geek" style= "text-align:center" >
<h1 style= "color:green" >
lsbin
</h1>
<h2>ng- if Directive</h2>
<div ng-controller= "app as vm" >
<div ng- if = "!vm.IsShow" >
<input type= "button" class= "btn btn-primary"
ng-click= "vm.IsShow=!vm.IsShow"
value= "Sign in" >
<p>Click to Sign in </p>
</div>
<div ng- if = "vm.IsShow" >
<button class= "btn btn-primary"
ng-click= "vm.IsShow=!vm.IsShow" >
Sign out
</button>
<p>
lsbin is the computer
science portal for geeks.
</p>
</div>
</div>
<script>
var app = angular.module( "geek" , []);
app.controller( 'app' , [ '$scope' , function ($scope) {
var vm = this ;
}]);
</script>
</body>
</html>
输出如下:
在点击按钮之前:
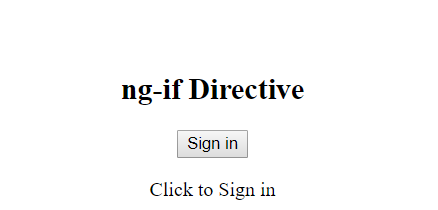
单击按钮后:
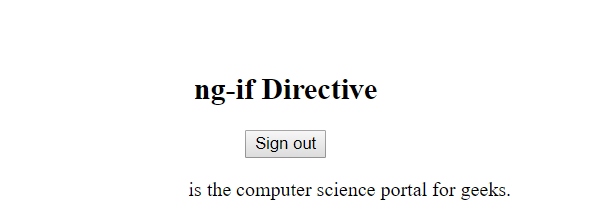
ng-click:
AngluarJS中的ng-click指令用于单击元素时应用自定义行为。它可以用于显示/隐藏某些元素, 或者在单击按钮时可以弹出警报。
例子:
本示例使用ng-click指令在单击元素后显示警报消息。
<!DOCTYPE html>
<html>
<head>
<title>ng-click Directive</title>
<script src=
"https://ajax.googleapis.com/ajax/libs/angularjs/1.6.9/angular.min.js" >
</script>
</head>
<body ng-app= "geek" style= "text-align:center" >
<h1 style= "color:green" >lsbin</h1>
<h2>ng-click Directive</h2>
<div ng-controller= "app" >
<button>
<a href= "" ng-click= "alert()" >
Click Here
</a>
</button>
</div>
<script>
var app = angular.module( "geek" , []);
app.controller( 'app' , [ '$scope' , function ($app) {
$app.alert = function () {
alert( "This is an example of ng-click" );
}
}]);
</script>
</body>
</html>
输出如下:
在单击按钮之前:
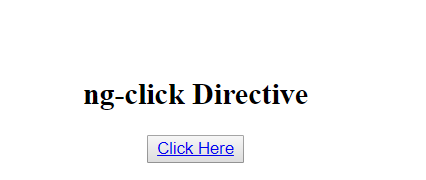
单击按钮后:
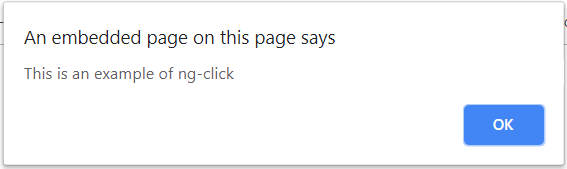