方法1:使用Node.contains()方法
Node.contains()方法用于检查给定节点是否是任何级别的另一个节点的后代。后代可以直接是子元素的父元素, 也可以是子元素的父元素。它返回结果的布尔值。
在父元素上使用此方法, 并且在该方法中传递的参数是要检查的子元素。如果子元素是父元素的后代, 则返回true。这意味着该元素是父级的子级。
语法如下:
function checkParent(parent, child) {
if (parent.contains(child))
return true ;
return false ;
}
例子:
< html >
< head >
< title >
How to Check if an element is a
child of a parent using JavaScript?
</ title >
< style >
.parent, .child, .non-child {
border: 2px solid;
padding: 5px;
margin: 5px;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >lsbin</ h1 >
< b >
How to Check if an element is
a child of a parent using JavaScript?
</ b >
< div class = "parent" >This is the parent div.
< div class = "child" >This is the child div.
</ div >
</ div >
< div class = "non-child" >
This is outside the parent div.
</ div >
< p >Click on the button to check if the
elements are child of a parent.</ p >
< p >Child has parent:
< span class = "output-child" ></ span >
</ p >
< p >Non-Child has parent:
< span class = "output-non-child" ></ span >
</ p >
< button onclick = "checkElements()" >
Check elements
</ button >
< script >
function checkParent(parent, child) {
if (parent.contains(child))
return true;
return false;
}
function checkElements() {
parent = document.querySelector('.parent');
child = document.querySelector('.child');
non_child = document.querySelector('.non-child');
output_child = checkParent(parent, child);
output_non_child = checkParent(parent, non_child);
document.querySelector('.output-child').textContent =
output_child;
document.querySelector('.output-non-child').textContent =
output_non_child;
}
</ script >
</ body >
</ html >
输出如下:
在单击按钮之前:
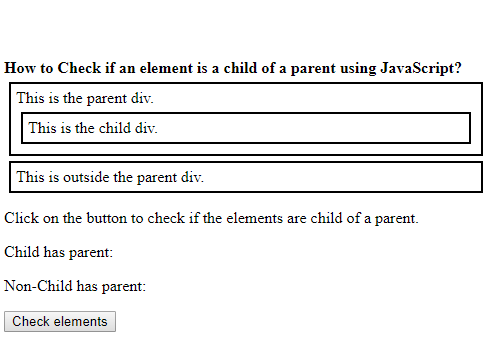
单击按钮后:
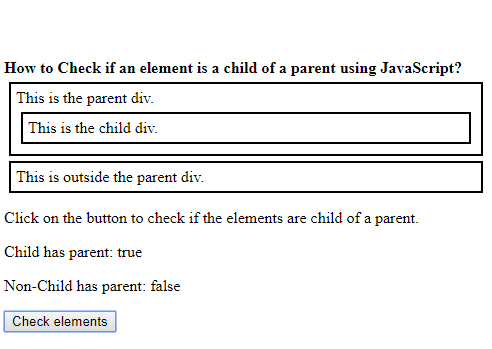
方法2:遍历给定子元素的父元素
通过不断地逐个元素地循环访问元素的父元素, 可以检查子元素是否具有给定的父元素。通过访问以下节点找到每个节点的父节点parentNode返回父节点(如果有)的属性。
使用while循环, 直到找到所需的父级或不再存在任何父级元素为止。在此循环内, 每次迭代中都会找到每个元素的父节点。如果父节点在任何迭代中都与给定节点匹配, 则意味着该元素是父节点的子节点。
语法如下:
function checkParent(parent, child) {
let node = child.parentNode;
// keep iterating unless null
while (node != null ) {
if (node == parent) {
return true ;
}
node = node.parentNode;
}
return false ;
}
例子:
< html >
< head >
< title >
How to Check if an element is a
child of a parent using JavaScript?
</ title >
< style >
.parent, .child, .non-child {
border: 2px solid;
padding: 5px;
margin: 5px;
}
</ style >
</ head >
< body >
< h1 style = "color: green" >lsbin</ h1 >
< b >
How to Check if an element is
a child of a parent using JavaScript?
</ b >
< div class = "parent" >This is the parent div.
< div class = "child" >This is the child div.
</ div >
</ div >
< div class = "non-child" >
This is outside the parent div.
</ div >
< p >Click on the button to check if
the elements are child of a parent.</ p >
< p >Child has parent:
< span class = "output-child" ></ span >
</ p >
< p >Non-Child has parent:
< span class = "output-non-child" ></ span >
</ p >
< button onclick = "checkElements()" >
Check elements
</ button >
< script >
function checkParent(parent, child) {
let node = child.parentNode;
// keep iterating unless null
while (node != null) {
if (node == parent) {
return true;
}
node = node.parentNode;
}
return false;
}
function checkElements() {
parent = document.querySelector('.parent');
child = document.querySelector('.child');
non_child = document.querySelector('.non-child');
output_child = checkParent(parent, child);
output_non_child = checkParent(parent, non_child);
document.querySelector('.output-child').textContent =
output_child;
document.querySelector('.output-non-child').textContent =
output_non_child;
}
</ script >
</ body >
</ html >
输出如下:
在单击按钮之前:
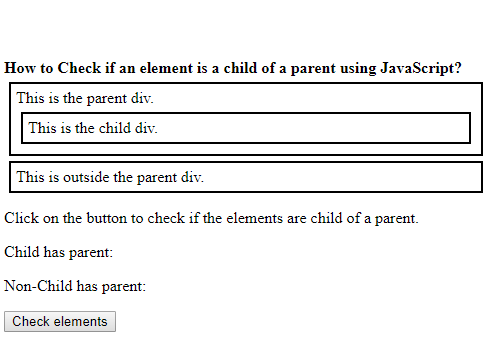
单击按钮后:
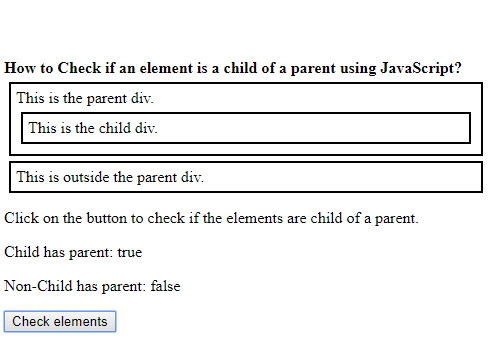