本文概述
Luhn算法,也称为模数10或mod 10算法,是一种简单的校验和公式,用于验证各种识别号码,如信用卡号码、IMEI号码、加拿大社会保险号码。Luhn公式是一群数学家在20世纪60年代后期提出的。此后不久,信用卡公司采用了它。因为这个算法是公开的,所以任何人都可以使用它。大多数信用卡和许多政 府识别号码使用该算法作为一种简单的方法,以区别输入错误或不正确的号码。它的设计是为了防止意外错误,而不是恶意攻击。
Luhn算法涉及的步骤
让我们通过一个例子来了解算法:
考虑帐户号码"79927398713"的示例
第1步–从最右边的数字开始, 将第二个数字的值加倍,
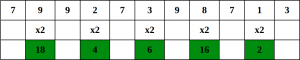
第2步–如果数字加倍导致两位数字, 即大于9(例如6×2 = 12), 则将乘积相加(例如12:1 + 2 = 3、15:1 + 5 = 6), 获得一个数字位数。
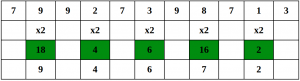
第三步–现在取所有数字的总和。
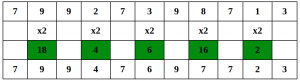
步骤4–如果总模10等于0(如果总以零结尾), 则该数字根据Luhn公式有效;否则无效。
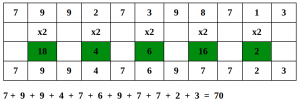
由于总和为70(是10的倍数), 因此该帐号可能有效。
这个想法很简单, 我们从头到尾遍历。对于第二个数字, 我们在添加之前将其加倍。我们将加倍后获得的数字加两位数。
C++
// C++ program to implement Luhn algorithm
#include <bits/stdc++.h>
using namespace std;
// Returns true if given card number is valid
bool checkLuhn( const string& cardNo)
{
int nDigits = cardNo.length();
int nSum = 0, isSecond = false ;
for ( int i = nDigits - 1; i >= 0; i--) {
int d = cardNo[i] - '0' ;
if (isSecond == true )
d = d * 2;
// We add two digits to handle
// cases that make two digits after
// doubling
nSum += d / 10;
nSum += d % 10;
isSecond = !isSecond;
}
return (nSum % 10 == 0);
}
// Driver code
int main()
{
string cardNo = "79927398713" ;
if (checkLuhn(cardNo))
printf ( "This is a valid card" );
else
printf ( "This is not a valid card" );
return 0;
}
Java
// Java program to implement
// Luhn algorithm
import java.io.*;
class GFG {
// Returns true if given
// card number is valid
static boolean checkLuhn(String cardNo)
{
int nDigits = cardNo.length();
int nSum = 0 ;
boolean isSecond = false ;
for ( int i = nDigits - 1 ; i >= 0 ; i--)
{
int d = cardNo.charAt(i) - '0' ;
if (isSecond == true )
d = d * 2 ;
// We add two digits to handle
// cases that make two digits
// after doubling
nSum += d / 10 ;
nSum += d % 10 ;
isSecond = !isSecond;
}
return (nSum % 10 == 0 );
}
// Driver code
static public void main (String[] args)
{
String cardNo = "79927398713" ;
if (checkLuhn(cardNo))
System.out.println( "This is a valid card" );
else
System.out.println( "This is not a valid card" );
}
}
// This Code is contributed by vt_m.
Python3
# Python3 program to implement
# Luhn algorithm
# Returns true if given card
# number is valid
def checkLuhn(cardNo):
nDigits = len (cardNo)
nSum = 0
isSecond = False
for i in range (nDigits - 1 , - 1 , - 1 ):
d = ord (cardNo[i]) - ord ( '0' )
if (isSecond = = True ):
d = d * 2
# We add two digits to handle
# cases that make two digits after
# doubling
nSum + = d / / 10
nSum + = d % 10
isSecond = not isSecond
if (nSum % 10 = = 0 ):
return True
else :
return False
# Driver code
if __name__ = = "__main__" :
cardNo = "79927398713"
if (checkLuhn(cardNo)):
print ( "This is a valid card" )
else :
print ( "This is not a valid card" )
# This code is contributed by rutvik_56
C#
// C# program to implement
// Luhn algorithm
using System;
class GFG {
// Returns true if given
// card number is valid
static bool checkLuhn(String cardNo)
{
int nDigits = cardNo.Length;
int nSum = 0;
bool isSecond = false ;
for ( int i = nDigits - 1; i >= 0; i--)
{
int d = cardNo[i] - '0' ;
if (isSecond == true )
d = d * 2;
// We add two digits to handle
// cases that make two digits
// after doubling
nSum += d / 10;
nSum += d % 10;
isSecond = !isSecond;
}
return (nSum % 10 == 0);
}
// Driver code
static public void Main()
{
String cardNo = "79927398713" ;
if (checkLuhn(cardNo))
Console.WriteLine( "This is a valid card" );
else
Console.WriteLine( "This is not a valid card" );
}
}
// This Code is contributed by vt_m.
输出如下:
This is a valid card
Luhn算法检测任何一位数字错误, 以及几乎所有相邻数字的换位。