方法是执行某些特定任务并将结果返回给调用者的语句的集合。一个方法可以执行一些特定的任务而不返回任何东西。方法允许我们重用代码而无需重新输入代码。在Java中,每个方法都必须是某些类的一部分,这些类不同于C、c++和Python等语言。
方法可以节省时间,并帮助我们重用代码而无需重新输入代码。
方法声明
通常, 方法声明包含六个部分:
- 修饰符-:定义访问类型方法的含义, 即可以从你的应用程序中访问的位置。在Java中, 有4种类型的访问说明符。
- public:可在你的应用程序的所有类中访问。
- protected:在定义它的类及其内部都可以访问子类
- private:只能在定义它的类中访问。
- default(在不使用任何修饰符的情况下进行声明/定义):可在定义其类的相同类和包中访问。
- 返回类型:方法返回的值的数据类型;如果不返回值, 则返回void。
- 方法名称:字段名称的规则也适用于方法名称, 但是约定有所不同。
- 参数列表:在括号内定义了输入参数的逗号分隔列表, 并以其数据类型开头。如果没有参数, 则必须使用空括号()。
- 异常列表:该方法期望的异常可能引发, 你可以指定这些异常。
- 方法主体:用大括号括起来。你需要执行代码才能执行预期的操作。
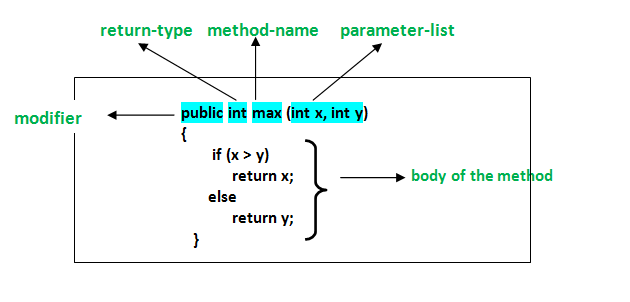
方法签名:它由方法名称和参数列表(参数数量, 参数类型和参数顺序)组成。返回类型和异常不视为其中的一部分。
以上函数的方法签名:
max(int x, int y)
如何命名一个方法?:方法名通常是一个单词,它应该是一个小写的动词或多个单词,以一个小写的动词开头,后面是形容词、名词.....在第一个单词之后,每个单词的第一个字母都要大写。例如,findSum,
computeMax, setX和getX
通常, 方法在定义该方法的类中具有唯一的名称, 但有时某个方法可能与在同一类中的其他方法名称具有相同的名称。Java中允许方法重载.
调用方法
需要使用该方法的功能来调用该方法。调用方法可能有三种情况:
在以下情况下, 方法将返回调用它的代码:
- 它完成了方法中的所有语句
- 它到达一个返回语句
- 引发异常
// Program to illustrate methodsin java
import java.io.*;
class Addition {
int sum = 0 ;
public int addTwoInt( int a, int b){
// adding two integer value.
sum = a + b;
//returning summation of two values.
return sum;
}
}
class GFG {
public static void main (String[] args) {
// creating an instance of Addition class
Addition add = new Addition();
// calling addTwoInt() method to add two integer using instance created
// in above step.
int s = add.addTwoInt( 1 , 2 );
System.out.println( "Sum of two integer values :" + s);
}
}
输出:
Sum of two integer values :3
请参见以下示例, 以详细了解方法调用:
// Java program to illustrate different ways of calling a method
import java.io.*;
class Test
{
public static int i = 0 ;
// constructor of class which counts
//the number of the objects of the class.
Test()
{
i++;
}
// static method is used to access static members of the class
// and for getting total no of objects
// of the same class created so far
public static int get()
{
// statements to be executed....
return i;
}
// Instance method calling object directly
// that is created inside another class 'GFG'.
// Can also be called by object directly created in the same class
// and from another method defined in the same class
// and return integer value as return type is int.
public int m1()
{
System.out.println( "Inside the method m1 by object of GFG class" );
// calling m2() method within the same class.
this .m2();
// statements to be executed if any
return 1 ;
}
// It doesn't return anything as
// return type is 'void'.
public void m2()
{
System.out.println( "In method m2 came from method m1" );
}
}
class GFG
{
public static void main(String[] args)
{
// Creating an instance of the class
Test obj = new Test();
// Calling the m1() method by the object created in above step.
int i = obj.m1();
System.out.println( "Control returned after method m1 :" + i);
// Call m2() method
// obj.m2();
int no_of_objects = Test.get();
System.out.print( "No of instances created till now : " );
System.out.println(no_of_objects);
}
}
输出:
Inside the method m1 by object of GFG class
In method m2 came from method m1
Control returned after method m1 :1
No of instances created till now : 1
以上程序的控制流程:
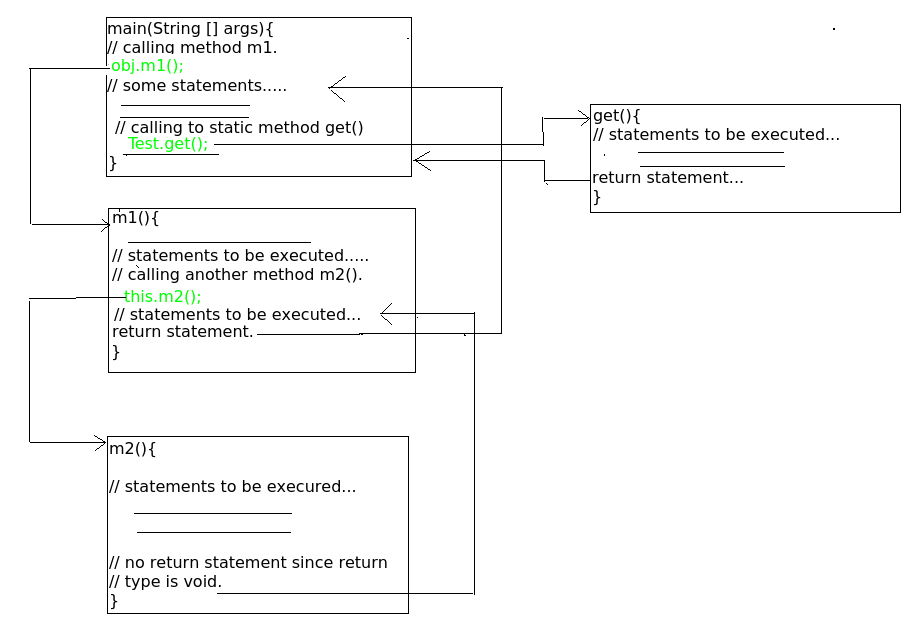
方法调用的内存分配
方法调用是通过堆栈实现的。每当在堆栈区域内创建一个被称为方法的堆栈框架时, 之后将传递给该参数的参数以及此被调用方法将要返回的局部变量和值存储在此堆栈框架中, 并且当被调用方法的执行完成时, 分配的堆栈帧将被删除。有一个堆栈指针寄存器, 用于跟踪堆栈顶部, 并相应地对其进行了调整。
相关文章:
- Java严格按价值传递
- Java中的方法重载和Null错误
- 我们可以重载或重写Java中的静态方法吗?
- Java测验
参考:
https://docs.oracle.com/javase/tutorial/java/javaOO/methods.html
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。