假设我们给出了一个String, 其中指定了一些范围, 并且我们必须将示例中给出的指定范围之间的数字放置在指定位置:
例子:
Input : string x = "1-5, 8, 11-14, 18, 20, 26-29"
Output : string y = "1, 2, 3, 4, 5, 8, 11, 12, 13, 14, 18, 20, 26, 27, 28, 29"
方法:要解决上述问题, 我们可以采用以下方法:
首先, 我们必须将String拆分为String []数组。我们必须在找到符号的地方拆分字符串。
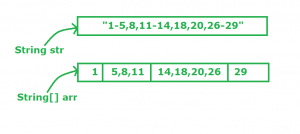
现在, 我们有了带有元素的String []数组。现在我们只需要转到String []数组的第一个索引的最后一个元素(即1)和先前的索引第一个元素(即5)。
之后, 借助for循环, 我们可以添加1到5之间的数字并将其存储在String变量中。
以上过程一直持续到String []数组的长度。
注意:借助Collections和各种实用程序方法, 我们可以轻松解决问题, 但是Collections概念并不是明智的选择权。如果我们通过Collections, 会降低性能并增加时间复杂度。在下面的程序中, 我们明确定义了自己的拆分方法和逻辑。
// Java code to demonstrate expansion
// of string
public class StringExpand {
static String[] split(String st)
{
// Count how many words in our string
// Irrespective of spaces
int wc = countWords(st);
String w[] = new String[wc];
char [] c = st.toCharArray();
int k = 0 ;
for ( int i = 0 ; i < c.length; i++) {
String s = "" ;
// Whenever we found an non-space character
while (i < c.length && c[i] != ' ' ) {
// Concat with the String s
// Increment the value of i
s = s + c[i];
i++;
}
// If the String is not empty
if (s.length() != 0 ) {
// Add the String to the String[]
// array
w[k] = s;
k++;
}
}
// Returning the String[] array
return w;
}
static int countWords(String str)
{
int count = 0 ;
for ( int i = 0 ; i < str.length(); i++) {
// The below condition to check
// whether the first character is
// space or not
if (i == 0 && str.charAt(i) != ' ' || str.charAt(i) != ' ' && str.charAt(i - 1 ) == ' ' ) {
count++;
}
}
return count;
}
public static void expand(String s)
{
String p = s;
String[] arr = p.split( "\\-" );
String k = "" ;
for ( int i = 0 ; i < arr.length; i++) {
if (i != arr.length - 1 ) {
String[] arr1 = arr[i].split( ", " );
String[] arr2 = arr[i + 1 ].split( ", " );
int a = Integer.parseInt(arr1[arr1.length - 1 ]);
int b = Integer.parseInt(arr2[ 0 ]);
for ( int j = a + 1 ; j < b; j++) {
arr[i] = arr[i] + ", " + j;
}
}
if (k != "" )
k = k + ", " + arr[i];
else
k = k + arr[i];
}
System.out.println(k);
}
// Driver code
public static void main(String[] args)
{
String s = "1-5, 8, 11-14, 18, 20, 26-29" ;
expand(s);
}
}
输出如下:
1, 2, 3, 4, 5, 8, 11, 12, 13, 14, 18, 20, 26, 27, 28, 29
实施更简单:
public class Solution {
public static void expand(String word)
{
StringBuilder sb = new StringBuilder();
// Get all intervals
String[] strArr = word.split( ", " );
// Traverse through every interval
for ( int i = 0 ; i < strArr.length; i++) {
// Get lower and upper
String[] a = strArr[i].split( "-" );
if (a.length == 2 ) {
int low = Integer.parseInt(a[ 0 ]);
int high = Integer.parseInt(a[a.length - 1 ]);
// Append all numbers
while (low <= high) {
sb.append(low + " " );
low++;
}
}
else {
sb.append(strArr[i] + " " );
}
}
System.out.println(sb.toString());
}
public static void main(String args[])
{
String s = "1-5, 8, 11-14, 18, 20, 26-29" ;
expand(s);
}
}
输出如下:
1 2 3 4 5 8 11 12 13 14 18 20 26 27 28 29