给定一个JavaScript日期, 任务是在JavaScript的帮助下将其舍入到5分钟。下面讨论了两种方法:
方法1:在这种方法中, 两个选项都可用于舍入或舍入日期对象。本示例使用基本Math.floor()函数和Math.ceil()函数执行操作。
例子:
本示例实现了上述方法。
<!DOCTYPE html>
< html >
< head >
< title >
Round off a Date Object to 5
minutes in JavaScript.
</ title >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
lsbin
</ h1 >
< p id = "gfg" style="font-size: 20px;
font-weight: bold">
</ p >
< button onclick = "GFG_Fun1();" >
Round Down
</ button >
< button onclick = "GFG_Fun2();" >
Round Up
</ button >
< p id = "geeks" style="font-size: 26px;
font-weight: bold;color: green;">
</ p >
< script >
var up = document.getElementById('gfg');
var down = document.getElementById('geeks');
var date = new Date();
up.innerHTML = "Click on the button to "
+ "round the date as specified."
+ "< br >< br >Date - " + date;
function GFG_Fun1() {
// ms in 5 minutes.
var coff = 1000 * 60 * 5;
down.innerHTML = new Date(
Math.floor(date / coff) * coff);
}
function GFG_Fun2() {
// ms in 5 minutes.
var coff = 1000 * 60 * 5;
down.innerHTML = new Date(
Math.ceil(date / coff) * coff);
}
</ script >
</ body >
</ html >
输出如下:
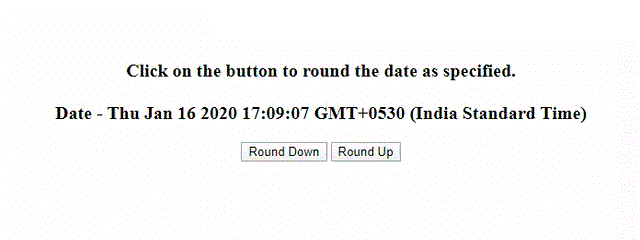
方法二:本示例使用基本Math.round()函数执行操作。计算5分钟内的毫秒数, 将日期对象除以毫秒数, 得到取整值, 然后再次乘以毫秒数。
例子:
本示例实现了上述方法。
<!DOCTYPE html>
< html >
< head >
< title >
Round off a Date Object to 5
minutes in JavaScript.
</ title >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >
lsbin
</ h1 >
< p id = "GFG_UP" style =
"font-size: 20px;font-weight: bold" >
</ p >
< button onclick = "GFG_Fun();" >
click here
</ button >
< p id = "GFG_DOWN" style = "font-size: 26px;
font-weight: bold;color: green;">
</ p >
< script >
var up = document.getElementById('GFG_UP');
var down = document.getElementById('GFG_DOWN');
var date = new Date();
up.innerHTML = "Click on the button to "
+ "round the date as specified."
+ "< br >< br >Date - " + date;
function GFG_Fun() {
// ms in 5 minutes.
var coff = 1000 * 60 * 5;
down.innerHTML = new Date(Math.round(
date.getTime() / coff) * coff);
}
</ script >
</ body >
</ html >
输出如下:
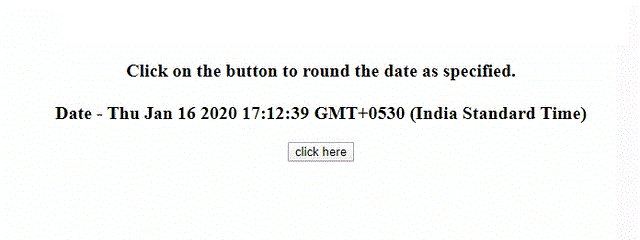