编写函数以检查两个给定的字符串是否为字谜彼此之间。
字符串的字谜是另一个包含相同字符的字符串, 只有字符顺序可以不同。
例如, " abcd"和" dabc"是彼此的Anagram。
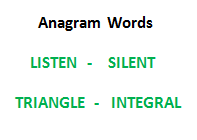
推荐:请尝试以下方法{IDE}首先, 在继续解决方案之前。
方法: 哈希图通过将每个字符串的字符映射到单独的哈希图并将它们进行比较, 还可以用于查找两个给定的字符串是否为字谜。
实现
// Java code to check whether two strings
// are Anagram or not using HashMap
import java.io.*;
import java.util.*;
class GFG {
// Function to check whether two strings
// are an anagram of each other
static boolean areAnagram(String str1, String str2)
{
HashMap<Character, Integer> hmap1
= new HashMap<Character, Integer>();
HashMap<Character, Integer> hmap2
= new HashMap<Character, Integer>();
char arr1[] = str1.toCharArray();
char arr2[] = str2.toCharArray();
// Mapping first string
for ( int i = 0 ; i < arr1.length; i++) {
if (hmap1.get(arr1[i]) == null ) {
hmap1.put(arr1[i], 1 );
}
else {
Integer c = ( int )hmap1.get(arr1[i]);
hmap1.put(arr1[i], ++c);
}
}
// Mapping second String
for ( int j = 0 ; j < arr2.length; j++) {
if (hmap2.get(arr2[j]) == null )
hmap2.put(arr2[j], 1 );
else {
Integer d = ( int )hmap2.get(arr2[j]);
hmap2.put(arr2[j], ++d);
}
}
if (hmap1.equals(hmap2))
return true ;
else
return false ;
}
// Test function
public static void test(String str1, String str2)
{
System.out.println( "Strings to be checked are:\n"
+ str1 + "\n" + str2 + "\n" );
// Find the result
if (areAnagram(str1, str2))
System.out.println( "The two strings are "
+ "anagrams of each other\n" );
else
System.out.println( "The two strings are not"
+ " anagrams of each other\n" );
}
// Driver program
public static void main(String args[])
{
// Get the Strings
String str1 = "lsbin" ;
String str2 = "forgeeksgeeks" ;
// Test the Strings
test(str1, str2);
// Get the Strings
str1 = "lsbin" ;
str2 = "geeks" ;
// Test the Strings
test(str1, str2);
}
}
输出如下:
Strings to be checked are:
lsbin
forgeeksgeeks
The two strings are anagrams of each other
Strings to be checked are:
lsbin
geeks
The two strings are not anagram of each other
相关文章: 检查两个字符串是否彼此相似