本文概述
List接口提供了一种存储有序集合的方法。它是采集。它是对象的有序集合, 可以在其中存储重复值。由于List保留插入顺序, 因此它允许元素的位置访问和插入。

宣言:List接口声明为:
公共抽象接口List扩展Collection
列表示例:
// Java program to demonstrate a List
import java.util.*;
public class ListDemo {
public static void main(String[] args)
{
// Creating a list
List<Integer> l1
= new ArrayList<Integer>();
// Adds 1 at 0 index
l1.add( 0 , 1 );
// Adds 2 at 1 index
l1.add( 1 , 2 );
System.out.println(l1);
// Creating another list
List<Integer> l2
= new ArrayList<Integer>();
l2.add( 1 );
l2.add( 2 );
l2.add( 3 );
// Will add list l2 from 1 index
l1.addAll( 1 , l2);
System.out.println(l1);
// Removes element from index 1
l1.remove( 1 );
System.out.println(l1);
// Prints element at index 3
System.out.println(l1.get( 3 ));
// Replace 0th element with 5
l1.set( 0 , 5 );
System.out.println(l1);
}
}
输出如下:
[1, 2]
[1, 1, 2, 3, 2]
[1, 2, 3, 2]
2
[5, 2, 3, 2]
使用执行各种操作列表界面和ArrayList类
由于List是一个接口, 因此只能与实现此接口的类一起使用。现在, 让我们看看如何在列表上执行一些常用的操作。
1.添加元素:为了将元素添加到列表中, 我们可以使用add()方法。重载此方法可根据不同的参数执行多项操作。他们是:
- 添加(对象):此方法用于在列表末尾添加元素。
- add(int index, Object):此方法用于在列表中的特定索引处添加元素。
// Java program to add elements
// to a List
import java.util.*;
public class GFG {
public static void main(String args[])
{
List<String> al = new ArrayList<>();
al.add( "Geeks" );
al.add( "Geeks" );
al.add( 1 , "For" );
System.out.println(al);
}
}
输出如下:
[Geeks, For, Geeks]
2.改变要素:添加元素后, 如果我们希望更改元素, 则可以使用组()方法。由于List已建立索引, 因此我们希望更改的元素由该元素的索引引用。因此, 此方法采用索引和需要在该索引处插入的更新元素。
// Java program to change elements
// in a List
import java.util.*;
public class GFG {
public static void main(String args[])
{
List<String> al = new ArrayList<>();
al.add( "Geeks" );
al.add( "Geeks" );
al.add( 1 , "Geeks" );
System.out.println( "Initial ArrayList " + al);
al.set( 1 , "For" );
System.out.println( "Updated ArrayList " + al);
}
}
输出如下:
Initial ArrayList [Geeks, Geeks, Geeks]
Updated ArrayList [Geeks, For, Geeks]
3.删除元素:为了从列表中删除一个元素, 我们可以使用remove()方法。重载此方法可根据不同的参数执行多项操作。他们是:
- remove(Object):此方法仅用于从列表中删除对象。如果存在多个此类对象, 则将删除对象的第一个匹配项。
- remove(int index):由于列表已建立索引, 因此此方法采用整数值, 该整数值会简单地删除列表中该特定索引处存在的元素。删除元素后, 所有元素都将向左移动以填充空间, 并且对象的索引将更新。
// Java program to remove elements
// in a List
import java.util.*;
public class GFG {
public static void main(String args[])
{
List<String> al = new ArrayList<>();
al.add( "Geeks" );
al.add( "Geeks" );
al.add( 1 , "For" );
System.out.println(
"Initial ArrayList " + al);
al.remove( 1 );
System.out.println(
"After the Index Removal " + al);
al.remove( "Geeks" );
System.out.println(
"After the Object Removal " + al);
}
}
输出如下:
Initial ArrayList [Geeks, For, Geeks]
After the Index Removal [Geeks, Geeks]
After the Object Removal [Geeks]
4.迭代列表:有多种方法可以遍历列表。最著名的方法是使用基本for循环结合get()方法使元素处于特定索引处, 并且先进的循环.
// Java program to iterate the elements
// in an ArrayList
import java.util.*;
public class GFG {
public static void main(String args[])
{
List<String> al
= new ArrayList<>();
al.add( "Geeks" );
al.add( "Geeks" );
al.add( 1 , "For" );
// Using the Get method and the
// for loop
for ( int i = 0 ; i < al.size(); i++) {
System.out.print(al.get(i) + " " );
}
System.out.println();
// Using the for each loop
for (String str : al)
System.out.print(str + " " );
}
}
输出如下:
Geeks For Geeks
Geeks For Geeks
创建列表对象
以来list是一个接口, 无法创建类型列表的对象。我们总是需要一个扩展此列表的类以创建一个对象。而且, 在引入泛型在Java 1.5中, 可以限制可以存储在List中的对象的类型。此类型安全列表可以定义为:
// Obj是要存储在List中的对象的类型List <Obj> list = new ArrayList <Obj>();
实现List接口的类
1.数组列表:在集合框架中实现的ArrayList类为我们提供了Java动态数组。虽然, 它可能比标准数组要慢, 但是在需要大量操作数组的程序中会很有帮助。让我们看看如何使用此类创建列表对象。
// Java program to demonstrate the
// creation of list object using the
// ArrayList class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of ArrayList
int n = 5 ;
// Declaring the List with initial size n
List<Integer> arrli
= new ArrayList<Integer>(n);
// Appending the new elements
// at the end of the list
for ( int i = 1 ; i <= n; i++)
arrli.add(i);
// Printing elements
System.out.println(arrli);
// Remove element at index 3
arrli.remove( 3 );
// Displaying the list after deletion
System.out.println(arrli);
// Printing elements one by one
for ( int i = 0 ; i < arrli.size(); i++)
System.out.print(arrli.get(i) + " " );
}
}
输出如下:
[1, 2, 3, 4, 5]
[1, 2, 3, 5]
1 2 3 5
2.向量:Vector是在收集框架中实现的类, 该类实现可增长的对象数组。 Vector实现了动态数组, 这意味着它可以根据需要增长或缩小。像数组一样, 它包含可以使用整数索引访问的组件。向量基本上属于传统类, 但现在与集合完全兼容。让我们看看如何使用此类创建列表对象。
// Java program to demonstrate the
// creation of list object using the
// Vector class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the vector
int n = 5 ;
// Declaring the List with initial size n
List<Integer> v = new Vector<Integer>(n);
// Appending the new elements
// at the end of the list
for ( int i = 1 ; i <= n; i++)
v.add(i);
// Printing elements
System.out.println(v);
// Remove element at index 3
v.remove( 3 );
// Displaying the list after deletion
System.out.println(v);
// Printing elements one by one
for ( int i = 0 ; i < v.size(); i++)
System.out.print(v.get(i) + " " );
}
}
输出如下:
[1, 2, 3, 4, 5]
[1, 2, 3, 5]
1 2 3 5
3.堆栈:Stack是一个在集合框架中实现的类, 它扩展了向量类模型并实现了堆栈数据结构。该课程基于后进先出的基本原则。除了基本的推入和弹出操作外, 该类还提供了空, 搜索和查看的三个功能。让我们看看如何使用此类创建列表对象。
// Java program to demonstrate the
// creation of list object using the
// Stack class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the stack
int n = 5 ;
// Declaring the List
List<Integer> s = new Stack<Integer>();
// Appending the new elements
// at the end of the list
for ( int i = 1 ; i <= n; i++)
s.add(i);
// Printing elements
System.out.println(s);
// Remove element at index 3
s.remove( 3 );
// Displaying the list after deletion
System.out.println(s);
// Printing elements one by one
for ( int i = 0 ; i < s.size(); i++)
System.out.print(s.get(i) + " " );
}
}
输出如下:
[1, 2, 3, 4, 5]
[1, 2, 3, 5]
1 2 3 5
4.链表:LinkedList是在收集框架中实现的类, 该框架固有地实现了链表数据结构。它是一种线性数据结构, 其中元素没有存储在连续的位置, 并且每个元素都是具有数据部分和地址部分的单独对象。元素使用指针和地址链接。每个元素称为一个节点。由于动态性以及插入和删除的简便性, 它们比阵列更可取。让我们看看如何使用此类创建列表对象。
// Java program to demonstrate the
// creation of list object using the
// LinkedList class
import java.io.*;
import java.util.*;
class GFG {
public static void main(String[] args)
{
// Size of the LinkedList
int n = 5 ;
// Declaring the List with initial size n
List<Integer> ll = new LinkedList<Integer>();
// Appending the new elements
// at the end of the list
for ( int i = 1 ; i <= n; i++)
ll.add(i);
// Printing elements
System.out.println(ll);
// Remove element at index 3
ll.remove( 3 );
// Displaying the list after deletion
System.out.println(ll);
// Printing elements one by one
for ( int i = 0 ; i < ll.size(); i++)
System.out.print(ll.get(i) + " " );
}
}
输出如下:
[1, 2, 3, 4, 5]
[1, 2, 3, 5]
1 2 3 5
现在让我们来看看深入列出界面 为了更好的理解:
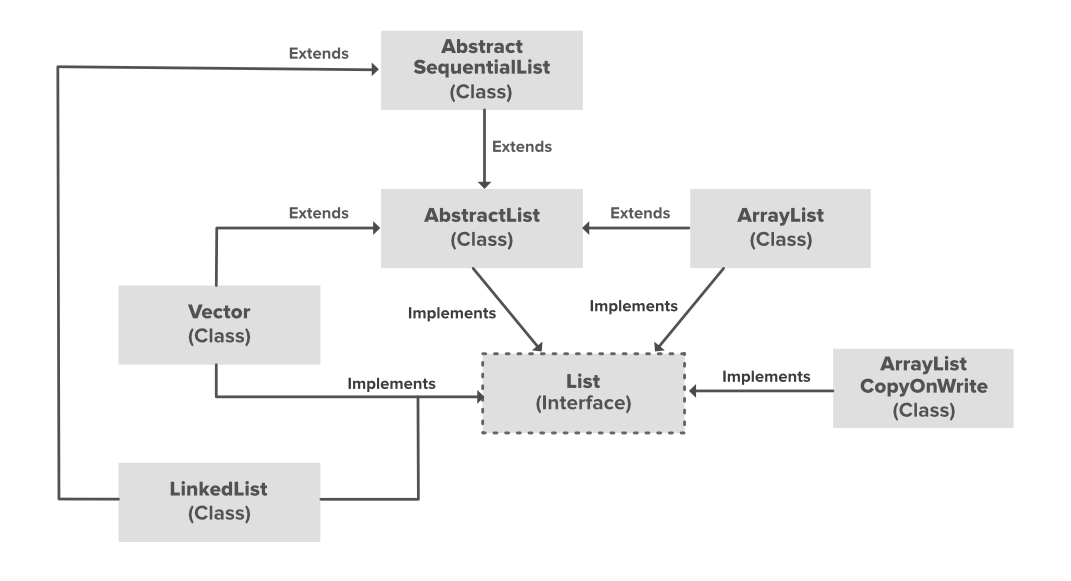
在上图中, 摘要清单, CopyOnWriteArrayList和AbstractSequentialList是实现列表接口的类。在每个提到的类中实现了单独的功能。他们是:
- 摘要清单:此类用于实现不可修改的列表, 对于该列表只需扩展此AbstractList类并仅实现得到()和尺寸()方法。
- CopyOnWriteArrayList:此类实现列表接口。它是的增强版本数组列表其中所有修改(添加, 设置, 删除等)都是通过制作列表的新副本来实现的。
- AbstractSequentialList:此类实现采集界面和AbstractCollection类。此类用于实现不可修改的列表, 对于该列表只需扩展此AbstractList类并仅实现得到()和尺寸()方法。
列表界面的方法
由于不同类型的列表背后的主要概念相同, 因此列表接口包含以下方法:
方法 | 描述 |
---|---|
add(int index, element) | 此方法用于在列表中的特定索引处添加元素。传递单个参数时, 它只是将元素添加到列表的末尾。 |
addAll(int index, Collection collection) | 此方法用于将给定集合中的所有元素添加到列表中。传递单个参数时, 它将给定集合的所有元素添加到列表的末尾。 |
尺寸() | 此方法用于返回列表的大小。 |
明确() | 此方法用于删除列表中的所有元素。但是, 创建的列表的引用仍被存储。 |
remove(int index) | 此方法从指定的索引中删除一个元素。它将后继元素(如果有)向左移动并将其索引减小1。 |
删除(元素) | 此方法用于删除列表中给定元素的第一次出现。 |
get(int索引) | 此方法返回指定索引处的元素。 |
set(int index, element) | 此方法将给定索引处的元素替换为新元素。此函数返回刚刚被新元素替换的元素。 |
indexOf(element) | 此方法返回给定元素的第一次出现;如果列表中不存在该元素, 则返回-1。 |
lastIndexOf(element) | 此方法返回给定元素的最后一次出现;如果列表中不存在该元素, 则返回-1。 |
等于(元素) | 此方法用于比较给定元素与列表元素的相等性。 |
哈希码() | 此方法用于返回给定列表的哈希码值。 |
是空的() | 此方法用于检查列表是否为空。如果列表为空, 则返回true, 否则返回false。 |
包含(元素) | 此方法用于检查列表是否包含给定元素。如果列表包含元素, 则返回true。 |
containsAll(Collection collection) | 此方法用于检查列表是否包含所有元素集合。 |
排序(比较器comp) | 此方法用于根据给定的比较器对列表中的元素进行排序。 |