我们知道装饰工是Python中非常强大且有用的工具, 因为它允许程序员修改函数或类的行为。在本文中, 我们将了解 带参数的装饰器借助多个示例。
Python函数是First Class公民, 这意味着可以像对待对象一样对待函数。
- 可以将功能分配给变量, 即可以对其进行引用。
- 可以将函数作为参数传递给另一个函数。
- 可以从函数返回函数。
具有参数的装饰器与普通装饰器类似。
具有参数的装饰器的语法–
@decorator(params)
def func_name():
''' Function implementation'''
上面的代码相当于
def func_name():
''' Function implementation'''
func_name = (decorator(params))(func_name)
"""
从左到右执行装饰器(参数)被调用返回一个函数对象fun_obj。使用fun_obj进行调用fun_obj(有趣的名字)制造。在内部函数内部, 执行所需的操作并返回实际的函数引用, 该引用将分配给func_name。现在, func_name()可用于调用应用了装饰器的函数。
带参数的装饰器如何实现
def decorators( * args, * * kwargs):
def inner(func):
'''
do operations with func
'''
return func
return inner #this is the fun_obj mentioned in the above content
@decorators (params)
def func():
"""
function implementation
"""
这里参数也可以为空。
首先观察这些–
# Python code to illustrate
# Decorators basic in Python
def decorator_fun(func):
print ( "Inside decorator" )
def inner( * args, * * kwargs):
print ( "Inside inner function" )
print ( "Decorated the function" )
# do operations with func
func()
return inner
@decorator_fun
def func_to():
print ( "Inside actual function" )
func_to()
另一种方式 -
# Python code to illustrate
# Decorators with parameters in Python
def decorator_fun(func):
print ( "Inside decorator" )
def inner( * args, * * kwargs):
print ( "Inside inner function" )
print ( "Decorated the function" )
func()
return inner
def func_to():
print ( "Inside actual function" )
# another way of using decorators
decorator_fun(func_to)()
输出如下:
Inside decorator
Inside inner function
I like lsbin
Inside actual function
让我们来看另一个例子–
范例1:
# Python code to illustrate
# Decorators with parameters in Python
def decorator( * args, * * kwargs):
print ( "Inside decorator" )
def inner(func):
# code functionality here
print ( "Inside inner function" )
print ( "I like" , kwargs[ 'like' ])
func()
# reurning inner function
return inner
@decorator (like = "lsbin" )
def my_func():
print ( "Inside actual function" )
输出如下:
Inside decorator
Inside inner function
I like lsbin
Inside actual function
范例2:
# Python code to illustrate
# Decorators with parameters in Python
def decorator_func(x, y):
def Inner(func):
def wrapper( * args, * * kwargs):
print ( "I like lsbin" )
print ( "Summation of values - {}" . format (x + y) )
func( * args, * * kwargs)
return wrapper
return Inner
# Not using decorator
def my_fun( * args):
for ele in args:
print (ele)
# another way of using dacorators
decorator_func( 12 , 15 )(my_fun)( 'Geeks' , 'for' , 'Geeks' )
输出如下:
I like lsbin
Summation of values - 27
Geeks
for
Geeks
这个例子还告诉我们, 外部函数参数可以由内部函数访问。
1.室内装饰器
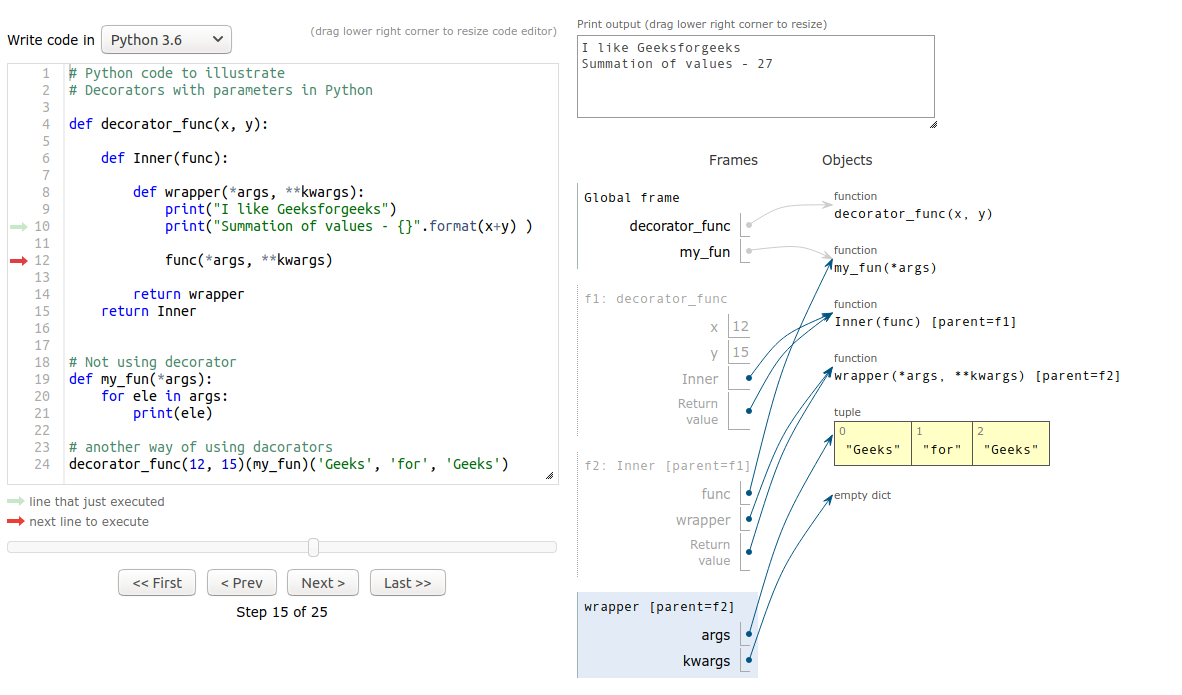
2.函数内部
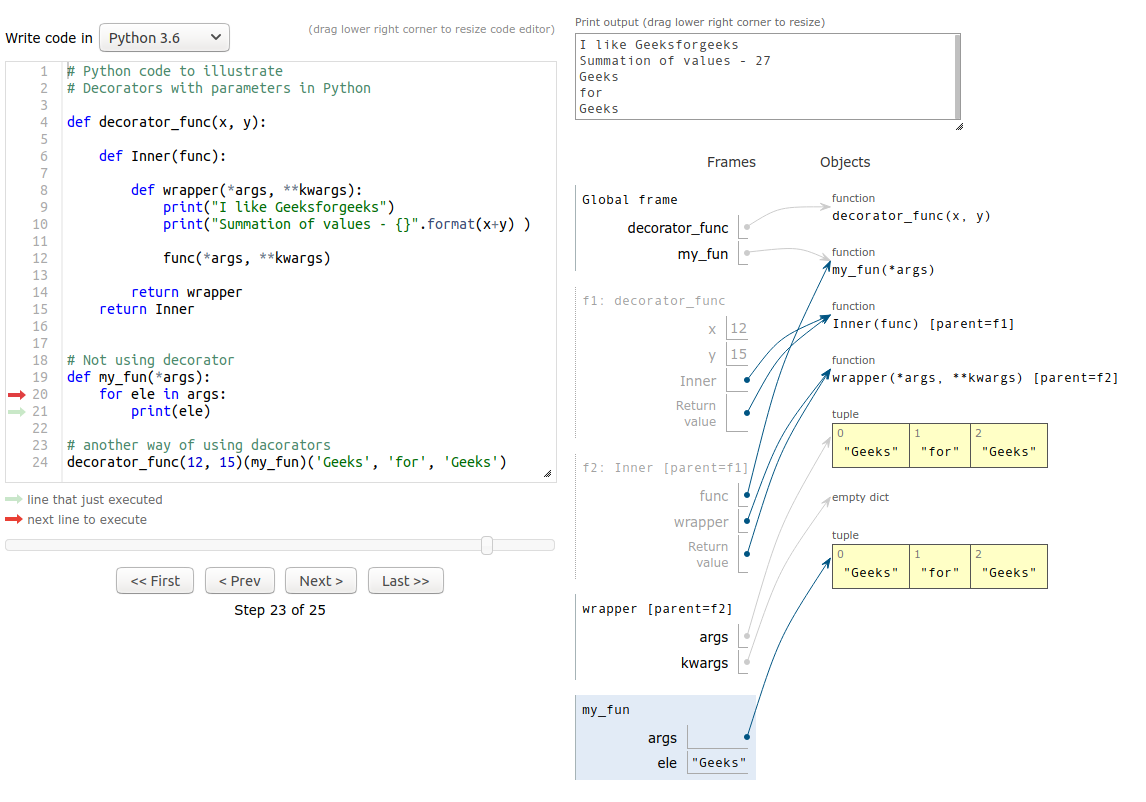
注意 :
图像快照是使用PythonTutor拍摄的。
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。