编程中的决策类似于现实生活中的决策。在编程中, 我们还遇到某些情况, 即当满足某些条件时, 我们希望执行特定的代码块。
编程语言使用控制语句根据某些条件来控制程序的执行流程。这些用于根据程序状态的更改使执行流程前进并分支。
if
嵌套if
if-else-if
这些语句使你可以根据仅在运行时已知的条件来控制程序的执行流程。
if
:if语句是最简单的决策语句。它用于决定是否执行某个语句或语句块, 即, 如果某个条件为真, 则执行语句块, 否则不执行。
语法
:
if(condition)
{
// Statements to execute if
// condition is true
}
这里,
condition
评估后将为真或为假。 if语句接受布尔值–如果该值为true, 则它将执行其下的语句块。
如果我们未在之后提供大括号" {"和"}"
如果(条件)
然后默认情况下, if语句将立即的一个语句视为在其块内。例如,
if(condition)
statement1;
statement2;
// Here if the condition is true, if block
// will consider only statement1 to be inside
// its block.
流程图:
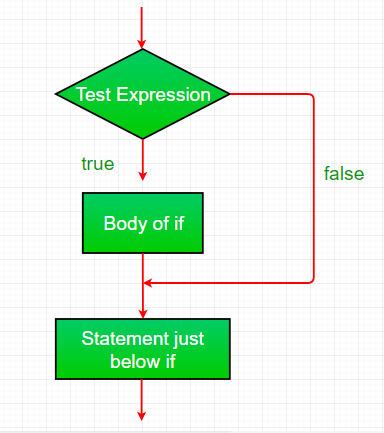
例子:
<script type = "text/javaScript" >
// JavaScript program to illustrate If statement
var i = 10;
if (i > 15)
document.write( "10 is less than 15" );
// This statement will be executed
// as if considers one statement by default
document.write( "I am Not in if" );
< /script>
输出如下:
I am Not in if
if-else
:仅if语句就告诉我们, 如果条件为true, 它将执行语句块, 如果条件为false, 则不会。但是如果条件为假, 我们想做其他事情怎么办。这是else语句。当条件为false时, 可以将else语句与if语句一起使用以执行代码块。
语法
:
if (condition)
{
// Executes this block if
// condition is true
}
else
{
// Executes this block if
// condition is false
}
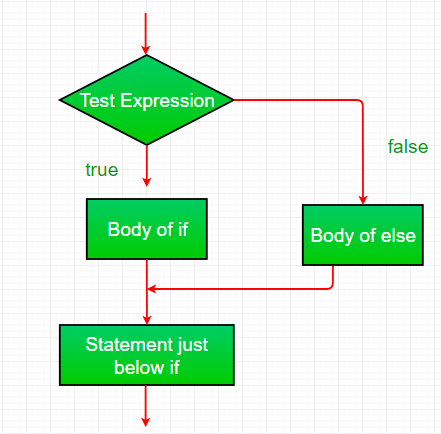
例子:
<script type = "text/javaScript" >
// JavaScript program to illustrate If-else statement
var i = 10;
if (i < 15)
document.write( "10 is less than 15" );
else
document.write( "I am Not in if" );
< /script>
输出如下:
i is smaller than 15
嵌套如果:
嵌套的if是if语句, 它是另一个if or else的目标。嵌套的if语句表示在if语句内的if语句。是的, JavaScript允许我们在if语句中嵌套if语句。也就是说, 我们可以将if语句放在另一个if语句中。
语法如下:
if (condition1)
{
// Executes when condition1 is true
if (condition2)
{
// Executes when condition2 is true
}
}
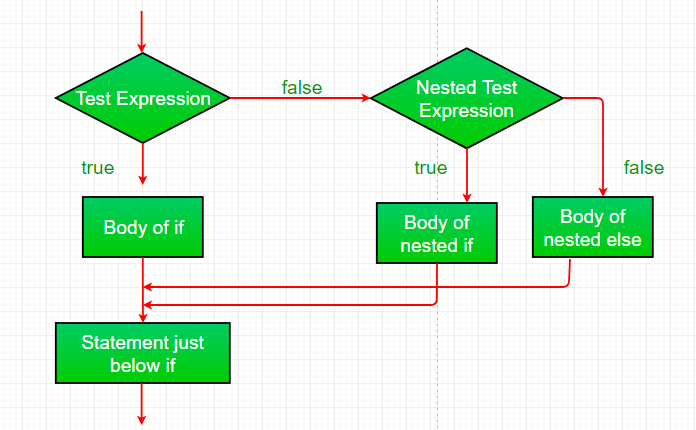
例子:
<script type = "text/javaScript" >
// JavaScript program to illustrate nested-if statement
var i = 10;
if (i == 10) {
// First if statement
if (i < 15)
document.write( "i is smaller than 15" );
// Nested - if statement
// Will only be executed if statement above
// it is true
if (i < 12)
document.write( "i is smaller than 12 too" );
else
document.write( "i is greater than 15" );
}
< /script>
输出如下:
i is smaller than 15
i is smaller than 12 too
if-else-if梯形图:
用户可以在这里选择多个选项。if语句从上至下执行。一旦控制if的条件之一为true, 则将执行与if关联的语句, 并跳过其余的阶梯。如果没有一个条件为真, 则将执行最终的else语句。
if (condition)
statement;
else if (condition)
statement;
.
.
else
statement;
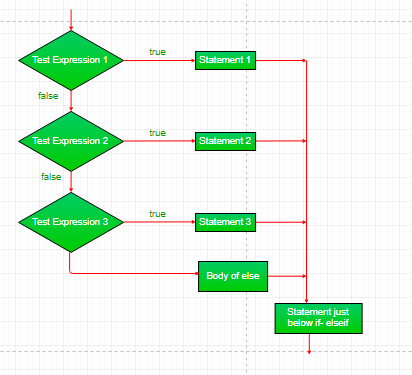
例子:
<script type = "text/javaScript" >
// JavaScript program to illustrate nested-if statement
var i = 20;
if (i == 10)
document.write( "i is 10" );
else if (i == 15)
document.write( "i is 15" );
else if (i == 20)
document.write( "i is 20" );
else
document.write( "i is not present" );
< /script>
输出如下:
i is 20