本文概述
指针存储变量的地址或存储位置。
// General syntax
datatype *var_name;
// An example pointer "ptr" that holds
// address of an integer variable or holds
// address of a memory whose value(s) can
// be accessed as integer values through "ptr"
int *ptr;
使用指针:
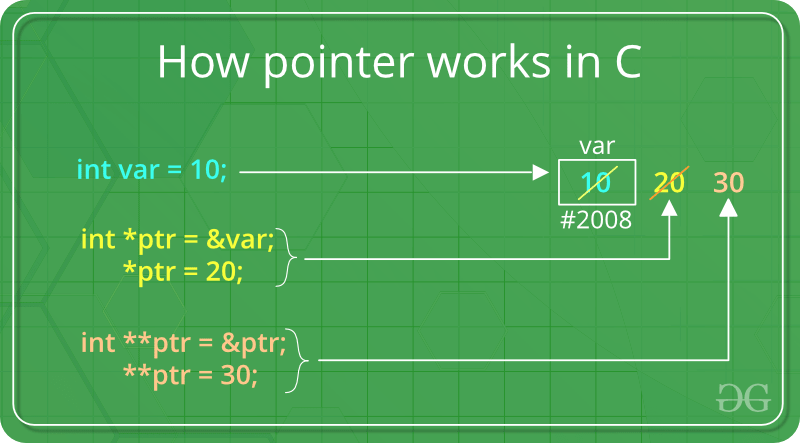
要在C中使用指针, 我们必须了解以下两个运算符。
要访问指向变量的地址, 我们使用一元运算符
&
(与号)返回该变量的地址。例如&x给我们变量x的地址。
// The output of this program can be different
// in different runs. Note that the program
// prints address of a variable and a variable
// can be assigned different address in different
// runs.
#include <stdio.h>
int main()
{
int x;
// Prints address of x
printf ( "%p" , &x);
return 0;
}
还有一个运算符是
一元*
(星号)用于两件事:
声明指针变量:在C / C ++中声明指针变量时, 其名称前必须带有*。
// C program to demonstrate declaration of
// pointer variables.
#include <stdio.h>
int main()
{
int x = 10;
// 1) Since there is * in declaration, ptr
// becomes a pointer varaible (a variable
// that stores address of another variable)
// 2) Since there is int before *, ptr is
// pointer to an integer type variable
int *ptr;
// & operator before x is used to get address
// of x. The address of x is assigned to ptr.
ptr = &x;
return 0;
}
要访问存储在地址中的值, 我们使用一元运算符(*), 该运算符返回位于其操作数指定地址处的变量的值。这也称为
取消引用
.
C ++
// C++ program to demonstrate use of * for pointers in C++
#include <iostream>
using namespace std;
int main()
{
// A normal integer variable
int Var = 10;
// A pointer variable that holds address of var.
int *ptr = &Var;
// This line prints value at address stored in ptr.
// Value stored is value of variable "var"
cout << "Value of Var = " << *ptr << endl;
// The output of this line may be different in different
// runs even on same machine.
cout << "Address of Var = " << ptr << endl;
// We can also use ptr as lvalue (Left hand
// side of assignment)
*ptr = 20; // Value at address is now 20
// This prints 20
cout << "After doing *ptr = 20, *ptr is " << *ptr << endl;
return 0;
}
// This code is contributed by
// shubhamsingh10
C
// C program to demonstrate use of * for pointers in C
#include <stdio.h>
int main()
{
// A normal integer variable
int Var = 10;
// A pointer variable that holds address of var.
int *ptr = &Var;
// This line prints value at address stored in ptr.
// Value stored is value of variable "var"
printf ( "Value of Var = %d\n" , *ptr);
// The output of this line may be different in different
// runs even on same machine.
printf ( "Address of Var = %p\n" , ptr);
// We can also use ptr as lvalue (Left hand
// side of assignment)
*ptr = 20; // Value at address is now 20
// This prints 20
printf ( "After doing *ptr = 20, *ptr is %d\n" , *ptr);
return 0;
}
输出:
Value of Var = 10
Address of Var = 0x7fffa057dd4
After doing *ptr = 20, *ptr is 20
下面是上述程序的图示:
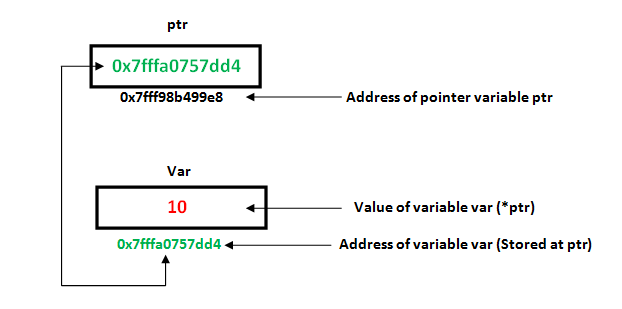
指针表达式和指针算术
可以对指针执行一组有限的算术运算。指针可以是:
- 递增(++)
- 递减(—)
- 可以将整数添加到指针(+或+ =)
- 可以从指针中减去一个整数(–或-=)
指针算术是没有意义的, 除非在数组上执行。
注意:指针包含地址。添加两个地址是没有意义的, 因为不知道它将指向什么。减去两个地址可让你计算这两个地址之间的偏移量。
// C++ program to illustrate Pointer Arithmetic
// in C/C++
#include <bits/stdc++.h>
// Driver program
int main()
{
// Declare an array
int v[3] = {10, 100, 200};
// Declare pointer variable
int *ptr;
// Assign the address of v[0] to ptr
ptr = v;
for ( int i = 0; i < 3; i++)
{
printf ( "Value of *ptr = %d\n" , *ptr);
printf ( "Value of ptr = %p\n\n" , ptr);
// Increment pointer ptr by 1
ptr++;
}
}
Output:Value of *ptr = 10
Value of ptr = 0x7ffcae30c710
Value of *ptr = 100
Value of ptr = 0x7ffcae30c714
Value of *ptr = 200
Value of ptr = 0x7ffcae30c718
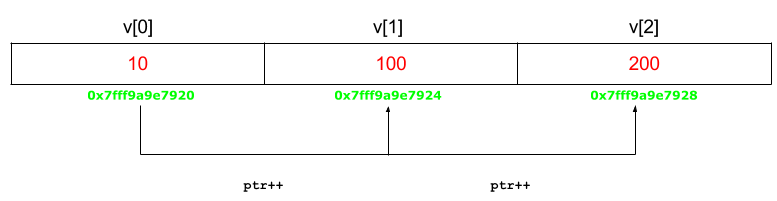
数组名称作为指针
数组名称的行为类似于指针常量。该指针常量的值是第一个元素的地址。
例如, 如果我们有一个名为val的数组, 则
值
和
&val [0]
可以互换使用。
// C++ program to illustrate Array Name as Pointers in C++
#include <bits/stdc++.h>
using namespace std;
void geeks()
{
// Declare an array
int val[3] = { 5, 10, 15};
// Declare pointer variable
int *ptr;
// Assign address of val[0] to ptr.
// We can use ptr=&val[0];(both are same)
ptr = val ;
cout << "Elements of the array are: " ;
cout << ptr[0] << " " << ptr[1] << " " << ptr[2];
return ;
}
// Driver program
int main()
{
geeks();
return 0;
}
Output:
Elements of the array are: 5 10 15

现在, 如果将此ptr作为参数发送给函数, 则可以以类似方式访问数组val。
指针和多维数组
考虑二维数字数组的指针符号。考虑以下声明
int nums[2][3] = { {16, 18, 20}, {25, 26, 27} };
通常, nums [i] [j]等于*(*(nums + i)+ j)
指针符号 | 数组符号 | 值 |
---|---|---|
*(*数字) | nums [0] [0] | 16 |
*(*数字+ 1) | nums [0] [1] | 18 |
*(*数字+ 2) | nums [0] [2] | 20 |
*(*(数字+1)) | nums [1] [0] | 25 |
*(*(数字+1)+1) | nums [1] [1] | 26 |
*(*(数字+1)+ 2) | nums [1] [2] | 27 |