方法通常是程序中的代码或语句块, 可让用户重用最终节省大量内存使用的同一代码, 省时间更重要的是, 它提供了更好的可读性代码。因此, 基本上, 方法是执行某些特定任务并将结果返回给调用方的语句的集合。方法也可以执行某些特定任务而无需返回任何内容。
范例:
// Method Name --> GetCircleArea()
// Return Type ---> double
static double GetCircleArea(double radius)
{
const float pi = 3.14F;
double area = pi * radius * radius;
return area;
}
方法声明
方法声明是指构造方法及其名称的方法。
句法 :
<Access_Modifier> <return_type> <method_name>([<param_list>])
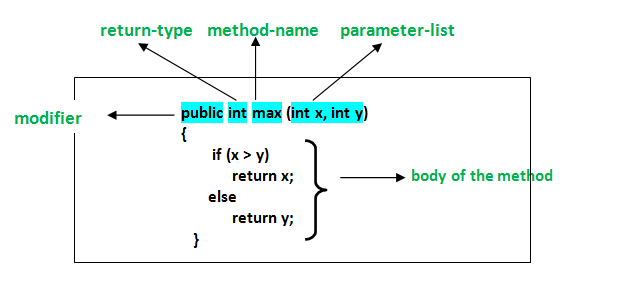
在C#中, 方法声明由以下组件组成:
- 修饰符:它定义了方法的访问类型, 即可以在应用程序中从何处访问方法。在C#中, 有"公共", "受保护", "私有"访问修饰符。
- 方法名称:它描述了用户定义的方法的名称, 用户可以通过该方法调用或引用它。例如。GetName()
- 返回类型:它定义了方法返回的数据类型。它取决于用户, 因为它也可能返回空值, 即什么都不返回
- 方法主体:它指的是该方法在执行过程中要执行的任务的代码行。它被括在大括号之间。
- 参数清单:在括号内定义了输入参数的逗号分隔列表, 并以其数据类型开头。如果没有参数, 则必须使用空括号()。
方法签名:方法签名主要由两个参数(参数数量, 参数类型和参数顺序)定义, 其中之一是方法名称第二个是参数清单.
方法命名:任何编程语言(无论是C ++还是Java或C#)中的方法或函数的名称都很重要, 主要用于调用该方法以执行该方法。例如, findSum, computeMax, setX和getX等。用户应遵循某些预先定义的命名方法规则:
- 方法名称必须是某种名词或动词。
- 命名的方式必须描述该方法的目的。
- 方法名称的首字母可以是小写字母或大写字母, 但是建议使用大写字母。
这些规则不是强制性的, 而是可推荐的。通常, 方法在定义它的类中具有唯一的名称, 但是有时某个方法可能与同一类中的其他方法名称具有相同的名称, 因为C#中允许方法重载。
方法主体:如上所述, 该方法的主体由用户想要执行的代码语句组成。声明方法后, 是否定义其实现取决于用户。不编写任何实现, 会使该方法不执行任何任务。但是, 当用户想要使用方法执行某些任务时, 则必须在方法的主体中编写要执行的语句。以下语法描述了方法主体的基本结构:
句法 :
<return_type> <method_name>(<parameter_list>)
{
// Implementation of the method code goes here.....
}
方法调用
方法调用或方法调用当用户想要执行该方法时完成。需要使用该方法的功能来调用该方法。在以下情况下, 方法将返回调用它的代码:
- 它完成了方法中的所有语句
- 它到达一个返回语句
- 引发异常
范例:在下面的代码中, 一个名为和()叫做。
// C# program to illustrate
// method calling
using System;
namespace ConsoleApplication1 {
class Geeks {
// Here Sum() method asks for two
// parameters from the user and
// calculates the sum of these
// and finally returns the result.
static int Sum( int x, int y)
{
// there are two local variables
// 'a' and 'b' where 'a' is assigned
// the value of parameter 'x' and
// 'b' is assigned the value of
// parameter 'y'
int a = x;
int b = y;
// The local variable calculates
// the sum of 'a' and 'b'
// and returns the result
// which is of 'int' type.
int result = a + b;
return result;
}
// Main Method
static void Main( string [] args)
{
int a = 12;
int b = 23;
// Method Sum() is invoked and
// the returned value is stored
// in the local variable say 'c'
int c = Sum(a, b);
// Display Result
Console.WriteLine( "The Value of the sum is " + c);
}
}
}
输出:
The Value of the sum is 35
方法参数
在某些情况下, 用户希望执行一种方法, 但有时该方法需要一些值输入才能执行和完成其任务。这些输入值称为参数用计算机语言术语来说。现在, 这些参数可以是int, long或float或double或char。但是, 这取决于用户要求。 C#中的方法可以分为不同类别基于返回类型以及输入参数。
没有参数和返回类型的示例程序
// C# program to illustrate method Without
// Parameters & Without Return Type
using System;
namespace ConsoleApplication2 {
class Geeks {
// Here the method 'PrintSentence()'
// neither takes any parameter nor
// returns any value. It simply performs
// the required operations and prints
// the result within it.
static void PrintSentence()
{
Console.WriteLine( "No parameters and return type void" );
}
// Main Method
static void Main( string [] args)
{
// Method Invoking or Method calling
PrintSentence();
}
}
}
输出:
No parameters and return type void
示例程序, 不带参数, 带返回值类型
// C# program to illustrate the method Without
// Parameters & With Return Value Type
using System;
namespace ConsoleApplication3 {
class Geeks {
// This method takes no parameter, // however returns the result obtained
static int sum()
{
int a = 78, b = 70, add;
add = a + b;
return add;
}
// Main Method
static void Main( string [] args)
{
// Here the calling variable
// is 'getresult'
int getresult = sum();
// Prining the value of
// 'getresult' variable
Console.WriteLine(getresult);
}
}
}
输出:
148
有参数且无返回值类型的示例程序
// C# program to illustrate Method With
// Parameters & Without Return Value Type
using System;
namespace ConsoleApplication3 {
class Geeks {
// This method take the side of
// the square as a parameter and
// after obtaining the result, // it simply print it without
// returning anything..
static void perimeter( int p)
{
// Displaying the perimeter
// of the square
Console.WriteLine( "Perimeter of the Square is " + 4 * p);
}
// Main Method
static void Main( string [] args)
{
// side of square
int p = 5;
// Method invoking
perimeter(p);
}
}
}
输出:
Perimeter of the Square is 20
具有参数和返回值类型的示例程序
// C# program to illustrate Method With
// Parameters & With Return Value Type
using System;
namespace ConsoleApplication4 {
class Geeks {
// This method asks a number from
// the user and using that it
// calculates the factorial
// of it and returns the result
static int factorial( int n)
{
int f = 1;
// Method to calculate the
// factorial of a number
for ( int i = 1; i<= n; i++)
{
f = f * i;
}
return f;
}
// Main Method
static void Main( string [] args)
{
int p = 4;
// displaying result by calling the function
Console.WriteLine( "Factorial is : " + factorial(p));
}
}
}
输出:
Factorial is : 24
使用方法的优势:
使用方法有很多优点。下面列出了其中一些:
- 它使程序结构合理。
- 方法提高了代码的可读性。
- 它为用户提供了一种有效的方式来重用现有代码。
- 它优化了执行时间和内存空间。