本文概述
希尔密码是一种基于线性代数的测谎替换密码。每个字母都由数字模26表示。通常使用简单方案A = 0, B = 1, …, Z = 25, 但这并不是密码。为了加密消息, 将n个字母的每个块(被视为n分量矢量)乘以可逆n×n矩阵(模数为26)。要解密消息, 将每个块乘以用于矩阵的逆。加密。
用于加密的矩阵是密码密钥, 应从一组可逆的n×n矩阵(模数为26)中随机选择。
例子:
Input : Plaintext: ACT
Key: GYBNQKURP
Output : Ciphertext: POH
Input : Plaintext: GFG
Key: HILLMAGIC
Output : Ciphertext: SWK
加密
我们必须对消息" ACT"(n = 3)进行加密。密钥为" GYBNQKURP", 可以将其写为nxn矩阵:
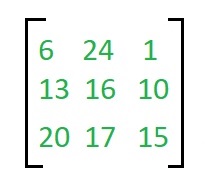
消息" ACT"被写为矢量:
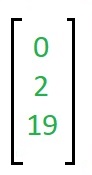
加密的向量为:
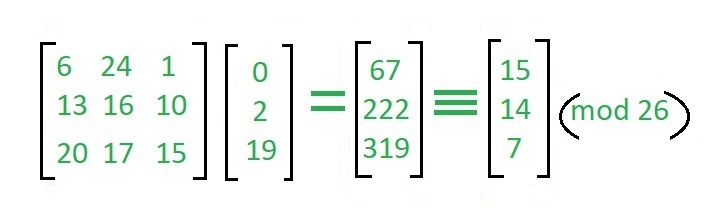
对应于" POH"的密文
为了解密消息, 我们将密文转换回向量, 然后简单地乘以密钥矩阵的逆矩阵(字母为IFKVIVVMI)。上例中使用的矩阵的逆为:
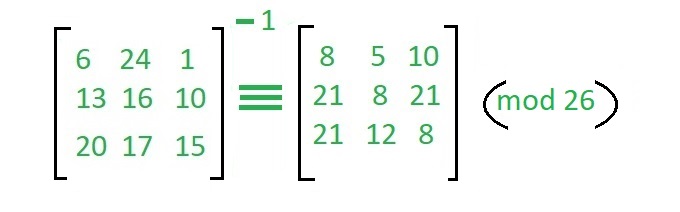
对于先前的密文" POH":
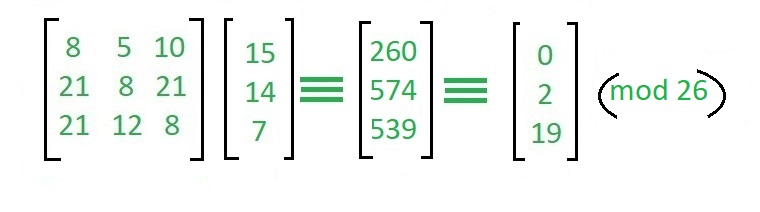
这使我们返回" ACT"。
假定所有字母均大写。
以下是n = 3时上述想法的实现。
C ++
// C++ code to implement Hill Cipher
#include <iostream>
using namespace std;
// Following function generates the
// key matrix for the key string
void getKeyMatrix(string key, int keyMatrix[][3])
{
int k = 0;
for ( int i = 0; i < 3; i++)
{
for ( int j = 0; j < 3; j++)
{
keyMatrix[i][j] = (key[k]) % 65;
k++;
}
}
}
// Following function encrypts the message
void encrypt( int cipherMatrix[][1], int keyMatrix[][3], int messageVector[][1])
{
int x, i, j;
for (i = 0; i < 3; i++)
{
for (j = 0; j < 1; j++)
{
cipherMatrix[i][j] = 0;
for (x = 0; x < 3; x++)
{
cipherMatrix[i][j] +=
keyMatrix[i][x] * messageVector[x][j];
}
cipherMatrix[i][j] = cipherMatrix[i][j] % 26;
}
}
}
// Function to implement Hill Cipher
void HillCipher(string message, string key)
{
// Get key matrix from the key string
int keyMatrix[3][3];
getKeyMatrix(key, keyMatrix);
int messageVector[3][1];
// Generate vector for the message
for ( int i = 0; i < 3; i++)
messageVector[i][0] = (message[i]) % 65;
int cipherMatrix[3][1];
// Following function generates
// the encrypted vector
encrypt(cipherMatrix, keyMatrix, messageVector);
string CipherText;
// Generate the encrypted text from
// the encrypted vector
for ( int i = 0; i < 3; i++)
CipherText += cipherMatrix[i][0] + 65;
// Finally print the ciphertext
cout << " Ciphertext:" << CipherText;
}
// Driver function for above code
int main()
{
// Get the message to be encrypted
string message = "ACT" ;
// Get the key
string key = "GYBNQKURP" ;
HillCipher(message, key);
return 0;
}
Java
// Java code to implement Hill Cipher
class GFG
{
// Following function generates the
// key matrix for the key string
static void getKeyMatrix(String key, int keyMatrix[][])
{
int k = 0 ;
for ( int i = 0 ; i < 3 ; i++)
{
for ( int j = 0 ; j < 3 ; j++)
{
keyMatrix[i][j] = (key.charAt(k)) % 65 ;
k++;
}
}
}
// Following function encrypts the message
static void encrypt( int cipherMatrix[][], int keyMatrix[][], int messageVector[][])
{
int x, i, j;
for (i = 0 ; i < 3 ; i++)
{
for (j = 0 ; j < 1 ; j++)
{
cipherMatrix[i][j] = 0 ;
for (x = 0 ; x < 3 ; x++)
{
cipherMatrix[i][j] +=
keyMatrix[i][x] * messageVector[x][j];
}
cipherMatrix[i][j] = cipherMatrix[i][j] % 26 ;
}
}
}
// Function to implement Hill Cipher
static void HillCipher(String message, String key)
{
// Get key matrix from the key string
int [][]keyMatrix = new int [ 3 ][ 3 ];
getKeyMatrix(key, keyMatrix);
int [][]messageVector = new int [ 3 ][ 1 ];
// Generate vector for the message
for ( int i = 0 ; i < 3 ; i++)
messageVector[i][ 0 ] = (message.charAt(i)) % 65 ;
int [][]cipherMatrix = new int [ 3 ][ 1 ];
// Following function generates
// the encrypted vector
encrypt(cipherMatrix, keyMatrix, messageVector);
String CipherText= "" ;
// Generate the encrypted text from
// the encrypted vector
for ( int i = 0 ; i < 3 ; i++)
CipherText += ( char )(cipherMatrix[i][ 0 ] + 65 );
// Finally print the ciphertext
System.out.print( " Ciphertext:" + CipherText);
}
// Driver code
public static void main(String[] args)
{
// Get the message to be encrypted
String message = "ACT" ;
// Get the key
String key = "GYBNQKURP" ;
HillCipher(message, key);
}
}
// This code has been contributed by 29AjayKumar
Python3
# Python3 code to implement Hill Cipher
keyMatrix = [[ 0 ] * 3 for i in range ( 3 )]
# Generate vector for the message
messageVector = [[ 0 ] for i in range ( 3 )]
# Generate vector for the cipher
cipherMatrix = [[ 0 ] for i in range ( 3 )]
# Following function generates the
# key matrix for the key string
def getKeyMatrix(key):
k = 0
for i in range ( 3 ):
for j in range ( 3 ):
keyMatrix[i][j] = ord (key[k]) % 65
k + = 1
# Following function encrypts the message
def encrypt(messageVector):
for i in range ( 3 ):
for j in range ( 1 ):
cipherMatrix[i][j] = 0
for x in range ( 3 ):
cipherMatrix[i][j] + = (keyMatrix[i][x] *
messageVector[x][j])
cipherMatrix[i][j] = cipherMatrix[i][j] % 26
def HillCipher(message, key):
# Get key matrix from the key string
getKeyMatrix(key)
# Generate vector for the message
for i in range ( 3 ):
messageVector[i][ 0 ] = ord (message[i]) % 65
# Following function generates
# the encrypted vector
encrypt(messageVector)
# Generate the encrypted text
# from the encrypted vector
CipherText = []
for i in range ( 3 ):
CipherText.append( chr (cipherMatrix[i][ 0 ] + 65 ))
# Finally print the ciphertext
print ( "Ciphertext: " , "".join(CipherText))
# Driver Code
def main():
# Get the message to
# be encrypted
message = "ACT"
# Get the key
key = "GYBNQKURP"
HillCipher(message, key)
if __name__ = = "__main__" :
main()
# This code is contributed
# by Pratik Somwanshi
C#
// C# code to implement Hill Cipher
using System;
class GFG
{
// Following function generates the
// key matrix for the key string
static void getKeyMatrix(String key, int [, ]keyMatrix)
{
int k = 0;
for ( int i = 0; i < 3; i++)
{
for ( int j = 0; j < 3; j++)
{
keyMatrix[i, j] = (key[k]) % 65;
k++;
}
}
}
// Following function encrypts the message
static void encrypt( int [, ]cipherMatrix, int [, ]keyMatrix, int [, ]messageVector)
{
int x, i, j;
for (i = 0; i < 3; i++)
{
for (j = 0; j < 1; j++)
{
cipherMatrix[i, j] = 0;
for (x = 0; x < 3; x++)
{
cipherMatrix[i, j] += keyMatrix[i, x] *
messageVector[x, j];
}
cipherMatrix[i, j] = cipherMatrix[i, j] % 26;
}
}
}
// Function to implement Hill Cipher
static void HillCipher(String message, String key)
{
// Get key matrix from the key string
int [, ]keyMatrix = new int [3, 3];
getKeyMatrix(key, keyMatrix);
int [, ]messageVector = new int [3, 1];
// Generate vector for the message
for ( int i = 0; i < 3; i++)
messageVector[i, 0] = (message[i]) % 65;
int [, ]cipherMatrix = new int [3, 1];
// Following function generates
// the encrypted vector
encrypt(cipherMatrix, keyMatrix, messageVector);
String CipherText = "" ;
// Generate the encrypted text from
// the encrypted vector
for ( int i = 0; i < 3; i++)
CipherText += ( char )(cipherMatrix[i, 0] + 65);
// Finally print the ciphertext
Console.Write( "Ciphertext: " + CipherText);
}
// Driver code
public static void Main(String[] args)
{
// Get the message to be encrypted
String message = "ACT" ;
// Get the key
String key = "GYBNQKURP" ;
HillCipher(message, key);
}
}
// This code is contributed by Rajput-Ji
输出如下:
Ciphertext: POH
以类似的方式, 你可以按照上述步骤编写用于解密加密消息的代码。
参考:
https://en.wikipedia.org/wiki/Hill_cipher