拍照只是单击的问题, 所以为什么要玩它应该不仅仅是几行代码。似乎不是python的情况。 python中提供了很多不错的库来处理图像, 例如open-cv, Pillow等。在本文中, 我们将使用公开简历, 一个用于计算机视觉的开源库。它具有C ++, python和java接口。它针对计算机视觉领域的实时应用进行了高度优化(用C / C ++编写)。
缩放图像:-
缩放操作增加/减小图像的尺寸。
import cv2
import numpy as np
FILE_NAME = 'volleyball.jpg'
try :
# Read image from disk.
img = cv2.imread(FILE_NAME)
# Get number of pixel horizontally and vertically.
(height, width) = img.shape[: 2 ]
# Specify the size of image along with interploation methods.
# cv2.INTER_AREA is used for shrinking, whereas cv2.INTER_CUBIC
# is used for zooming.
res = cv2.resize(img, ( int (width / 2 ), int (height / 2 )), interpolation = cv2.INTER_CUBIC)
# Write image back to disk.
cv2.imwrite( 'result.jpg' , res)
except IOError:
print ( 'Error while reading files !!!' )
输出如下:
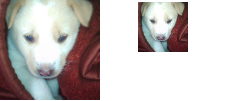
旋转图像:-
图像可以顺时针或以其他方式旋转。我们只需要定义列出旋转点, 旋转度和比例因子的旋转矩阵即可。
import cv2
import numpy as np
FILE_NAME = 'volleyball.jpg'
try :
# Read image from the disk.
img = cv2.imread(FILE_NAME)
# Shape of image in terms of pixels.
(rows, cols) = img.shape[: 2 ]
# getRotationMatrix2D creates a matrix needed for transformation.
# We want matrix for rotation w.r.t center to 45 degree without scaling.
M = cv2.getRotationMatrix2D((cols / 2 , rows / 2 ), 45 , 1 )
res = cv2.warpAffine(img, M, (cols, rows))
# Write image back to disk.
cv2.imwrite( 'result.jpg' , res)
except IOError:
print ( 'Error while reading files !!!' )
输出如下:
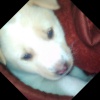
移动图像:-
移动图像意味着在给定的参考框架内移动图像。
import cv2
import numpy as np
FILE_NAME = 'volleyball.jpg'
# Create translation matrix.
# If the shift is (x, y) then matrix would be
# M = [1 0 x]
# [0 1 y]
# Let's shift by (100, 50).
M = np.float32([[ 1 , 0 , 100 ], [ 0 , 1 , 50 ]])
try :
# Read image from disk.
img = cv2.imread(FILE_NAME)
(rows, cols) = img.shape[: 2 ]
# warpAffine does appropriate shifting given the
# translation matrix.
res = cv2.warpAffine(img, M, (cols, rows))
# Write image back to disk.
cv2.imwrite( 'result.jpg' , res)
except IOError:
print ( 'Error while reading files !!!' )
输出如下:
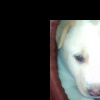
图像中的边缘检测:
图像检测过程涉及检测图像中的尖锐边缘。此边缘检测对于图像识别或
对象定位/检测
。由于具有广泛的适用性, 因此有多种检测边缘的算法。我们将使用一种称为
坎尼边缘检测
.
import cv2
import numpy as np
FILE_NAME = 'volleyball.jpg'
try :
# Read image from disk.
img = cv2.imread(FILE_NAME)
# Canny edge detection.
edges = cv2.Canny(img, 100 , 200 )
# Write image back to disk.
cv2.imwrite( 'result.jpg' , edges)
except IOError:
print ( 'Error while reading files !!!' )
输出如下:
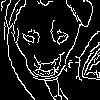
请参考Github更多细节。
注意怪胎!巩固你的基础Python编程基础课程和学习基础知识。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。