继承是OOP(面向对象编程)的重要支柱。这是Java中允许一个类继承另一类的功能(字段和方法)的机制。
重要术语:
- 超级班:继承了其功能的类称为超类(或基类或父类)。
- 子类:继承另一个类的类称为子类(或派生类, 扩展类或子类)。除了超类的字段和方法, 子类还可以添加自己的字段和方法。
- 可重用性:继承支持"可重用性"的概念, 即, 当我们要创建一个新类并且已经有一个包含某些所需代码的类时, 我们可以从现有类中派生新类。通过这样做, 我们可以重用现有类的字段和方法。
如何在Java中使用继承
用于继承的关键字是
.
句法 :
class derived-class extends base-class
{
//methods and fields
}
例子:在下面的继承示例中, Bicycle类是基类, MountainBike类是扩展了Bicycle类的派生类, 而Test类是要运行程序的驱动程序类。
//Java program to illustrate the
// concept of inheritance
// base class
class Bicycle
{
// the Bicycle class has two fields
public int gear;
public int speed;
// the Bicycle class has one constructor
public Bicycle( int gear, int speed)
{
this .gear = gear;
this .speed = speed;
}
// the Bicycle class has three methods
public void applyBrake( int decrement)
{
speed -= decrement;
}
public void speedUp( int increment)
{
speed += increment;
}
// toString() method to print info of Bicycle
public String toString()
{
return ( "No of gears are " +gear
+ "\n"
+ "speed of bicycle is " +speed);
}
}
// derived class
class MountainBike extends Bicycle
{
// the MountainBike subclass adds one more field
public int seatHeight;
// the MountainBike subclass has one constructor
public MountainBike( int gear, int speed, int startHeight)
{
// invoking base-class(Bicycle) constructor
super (gear, speed);
seatHeight = startHeight;
}
// the MountainBike subclass adds one more method
public void setHeight( int newValue)
{
seatHeight = newValue;
}
// overriding toString() method
// of Bicycle to print more info
@Override
public String toString()
{
return ( super .toString()+
"\nseat height is " +seatHeight);
}
}
// driver class
public class Test
{
public static void main(String args[])
{
MountainBike mb = new MountainBike( 3 , 100 , 25 );
System.out.println(mb.toString());
}
}
输出如下:
No of gears are 3
speed of bicycle is 100
seat height is 25
在上面的程序中, 创建MountainBike类的对象时, 超类的所有方法和字段的副本将在该对象中获取内存。这就是为什么通过使用子类的对象, 我们还可以访问超类的成员。
请注意, 在继承期间仅创建子类的对象, 而不创建超类的对象。有关更多信息, 请参阅
继承类的Java对象创建
.
程序的说明图:
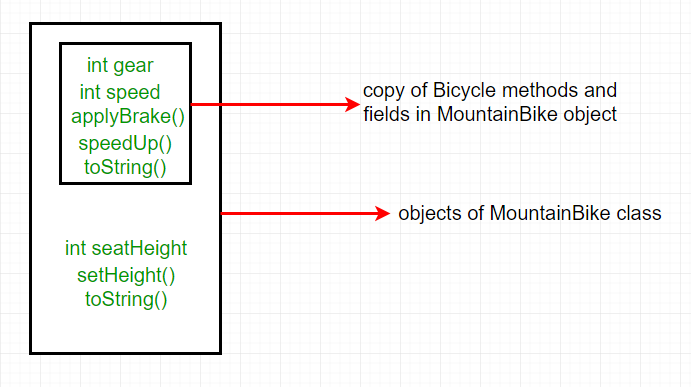
在实践中, 继承和多态性在Java中一起使用可实现快速的性能和代码的可读性。
Java中的继承类型
以下是Java支持的不同类型的继承。
单一继承:
在单一继承中, 子类继承一个超类的功能。在下图中, 类A用作派生类B的基类。
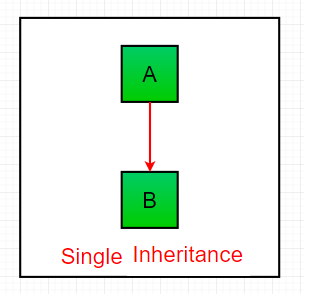
//Java program to illustrate the
// concept of single inheritance
import java.util.*;
import java.lang.*;
import java.io.*;
class one
{
public void print_geek()
{
System.out.println( "Geeks" );
}
}
class two extends one
{
public void print_for()
{
System.out.println( "for" );
}
}
// Driver class
public class Main
{
public static void main(String[] args)
{
two g = new two();
g.print_geek();
g.print_for();
g.print_geek();
}
}
输出如下:
Geeks
for
Geeks
多级继承:
在"多级继承"中, 派生类将继承基类, 并且派生类还充当其他类的基类。在下图中, 类A充当派生类B的基类, 后者又充当派生类C的基类。在Java中, 类无法直接访问
祖父母的成员
.
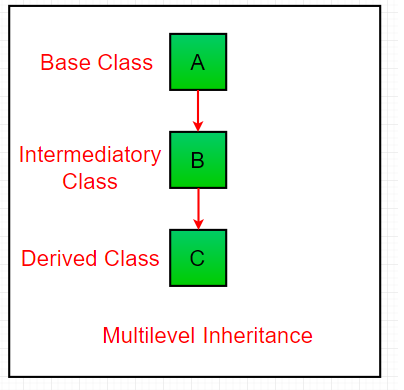
// Java program to illustrate the
// concept of Multilevel inheritance
import java.util.*;
import java.lang.*;
import java.io.*;
class one
{
public void print_geek()
{
System.out.println( "Geeks" );
}
}
class two extends one
{
public void print_for()
{
System.out.println( "for" );
}
}
class three extends two
{
public void print_geek()
{
System.out.println( "Geeks" );
}
}
// Drived class
public class Main
{
public static void main(String[] args)
{
three g = new three();
g.print_geek();
g.print_for();
g.print_geek();
}
}
输出如下:
Geeks
for
Geeks
层次继承:在层次继承中, 一个类充当多个子类的超类(基类)。在下图中, 类A充当派生类B, C和D的基类。
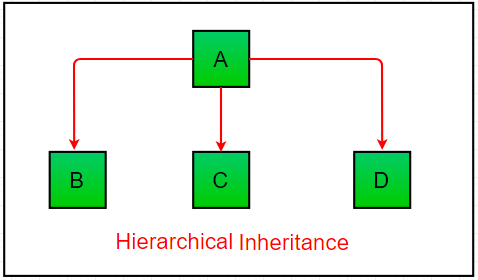
// Java program to illustrate the
// concept of Hierarchical inheritance
import java.util.*;
import java.lang.*;
import java.io.*;
class one
{
public void print_geek()
{
System.out.println( "Geeks" );
}
}
class two extends one
{
public void print_for()
{
System.out.println( "for" );
}
}
class three extends one
{
/*............*/
}
// Drived class
public class Main
{
public static void main(String[] args)
{
three g = new three();
g.print_geek();
two t = new two();
t.print_for();
g.print_geek();
}
}
输出如下:
Geeks
for
Geeks
多重继承
(通过接口):
在多重继承中, 一个类可以具有多个父类, 并且可以从所有父类继承功能。请注意, Java确实
不
支持
多重继承
与类。在Java中, 我们只能通过以下方式实现多重继承
介面
。在下图中, 类C从接口A和B派生。
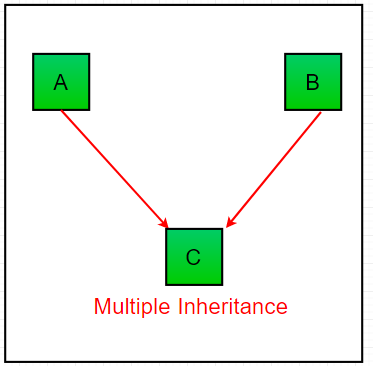
// Java program to illustrate the
// concept of Multiple inheritance
import java.util.*;
import java.lang.*;
import java.io.*;
interface one
{
public void print_geek();
}
interface two
{
public void print_for();
}
interface three extends one, two
{
public void print_geek();
}
class child implements three
{
@Override
public void print_geek() {
System.out.println( "Geeks" );
}
public void print_for()
{
System.out.println( "for" );
}
}
// Drived class
public class Main
{
public static void main(String[] args)
{
child c = new child();
c.print_geek();
c.print_for();
c.print_geek();
}
}
输出如下:
Geeks
for
Geeks
混合继承(通过接口):
它是上述继承类型中的两种或多种的混合。由于Java不支持类的多重继承, 因此混合继承也无法用于类。在Java中, 我们只能通过以下方式实现混合继承
介面
.
有关Java继承的重要事实
- 默认超类: 除Object类, 它没有超类, 每个类只有一个并且只有一个直接超类(单继承)。在没有任何其他显式超类的情况下, 每个类都隐式地是的子类。Object类。
- 超类只能是以下一种:一个超类可以具有任意数量的子类。但是子类只能具有一超类。这是因为Java不支持多重继承与类。尽管具有接口, 但Java支持多重继承。
- 继承构造函数:子类从其超类继承所有成员(字段, 方法和嵌套类)。构造函数不是成员, 因此它们不会被子类继承, 但是可以从子类调用超类的构造函数。
- 私有成员继承:子类不继承其父类的私有成员。但是, 如果超类具有用于访问其私有字段的公共或受保护的方法(如getter和setter), 则这些也可以由子类使用。
在子类中可以做什么?
在子类中, 我们可以按原样继承成员, 替换它们, 隐藏它们或用新成员补充它们:
- 继承的字段可以像其他任何字段一样直接使用。
- 我们可以在子类中声明不在超类中的新字段。
- 继承的方法可以直接使用。
- 我们可以写一个新的实例子类中的方法具有与超类中的签名相同的签名, 因此压倒一切它(如上面的示例所示, toString()方法被覆盖)。
- 我们可以写一个新的static子类中的方法具有与超类中的签名相同的签名, 因此隐藏它。
- 我们可以在子类中声明不在超类中的新方法。
- 我们可以编写一个隐式地或通过使用关键字调用超类的构造函数的子类构造函数。超.